Temperature Conversion Application :
1. Convert from Fahrenheit to Celsius
2. Convert from Celsius to Fahrenheit
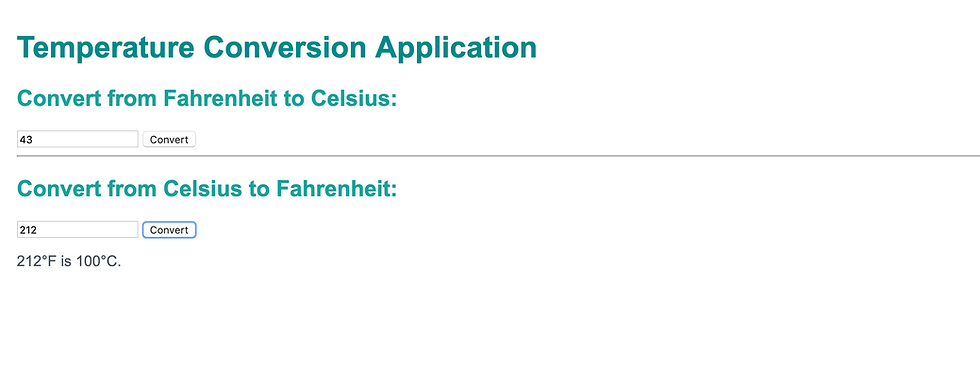
Solution:
Step 1: create index.html file and copy the following code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Temperature Conversion</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>Temperature Conversion Application</h1>
<h2>Convert from Fahrenheit to Celsius: </h2>
<input type="number" id="fahrenheit"></input> <button id="fToC" onclick="fToC(fahrenheit.value)">Convert</button>
<hr />
<h2>Convert from Celsius to Fahrenheit: </h2>
<input type="number" id="celsius"></input> <button id="cToF" onclick="fToC(celsius.value)">Convert</button>
<p id="conversion"></p>
<script src="main.js"></script>
</body>
</html>
Step 2: create main.js in the same location where index.html, copy and paste the below code
function cToF(celsius)
{
console.log(celsius);
var cTemp = parseInt(celsius);
var cToFahr = cTemp * 9 / 5 + 32;
//Note that the Math.round() method is used, so that the result will be returned as an integer.
var message = cTemp+'\xB0C is ' + Math.round(cToFahr) + ' \xB0F.';
document.getElementById("conversion").innerHTML = message;
//console.log(message);
}
function fToC(fahrenheit)
{
console.log(fahrenheit);
var fTemp = parseInt(fahrenheit);
var fToCel = (fTemp - 32) * 5 / 9;
//Note that the Math.round() method is used, so that the result will be returned as an integer.
var message = fTemp+'\xB0F is ' + Math.round(fToCel) + '\xB0C.';
document.getElementById("conversion").innerHTML = message;
//console.log(message);
}
Step 3: finally create style.css in the same location and copy and paste the code below
body {
padding: 20px;
color: #ececec;
}
h1, h2, p {
font-family: Arial, sans-serif;
}
h1 {
color: #008B8B;
}
h2 {
color: #1BA39C;
}
p, label {
color: #4F5A65;
}
All done !!! just run the index.html in browser or open with chrome or Mozila or safari whatever you prefer