Overview and Learning Objectives:
Through this assignment you will build a utility application that allows you to search through your file system for files containing a specific substring within their relative path name.
This assignment will give you practice working with iterators that can be used to step through the contents of a linear array, or through files organized into a directory tree. Independent of how this data is stored, the iterator provides a common interface for stepping through these different kinds of collections of data.
Requirements:
The only types outside of java.lang that can be used for this assignment are:
• java.util.Iterator
• java.io.File
• java.io.FileNotFoundException
• java.util.NoSuchElementException
• java.util.Arrays (only to call Arrays.sort)
The appendix includes links to some of the methods within these classes that are likely to be helpful while implementing this assignment.
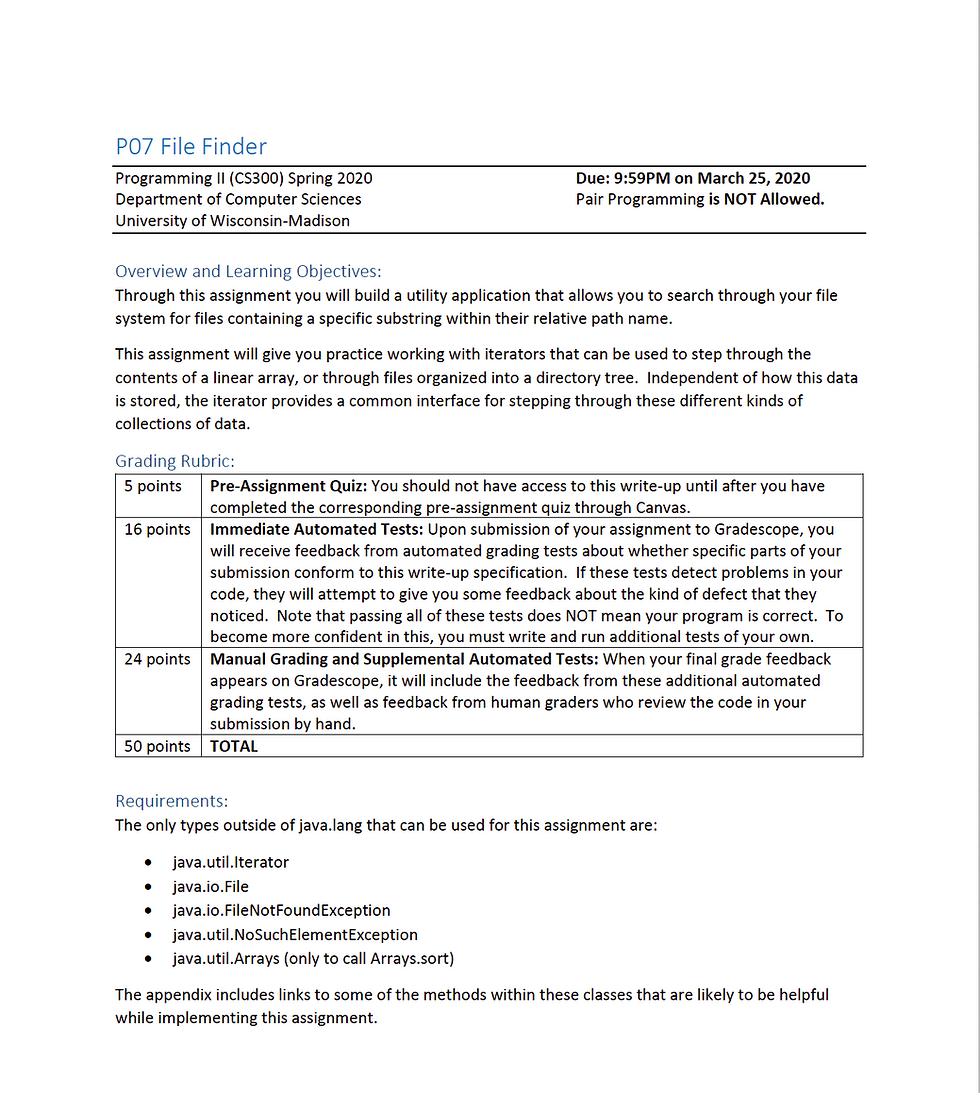
Project Files and Setup
Create a new Java Project in eclipse with a suitable name, like P07 File Finder. Since this project involves stepping through folders and files within a filesystem, we are providing the following p07filesystem.zip to practice with and to demonstrate the expected behavior for this assignment. Download and extract the contents of this zip file into your project folder. The resulting hierarchy of folders should look as follows:
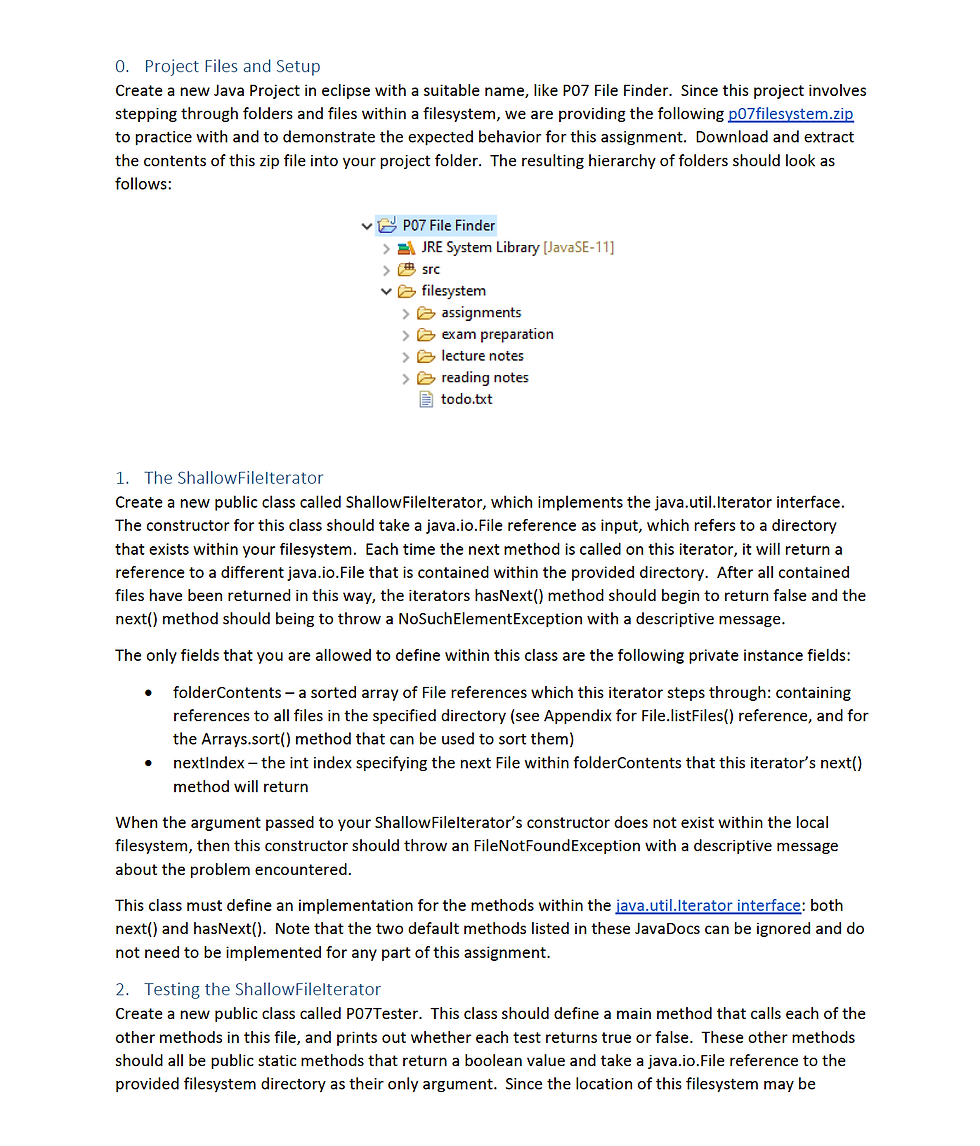
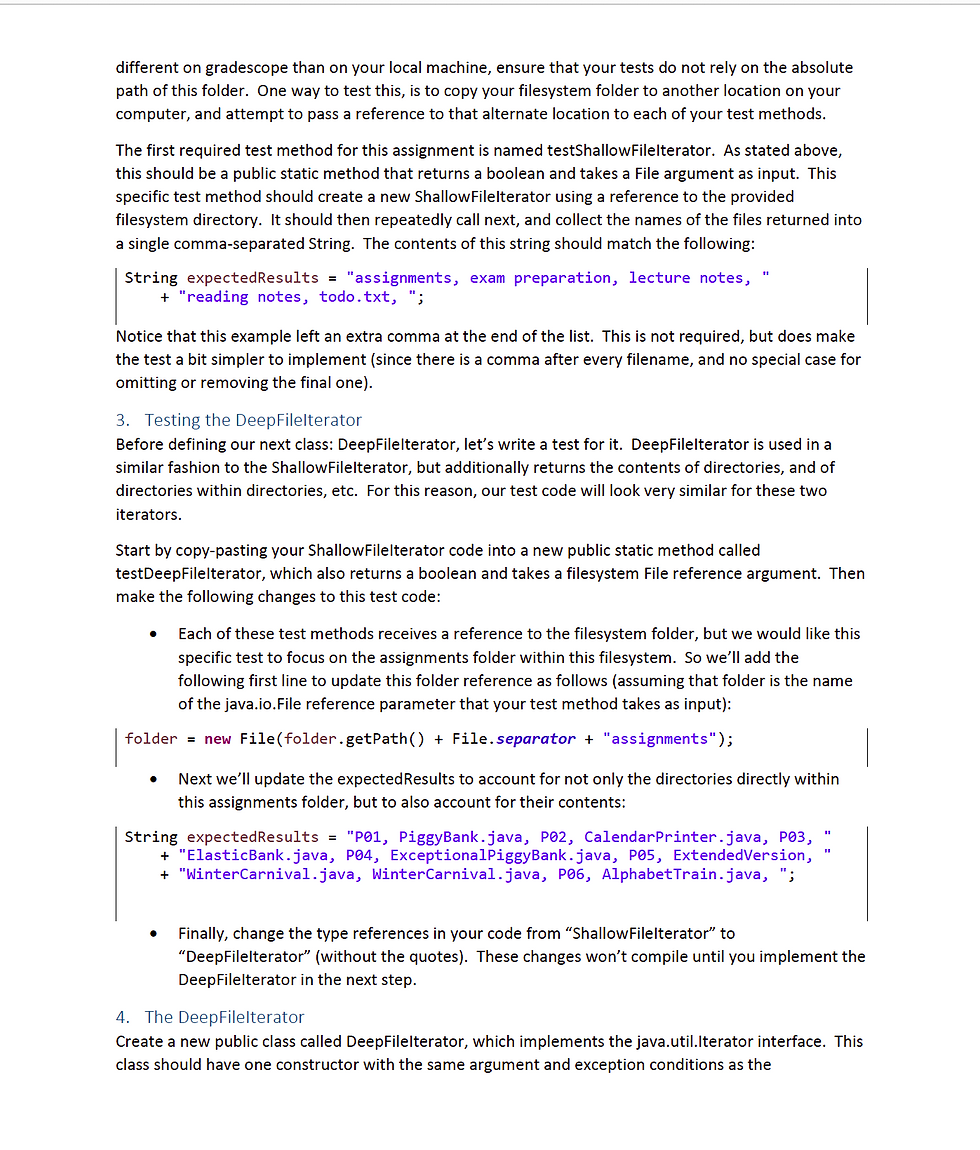
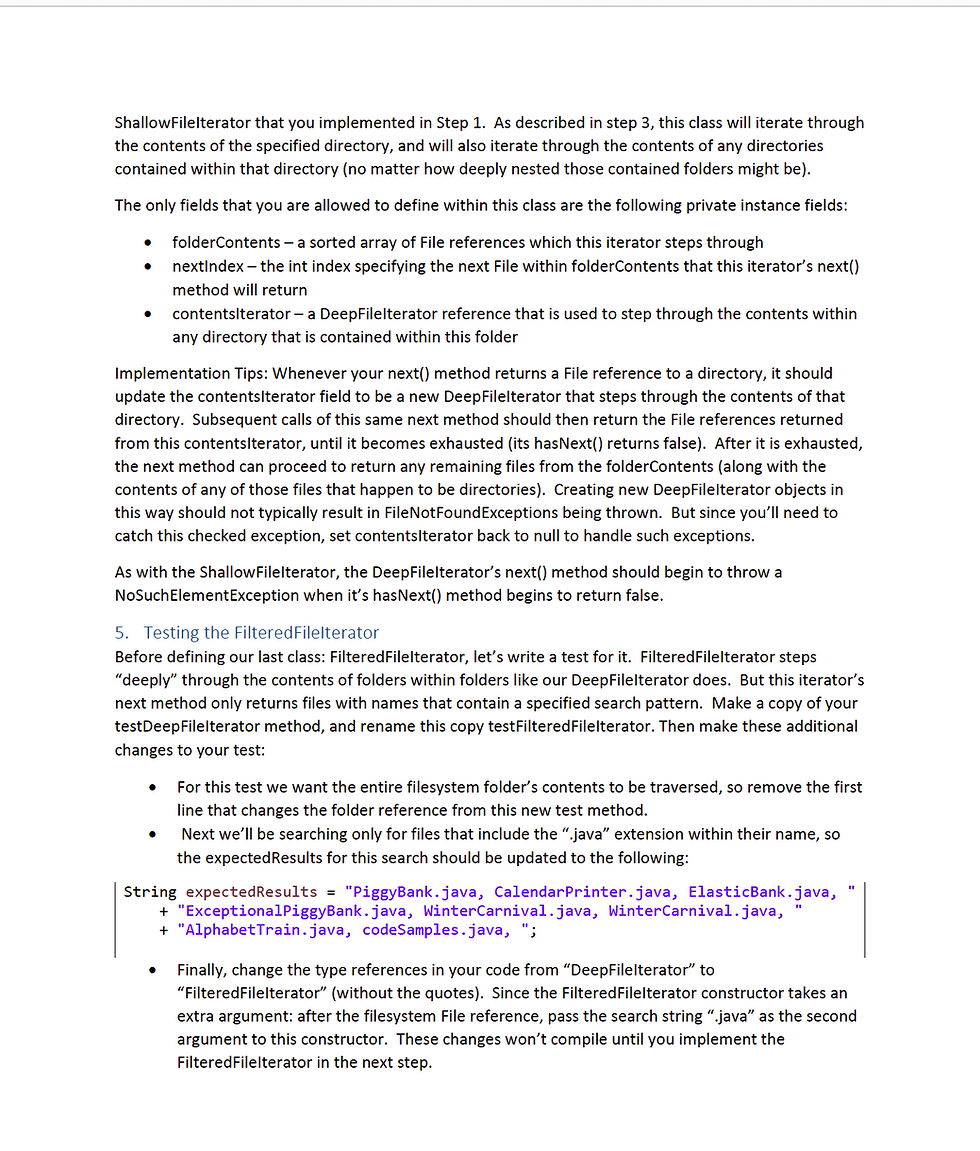
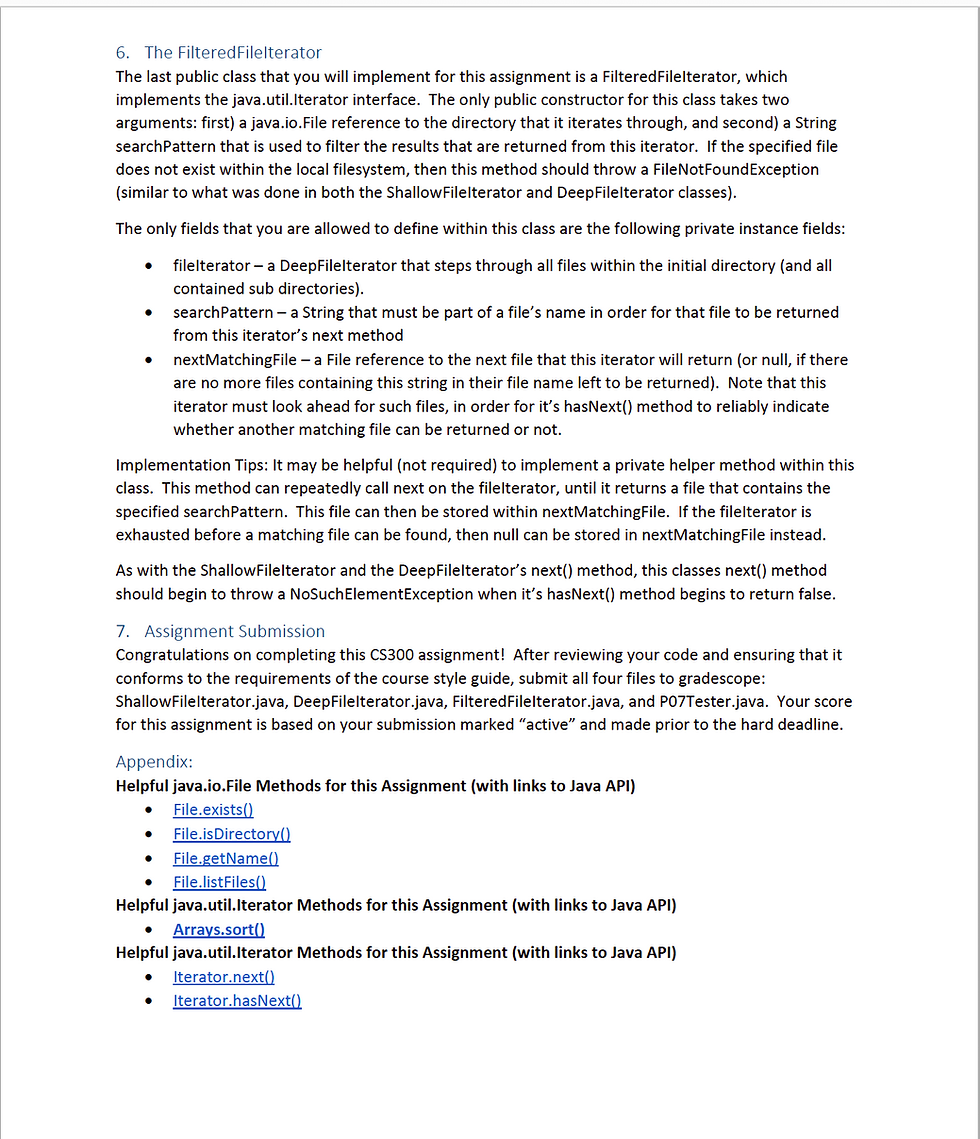
Complete Solutions:
DeepFileIterator.java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Arrays;
import java.util.Iterator;
import java.util.NoSuchElementException;
public class DeepFileIterator implements Iterator<File> {
private File[] folderContents;
private int nextIndex;
private DeepFileIterator contentsIterator;
public DeepFileIterator(File directory) throws FileNotFoundException {
if (!directory.isDirectory()) {
throw new FileNotFoundException();
}
this.folderContents = directory.listFiles();
Arrays.sort(folderContents);
this.nextIndex = -1;
this.contentsIterator = null;
}
@Override
public boolean hasNext() {
return nextIndex != folderContents.length - 1 || (contentsIterator != null && contentsIterator.hasNext());
}
@Override
public File next() {
if (hasNext()) {
if (contentsIterator != null && contentsIterator.hasNext()) {
return contentsIterator.next();
}
contentsIterator = null;
try {
nextIndex++;
contentsIterator = new DeepFileIterator(folderContents[nextIndex]);
} catch (Exception e) {
}
return folderContents[nextIndex];
}
throw new NoSuchElementException("No Next Files");
}
}
FilteredFileIterator.java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* FilteredFileIterator
*/
public class FilteredFileIterator implements Iterator<File> {
private DeepFileIterator fileIterator;
private String searchPattern;
private File nextMatchingFile;
public FilteredFileIterator(File directory, String search) throws FileNotFoundException {
this.fileIterator = new DeepFileIterator(directory);
this.searchPattern = search;
this.nextMatchingFile = searchNext();
}
private File searchNext() {
if (!fileIterator.hasNext()) {
return null;
}
File f = fileIterator.next();
while (!f.getName().contains(searchPattern)) {
try {
f = fileIterator.next();
} catch (Exception e) {
return null;
}
}
return f;
}
@Override
public boolean hasNext() {
return nextMatchingFile != null;
}
@Override
public File next() {
if (hasNext()) {
File t = nextMatchingFile;
nextMatchingFile = searchNext();
return t;
}
throw new NoSuchElementException("No Next Files");
}
}
ShallowFileIterator.java
import java.util.Arrays;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.io.File;
import java.io.FileNotFoundException;
public class ShallowFileIterator implements Iterator<File> {
private File[] folderContents;
private int nextIndex;
public ShallowFileIterator(File directory) throws FileNotFoundException {
if (!directory.isDirectory()) {
throw new FileNotFoundException();
}
this.folderContents = directory.listFiles();
Arrays.sort(folderContents);
this.nextIndex = -1;
}
@Override
public boolean hasNext() {
return nextIndex != folderContents.length - 1;
}
@Override
public File next() {
if (hasNext()) {
nextIndex++;
return folderContents[nextIndex];
}
throw new NoSuchElementException("No Next Files");
}
}
P07Tester.java
import java.io.File;
import java.io.FileNotFoundException;
public class P07Tester {
public static boolean testShallowFileIterator(File directory) {
try {
ShallowFileIterator fileiter = new ShallowFileIterator(directory);
String expectedResults = "";
while (fileiter.hasNext()) {
expectedResults += fileiter.next().getName() + ", ";
}
System.out.println(expectedResults);
return true;
} catch (Exception e) {
return false;
}
}
public static boolean testDeepFileIterator(File folder) {
folder = new File(folder.getPath() + File.separator + "assignments");
try {
DeepFileIterator fileiter = new DeepFileIterator(folder);
String expectedResults = "";
while (fileiter.hasNext()) {
expectedResults += fileiter.next().getName() + ", ";
}
System.out.println(expectedResults);
return true;
} catch (Exception e) {
return false;
}
}
public static boolean testFilteredFileIterator(File directory, String search) {
try {
FilteredFileIterator fileiter = new FilteredFileIterator(directory, search);
String expectedResults = "";
while (fileiter.hasNext()) {
expectedResults += fileiter.next().getName() + ", ";
}
System.out.println(expectedResults);
return true;
} catch (Exception e) {
return false;
}
}
}
Keeping us on top of that foundation, we are creating and providing the best solutions and almost every type of coding solutions to help students.
Contact us for this java assignment Solutions by Codersarts Specialist who can help you mentor and guide for such java assignment, homework, project and coursework
If you have project or assignment files, You can send at contact@codersarts.com directly