Specifications:
1 SceneNode
This class represents a specific scene. A scene has a title(String), a sceneDescription(String), a sceneID(int), and up to three possible child sceneNode references(called left, middle, and right). In addition this class should have the following methods
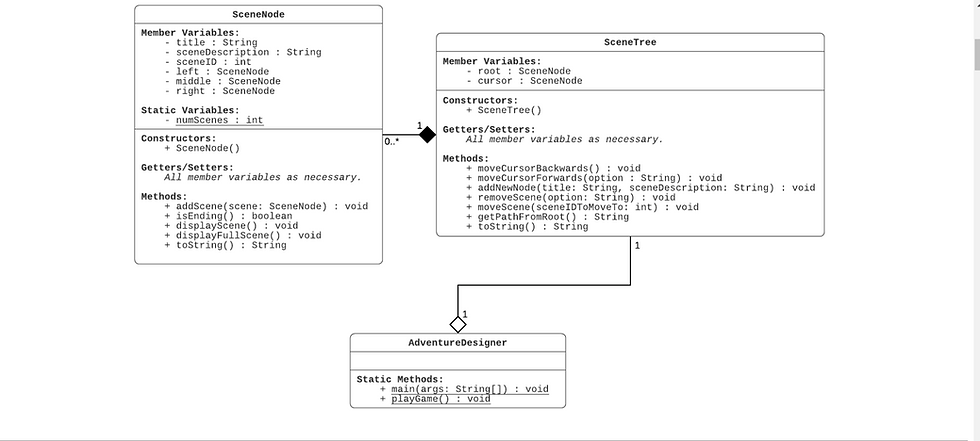
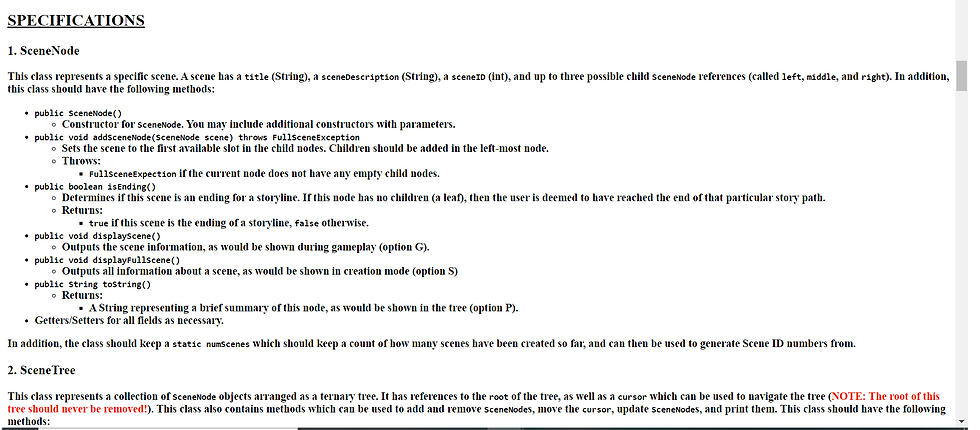
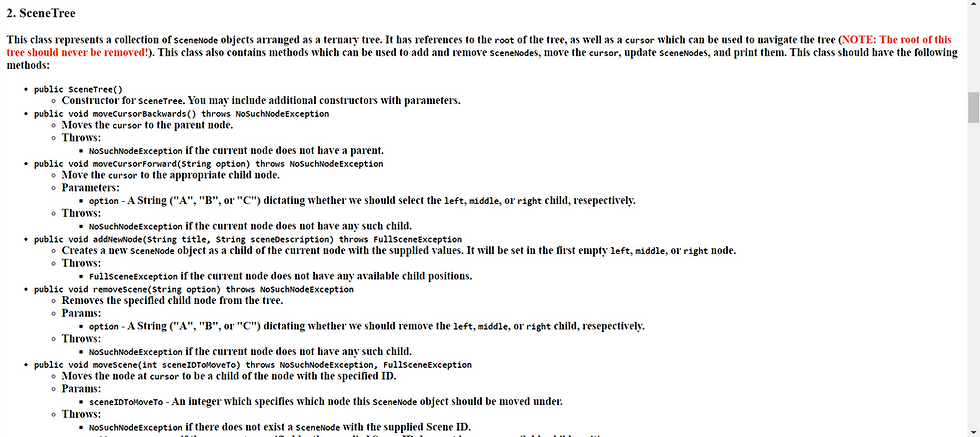
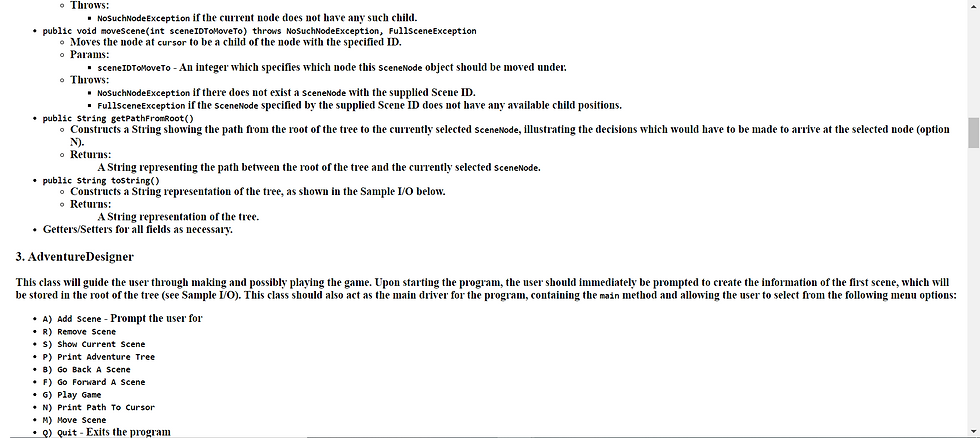
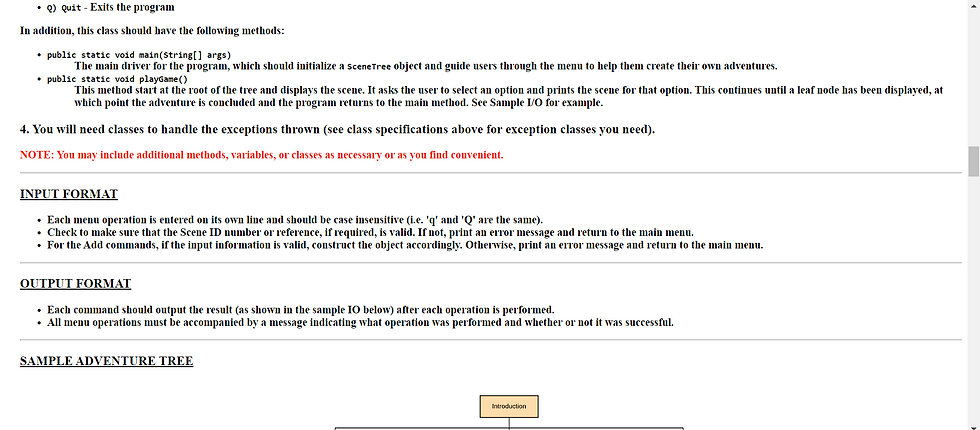
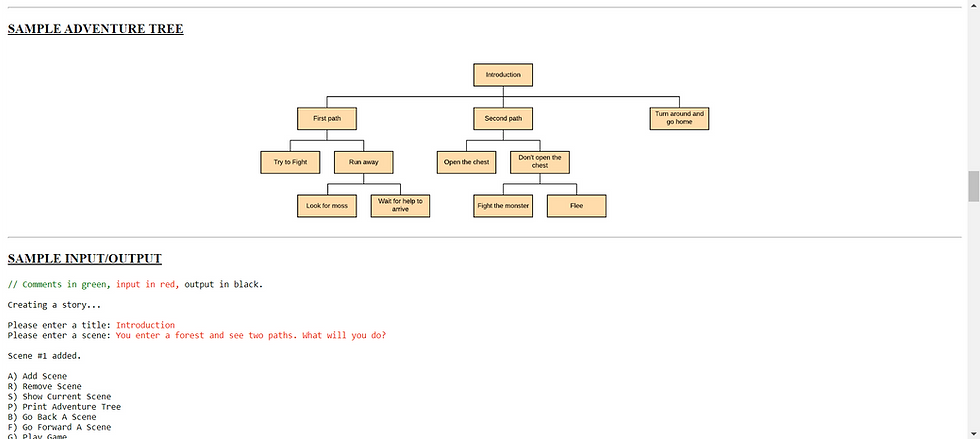
Complete Solutions:
SceneNode.java
public class SceneNode {
private String title, sceneDescription;
private int sceneID;
private SceneNode parent = null, left, middle, right;
private static int numScenes;
public SceneNode() {
}
public SceneNode(String title, String sceneDescription, int sceneID) {
this.title = title;
this.sceneDescription = sceneDescription;
this.sceneID = sceneID;
}
public void addScene(SceneNode scene) throws FullSceneException {
if (left == null) {
left = scene;
left.setParent(this);
} else if (middle == null) {
middle = scene;
middle.setParent(this);
} else if (right == null) {
right = scene;
right.setParent(this);
} else {
throw new FullSceneException("You cannot add another scene!");
}
}
public boolean isEnding() {
return left == null;
}
public void displayScene() { // as shown in G (Gameplay)
if (left != null) {
System.out.println(title);
System.out.println(sceneDescription + "\n");
String s = "";
s += "A) " + left.getTitle() + "\n";
if (middle != null) {
s += "B) " + middle.getTitle() + "\n";
if (right != null) {
s += "C) " + right.getTitle() + "\n";
}
}
System.out.println(s);
}
}
public void displayFullScene() { // as shown in S (creation mode)
System.out.println("Scene ID #" + sceneID);
System.out.println("Title: " + title);
System.out.println("Scene: " + sceneDescription);
String s = "";
if (left != null) {
s += "'" + left.getTitle() + "' (#" + left.getSceneID() + ")";
if (middle != null) {
s += ", '" + middle.getTitle() + "' (#" + middle.getSceneID() + ")";
if (right != null) {
s += ", '" + right.getTitle() + "' (#" + right.getSceneID() + ")";
}
}
}
if (s.equals(""))
s = "None";
System.out.println("Leads to: " + s);
}
public String toString() { // as shown in P (Tree Print)
return title + " (#" + getSceneID() + ")";
}
// Getters and Setters
public SceneNode getParent() {
return parent;
}
public SceneNode getLeft() {
return left;
}
public SceneNode getMiddle() {
return middle;
}
public SceneNode getRight() {
return right;
}
public String getSceneDescription() {
return sceneDescription;
}
public String getTitle() {
return title;
}
public int getSceneID() {
return sceneID;
}
public void setParent(SceneNode parent) {
this.parent = parent;
}
public void setLeft(SceneNode left) {
this.left = left;
}
public void setMiddle(SceneNode middle) {
this.middle = middle;
}
public static void setNumScenes(int numScenes) {
SceneNode.numScenes = numScenes;
}
public void setRight(SceneNode right) {
this.right = right;
}
public void setSceneDescription(String sceneDescription) {
this.sceneDescription = sceneDescription;
}
public void setSceneID(int sceneID) {
this.sceneID = sceneID;
}
public void setTitle(String title) {
this.title = title;
}
}
SceneTree.java
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.Queue;
public class SceneTree {
private SceneNode root, cursor;
private int newNodeID;
public SceneTree() {
}
public SceneTree(String title, String scene) {
root = new SceneNode(title, scene, 1);
cursor = root;
newNodeID = 1;
}
public void moveCursorForwards(String option) throws NoSuchNodeException { // A, B or C
switch (option) {
case "a":
if (cursor.getLeft() != null) {
cursor = cursor.getLeft();
} else
throw new NoSuchNodeException("No child scenes.");
break;
case "b":
if (cursor.getMiddle() != null) {
cursor = cursor.getMiddle();
} else
throw new NoSuchNodeException("That option does not exist.");
break;
case "c":
if (cursor.getRight() != null) {
cursor = cursor.getRight();
} else
throw new NoSuchNodeException("That option does not exist.");
default:
throw new NoSuchNodeException("Invalid input");
}
System.out.println();
System.out.println("Succesfully moved to " + cursor.getTitle() + ".");
}
public void moveCursorBackwords() throws NoSuchNodeException {
if (cursor.getParent() == null) {
throw new NoSuchNodeException("No scene backwards");
}
cursor = cursor.getParent();
System.out.println("Succesfully moved back to " + cursor.getTitle() + ".");
}
public void addNewNode(String title, String sceneDescription) throws FullSceneException {
try {
cursor.addScene(new SceneNode(title, sceneDescription, newNodeID + 1));
newNodeID++;
} catch (Exception e) {
throw e;
}
}
public void removeScene(String option) throws NoSuchNodeException {
SceneNode t = null;
switch (option) {
case "a":
if ((t = cursor.getLeft()) != null) {
cursor.setLeft(cursor.getMiddle());
cursor.setMiddle(cursor.getRight());
cursor.setRight(null);
} else
throw new NoSuchNodeException("No scenes further");
break;
case "b":
if ((t = cursor.getMiddle()) != null) {
cursor.setMiddle(cursor.getRight());
cursor.setRight(null);
} else
throw new NoSuchNodeException("That option does not exist");
break;
case "c":
if ((t = cursor.getRight()) != null) {
cursor.setRight(null);
} else
throw new NoSuchNodeException("That option does not exist");
break;
default:
throw new NoSuchNodeException("Invalid input");
}
System.out.println(t.getTitle() + " removed.");
}
public void moveScene(int sceneIDToMoveTo) throws NoSuchNodeException, FullSceneException {
SceneNode n = find(sceneIDToMoveTo);
if (n == null) {
throw new NoSuchNodeException("No scene has ID #" + sceneIDToMoveTo);
}
if (cursor.getParent().getLeft() == cursor) {
cursor.getParent().setLeft(null);
} else if (cursor.getParent().getMiddle() == cursor) {
cursor.getParent().setMiddle(null);
} else if (cursor.getParent().getRight() == cursor) {
cursor.getParent().setRight(null);
} else {
throw new NoSuchNodeException("Can't move a parent below it's child");
}
try {
n.addScene(cursor);
cursor.setParent(n);
} catch (Exception e) {
throw e;
}
}
public SceneNode find(int id) {
Queue<SceneNode> q = new LinkedList<>();
if (root != null) {
q.add(root);
SceneNode t = null;
while (!q.isEmpty()) {
t = q.poll();
if (t.getSceneID() == id) {
return t;
}
if (t.getLeft() != null) {
q.add(t.getLeft());
if (t.getMiddle() != null) {
q.add(t.getMiddle());
if (t.getRight() != null) {
q.add(t.getRight());
}
}
}
}
}
return null;
}
public String getPathFromRoot() {
ArrayList<String> l = new ArrayList<>();
getPath(root, l);
String t = "";
for (int i = 0; i < l.size() - 1; i++) {
t += l.get(l.size() - i - 1) + ", ";
}
t += l.get(0);
return t;
}
public boolean getPath(SceneNode n, ArrayList<String> l) {
if (n == null) {
return false;
}
if (n.getSceneID() == cursor.getSceneID()) {
l.add(n.getTitle());
return true;
}
if (getPath(n.getLeft(), l) || getPath(n.getMiddle(), l) || getPath(n.getRight(), l)) {
l.add(n.getTitle());
return true;
} else {
return false;
}
}
public String toString() {
if (root != null) {
StringBuilder s = new StringBuilder();
rectoString(root, s, 0);
return s.toString();
} else
return "None";
}
public void rectoString(SceneNode n, StringBuilder s, int level) {
String t = "";
for (int i = 0; i <= level; i++) {
t += " ";
}
s.append(n.toString());
if (n == cursor)
s.append(" * ");
s.append("\n");
if (n.getLeft() != null) {
s.append(t + "A) ");
rectoString(n.getLeft(), s, level + 1);
if (n.getMiddle() != null) {
s.append(t + "B) ");
rectoString(n.getMiddle(), s, level + 1);
if (n.getRight() != null) {
s.append(t + "C) ");
rectoString(n.getRight(), s, level + 1);
}
}
}
}
public int getNewNodeID() {
return newNodeID;
}
public SceneNode getCursor() {
return cursor;
}
public SceneNode getRoot() {
return root;
}
public void setCursor(SceneNode cursor) {
this.cursor = cursor;
}
public void setRoot(SceneNode root) {
this.root = root;
}
}
AdventureDesigner.java
import java.util.Scanner;
public class AdventureDesigner {
private static Scanner s = new Scanner(System.in);
public static void playGame(SceneTree play) {
System.out.println("Now beginning game...\n");
SceneNode t = play.getRoot();
String option = "";
SceneNode tt = null;
while (true) {
t.displayScene();
tt = t;
if (t.isEnding()) {
break;
}
System.out.print("Please enter an option: ");
option = s.nextLine().toLowerCase();
t = (option.equals("a") ? t.getLeft()
: option.equals("b") ? t.getMiddle() : option.equals("c") ? t.getRight() : t);
if (t == tt) {
System.out.println("Invalid option, enter again");
}
System.out.println();
}
System.out.println("The End\n");
System.out.println("Returning back to creation mode...");
}
public static void showMenu() {
System.out.println();
System.out.println("A) Add Scene");
System.out.println("R) Remove Scene");
System.out.println("S) Show Current Scene");
System.out.println("P) Print Adventure Tree");
System.out.println("B) Go Back a Scene");
System.out.println("F) Go Forward a Scene");
System.out.println("G) Play Game");
System.out.println("N) Print Path To Cursor");
System.out.println("M) Move Scene");
System.out.println("Q) Quit");
System.out.println();
System.out.print("Please enter a selection: ");
}
public static void main(String[] args) {
System.out.println("Creating a Story...");
System.out.print("\nPlease enter a title: ");
String title = s.nextLine();
System.out.print("Please enter a scene: ");
String sceneDescription = s.nextLine();
SceneTree tree = new SceneTree(title, sceneDescription);
System.out.println("\nScene #1 added");
String inputCommand = "";
while (!inputCommand.equals("q")) {
showMenu();
inputCommand = s.nextLine().toLowerCase();
System.out.println();
switch (inputCommand) {
case "a":
System.out.print("Please enter a title: ");
title = s.nextLine();
System.out.print("Please enter a scene: ");
sceneDescription = s.nextLine();
System.out.println();
try {
tree.addNewNode(title, sceneDescription);
System.out.println("Scene #" + tree.getNewNodeID() + " added.");
} catch (Exception e) {
System.out.println(e.getMessage());
}
break;
case "r":
System.out.print("Please enter an option: ");
try {
tree.removeScene(s.nextLine().toLowerCase()); // printed in the function
} catch (Exception e) {
System.out.println(e.getMessage());
}
break;
case "s":
tree.getCursor().displayFullScene();
break;
case "p":
System.out.println(tree.toString());
break;
case "b":
try {
tree.moveCursorBackwords();
} catch (Exception e) {
System.out.println(e.getMessage());
}
break;
case "f":
System.out.print("Which option do you wish to go to: ");
try {
tree.moveCursorForwards(s.nextLine().toLowerCase());
} catch (Exception e) {
System.out.println();
System.out.println(e.getMessage());
}
break;
case "g":
playGame(tree);
break;
case "n":
System.out.println(tree.getPathFromRoot());
break;
case "m":
System.out.print("Move current scene to: ");
try {
tree.moveScene(Integer.parseInt(s.nextLine()));
System.out.println("\nSuccesfully moved scene.");
} catch (Exception e) {
System.out.println(e.getMessage());
}
break;
case "q":
System.out.println("Program terminating normally...");
break;
default:
System.out.println("Invalid input, enter again");
break;
}
}
}
}
FullSceneException.java
public class FullSceneException extends Exception {
public FullSceneException(String s) {
super(s);
}
}
NoSuchNodeException.java
public class NoSuchNodeException extends Exception {
public NoSuchNodeException(String s) {
super(s);
}
}
Keeping us on top of that foundation, we are creating and providing the best solutions and almost every type of coding solutions to help students.
Contact us for this java assignment Solutions by Codersarts Specialist who can help you mentor and guide for such java assignment, homework, project and coursework
If you have project or assignment files, You can send at contact@codersarts.com directly