Using the following UML, create a class called SlotMachine that simulates a casino slot machine in which three numbers between 0 and 9 are randomly selected and printed side by side. This is done using the “pullLever” method which is overloaded, a default method which uses one token, and a parameter method which can specify how many tokens to gamble for that single pull. There are five situations which occur when the lever is pulled.
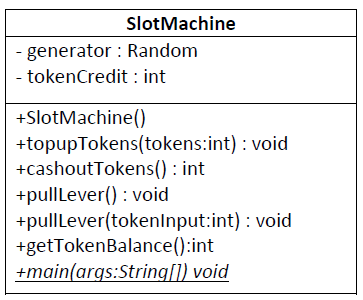
1) {0,0,0} Super Jackpot, all zeros. Token input is multiplied by a factor of 500 and added to the machine token credit. Should output suitable “Super Jackpot Winner” message showing winnings.
2) {X,X,X} Three number win. Where X is a value 1-9, token input is multiplied by a factor of 50 and added to the machine token credit. Should output suitable “Jackpot Winner” message showing winnings.
3) {X,X,Z} Free spin. Where X and Z are values 0-9 and Z≠X, token input is added back into the machine token credit. Should output suitable “Free Spin” message.
4) {X,Y,Z} Bad luck, Where X,Y,Z are values 0-9 and Z≠Y≠X, token input subtracted from machine token credit. Should output suitable “Bad Luck, Try again” message.
5) Insufficient Token Balance, where there is not enough tokens in machine to pullLever using the token input amount.
A user can also top up their machine token credit, and cashout tokens. Add a suitable main method which tests the class. It should use Scanner to repeatedly allow user to input tokens, and pull lever using the desired amount of tokens they wish gamble with. The user can exit the machine and “cash out” once they have done playing. Use suitable output to show user what is happening in the class and repeatedly show the current machine token balance.
Add another variable called houseCredit, which is used to keep track of the total amount of tokens inserted across all instances of SlotMachine, add another method called getHouseCredit, which can be used to obtain this information. Show the changes in main in a suitable way, a user should be able to jump across different slot machines, until they choose to leave the casino.
Solution : SlotMachine.java
import java.util.Random;
import java.util.Scanner;
public class SlotMachine {
private Random generator;
private int tokenCredit;
private static int houseCredit;
public SlotMachine() {
}
public void topupTokens(int tokens) {
tokenCredit =tokens;
}
public int cashoutTokens() {
return 12*tokenCredit;
}
public void pullLever() {
int slotNumber[] = new int[3];
int max = 9;
int min = 0;
for(int i=0; i<3;i++) {
slotNumber[i] =(int) ((Math.random()*((max-min)+1))+min);
}
if((slotNumber[0]==0)&&(slotNumber[1]==0)&&(slotNumber[2]==0)) {
System.out.println("Super Jackpot Winner");
tokenCredit = tokenCredit+(500*1);
}
if((slotNumber[0]==slotNumber[1])&&(slotNumber[1]==slotNumber[2])&&(slotNumber[2]==slotNumber[0])) {
System.out.println("Jackpot Winner");
tokenCredit = tokenCredit+(50*1);
}
if((slotNumber[0]==slotNumber[1])&&(slotNumber[2]>=0||slotNumber[2]<=9)) {
System.out.println("Free Spin");
tokenCredit = tokenCredit+1;
}
if((slotNumber[0]!=slotNumber[1])&&(slotNumber[1]!=slotNumber[2])&&(slotNumber[2]!=slotNumber[0])) {
System.out.println("Bad Luck");
tokenCredit = tokenCredit-1;
}
if(1<getTokenBalance())
System.out.println("Insufficient Token Balance To Pull Lever");
}
public void pullLever(int tokenInput) {
int slotNumber[] = new int[3];
int max = 9;
int min = 0;
for(int i=0; i<3;i++) {
slotNumber[i] =(int) ((Math.random()*((max-min)+1))+min);
}
if((slotNumber[0]==0)&&(slotNumber[1]==0)&&(slotNumber[2]==0)) {
System.out.println("Super Jackpot Winner");
tokenCredit = tokenCredit+(500*tokenInput);
}
else if((slotNumber[0]==slotNumber[1])&&(slotNumber[1]==slotNumber[2])&&(slotNumber[2]==slotNumber[0])) {
System.out.println("Jackpot Winner");
tokenCredit = tokenCredit+(50*tokenInput);
}
else if((slotNumber[0]==slotNumber[1])&&(slotNumber[2]>=0||slotNumber[2]<=9)) {
System.out.println("Free Spin");
tokenCredit = tokenCredit+tokenInput;
}
else if((slotNumber[0]!=slotNumber[1])&&(slotNumber[1]!=slotNumber[2])&&(slotNumber[2]!=slotNumber[0])) {
System.out.println("Bad Luck");
tokenCredit = tokenCredit-tokenInput;
}
else if(tokenInput>tokenCredit)
System.out.println("Insufficient Token Balance To Pull Lever " +getTokenBalance());
else
System.out.println("Play Again");
}
public int getTokenBalance() {
return tokenCredit;
}
public static int getHouseCredit() {
return houseCredit;
}
public static void main(String[] args) {
int cashOutToken=0;
int inputNOOfToken;
int machineTokenCredit=0;
int noOfSlotMachine=3;
int slotChoice;
int exitChoice;
int playChoice;
int addAmount;
SlotMachine SlotMachineGame[] = new SlotMachine[3];
Scanner scanInput = new Scanner(System.in);
for(int i=0 ;i<noOfSlotMachine;i++) {
SlotMachine game = new SlotMachine();
SlotMachineGame[i]= game;
}
System.out.println("No of Slot Machine in Casino : "+noOfSlotMachine);
do {
for(int i=0; i<noOfSlotMachine;i++)
System.out.println("Slot Machine " +(i+1));
System.out.println("Total amount of tokens inserted across all instances of SlotMachine " + houseCredit);
System.out.println("Enter your choice for slot machine");
slotChoice = scanInput.nextInt();
switch(slotChoice) {
case 1:
do {
System.out.println("Token Available in Machine 1 : "+SlotMachineGame[0].getTokenBalance());
System.out.println("Do you want to add amount in the Machine if yes enter 1 or else 0");
addAmount = scanInput.nextInt();
if(addAmount == 1) {
System.out.println("Enter the amount of Token to enter in machine 1: ");
machineTokenCredit = scanInput.nextInt();
machineTokenCredit = machineTokenCredit+SlotMachineGame[0].getTokenBalance();
SlotMachineGame[0].topupTokens(machineTokenCredit);
}
else {
machineTokenCredit = SlotMachineGame[0].getTokenBalance();
SlotMachineGame[0].topupTokens(machineTokenCredit);
}
System.out.println("Enter the no of Input Tokens to Gamble : ");
inputNOOfToken = scanInput.nextInt();
houseCredit = houseCredit+machineTokenCredit;
machineTokenCredit = machineTokenCredit+SlotMachineGame[0].getTokenBalance();
SlotMachineGame[0].pullLever(inputNOOfToken);
System.out.println("Do you want to play again enter 1 or else 0");
playChoice = scanInput.nextInt();
}while(playChoice==1);
break;
case 2:
do {
System.out.println("Token Available in Machine 2 : "+SlotMachineGame[1].getTokenBalance());
System.out.println("Do you want to add amount in the Machine if yes enter 1 or else 0");
addAmount = scanInput.nextInt();
if(addAmount == 1) {
System.out.println("Enter the amount of Token to enter in machine 2: ");
machineTokenCredit = scanInput.nextInt();
machineTokenCredit = machineTokenCredit+SlotMachineGame[1].getTokenBalance();
SlotMachineGame[1].topupTokens(machineTokenCredit);
}
else {
machineTokenCredit = SlotMachineGame[1].getTokenBalance();
SlotMachineGame[1].topupTokens(machineTokenCredit);
}
System.out.println("Enter the no of Input Tokens to Gamble : ");
inputNOOfToken = scanInput.nextInt();
houseCredit = houseCredit+machineTokenCredit;
machineTokenCredit = machineTokenCredit+SlotMachineGame[1].getTokenBalance();
SlotMachineGame[1].pullLever(inputNOOfToken);
System.out.println("Do you want to play again enter 1 or else 0");
playChoice = scanInput.nextInt();
}while(playChoice==1);
break;
case 3:
do {
System.out.println("Token Available in Machine 3 : "+SlotMachineGame[2].getTokenBalance());
System.out.println("Do you want to add amount in the Machine if yes enter 1 or else 0");
addAmount = scanInput.nextInt();
if(addAmount == 1) {
System.out.println("Enter the amount of Token to enter in machine 3: ");
machineTokenCredit = scanInput.nextInt();
machineTokenCredit = machineTokenCredit+SlotMachineGame[2].getTokenBalance();
SlotMachineGame[2].topupTokens(machineTokenCredit);
}
else {
machineTokenCredit = SlotMachineGame[2].getTokenBalance();
SlotMachineGame[2].topupTokens(machineTokenCredit);
}
System.out.println("Enter the no of Input Tokens to Gamble : ");
inputNOOfToken = scanInput.nextInt();
houseCredit = houseCredit+machineTokenCredit;
SlotMachineGame[2].pullLever(inputNOOfToken);
System.out.println("Do you want to play again enter 1 or else 0");
playChoice = scanInput.nextInt();
}while(playChoice==1);
break;
}
System.out.println("Do you want to Exit Casino enter 1 or else 0");
exitChoice = scanInput.nextInt();
}while(exitChoice==1);
cashOutToken = SlotMachineGame[0].cashoutTokens()+SlotMachineGame[1].cashoutTokens()+SlotMachineGame[2].cashoutTokens();
System.out.println("Cash Out the Token");
System.out.println("Token Amount is cashed Out " + cashOutToken);
}
}
Output:
No of Slot Machine in Casino : 3
Slot Machine 1
Slot Machine 2
Slot Machine 3
Total amount of tokens inserted across all instances of SlotMachine 0
Enter your choice for slot machine
1
Token Available in Machine 1 : 0
Do you want to add amount in the Machine if yes enter 1 or else 0
1
Enter the amount of Token to enter in machine 1:
40
Enter the no of Input Tokens to Gamble :
10
Bad Luck
Do you want to play again enter 1 or else 0
1
Token Available in Machine 1 : 30
Do you want to add amount in the Machine if yes enter 1 or else 0
1
Enter the amount of Token to enter in machine 1:
20
Enter the no of Input Tokens to Gamble :
30
Play Again
Do you want to play again enter 1 or else 0
1
Token Available in Machine 1 : 50
Do you want to add amount in the Machine if yes enter 1 or else 0
1
Enter the amount of Token to enter in machine 1:
5
Enter the no of Input Tokens to Gamble :
15
Bad Luck
Do you want to play again enter 1 or else 0
1
Token Available in Machine 1 : 40
Do you want to add amount in the Machine if yes enter 1 or else 0
0
Enter the no of Input Tokens to Gamble :
10
Bad Luck
Do you want to play again enter 1 or else 0
1
Token Available in Machine 1 : 30
Do you want to add amount in the Machine if yes enter 1 or else 0
0
Enter the no of Input Tokens to Gamble :
10
Bad Luck
Do you want to play again enter 1 or else 0
0
Do you want to Exit Casino enter 1 or else 0
1
Slot Machine 1
Slot Machine 2
Slot Machine 3
Total amount of tokens inserted across all instances of SlotMachine 215
Enter your choice for slot machine
1
Token Available in Machine 1 : 20
Do you want to add amount in the Machine if yes enter 1 or else 0
0
Enter the no of Input Tokens to Gamble :
10
Bad Luck
Do you want to play again enter 1 or else 0
0
Do you want to Exit Casino enter 1 or else 0
0
Cash Out the Token
Token Amount is cashed Out 120