Hi everyone, hope you are fit and fine.
Today, I will share a Basic Java Swing Project "Bus Ticket Reservation System" . This project is good for you to implement your Java Swing Knowledge.
Components of Projects :
>User Panel
> Login and Sign Up of User
> Book Tickets
> View Booking Details
>Admin Panel
> Add Bus
> Update and Delete Bus
> View Bus Details
Software & Tools Required :
1, Netbeans
2, MySql
3. Java Swing Knowledge
How To Run Project :
Open Project in netbeans
Create Database as
Username = "root"
Password = "Bhanu@123"
3. Create Database named "bus"
Add Entities
1. buses
2. user
3. ticket
4. Run Code Successfully
Follow Below Steps for Making This Project :
Create Project in netbeans "Bus Reservation System".
Create Main class
package bus.ticket.reservation.system;
/**
*
* @author BHANU UDAY
*/
public class Main {
public static void main(String s[]) {
new Registration();
}
}
Now create Helper Class for getting Connection of MySql database.
package bus.ticket.reservation.system;
/**
*
* @author BHANU UDAY
*/
import java.sql.*;
public class ConnectionProvider {
private static Connection con;
public static Connection getConnection() {
try {
if (con == null) {
//driver class load
Class.forName("com.mysql.cj.jdbc.Driver");
//create a connection..
con = DriverManager.
getConnection("jdbc:mysql://localhost:3306/bus", "root", "Bhanu@123");
}
} catch (Exception e) {
e.printStackTrace();
}
return con;
}
}
now create Registration.java class:
package bus.ticket.reservation.system;
import java.sql.*;
import java.awt.*;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.*;
import javax.swing.border.TitledBorder;
public class Registration implements ActionListener {
JFrame frame;
JPanel signup_panel, front, registration;
JPanel login_panel;
JLabel signup_username, welcome;
JLabel signup_email;
JLabel signup_password, signup_confirm_password;
JLabel login_email;
JLabel login_password;
JTextField tf_signup_username;
JTextField tf_signup_email;
JPasswordField tf_signup_password;
JPasswordField tf_signup_confirm_password;
JTextField tf_login_email;
JPasswordField tf_login_password;
JButton btn_signup, btn_login, login, signup;
Boolean isLogined;
public Registration() {
frame = new JFrame();
signup_panel = new JPanel();
front = new JPanel();
registration = new JPanel();
login_panel = new JPanel();
signup_username = new JLabel("User Name : ");
welcome = new JLabel("Welcome to Bus Ticket Reservation System");
signup_email = new JLabel("Email : ");
signup_password = new JLabel("Password : ");
signup_confirm_password = new JLabel("Confirm "
+ "Password : ");
tf_signup_username = new JTextField();
tf_signup_email = new JTextField();
tf_signup_password = new JPasswordField();
tf_signup_confirm_password = new JPasswordField();
btn_signup = new JButton("Sign Up");
signup = new JButton("Sign Up");
login = new JButton("Login");
login_email = new JLabel("Email:");
login_password = new JLabel("Password :");
tf_login_email = new JTextField();
tf_login_password = new JPasswordField();
btn_login = new JButton("Login");
signup_username.setBounds(10, 100, 120, 30);
signup_email.setBounds(10, 140, 120, 30);
signup_password.setBounds(10, 180, 120, 30);
signup_confirm_password.setBounds(10, 220, 180, 30);
tf_signup_username.setBounds(180, 100, 120, 30);
tf_signup_email.setBounds(180, 140, 120, 30);
tf_signup_password.setBounds(180, 180, 120, 30);
tf_signup_confirm_password.setBounds(180, 220, 120, 30);
btn_signup.setBounds(180, 300, 80, 40);
login_email.setBounds(10, 100, 120, 30);
login_password.setBounds(10, 140, 120, 30);
//tf_signup_username.setBounds(80, 100, 120, 30);
tf_login_email.setBounds(80, 100, 120, 30);
tf_login_password.setBounds(80, 140, 120, 30);
btn_login.setBounds(100, 220, 80, 40);
login.setBounds(200, 400, 80, 40);
signup.setBounds(400, 400, 80, 40);
login.setForeground(Color.blue);
signup.setForeground(Color.blue);
welcome.setBounds(30, 50, 700, 200);
welcome.setFont(new Font("Jokerman", Font.PLAIN, 26));
welcome.setForeground(Color.BLUE);
registration.add(welcome);
registration.add(login);
registration.add(signup);
signup_panel.setBorder(BorderFactory.createTitledBorder(BorderFactory.createEtchedBorder(), "SIGN UP FORM", TitledBorder.CENTER, TitledBorder.TOP));
login_panel.setBorder(BorderFactory.createTitledBorder(BorderFactory.createEtchedBorder(), "LOGIN FORM", TitledBorder.CENTER, TitledBorder.TOP));
signup_panel.add(signup_username);
signup_panel.add(tf_signup_username);
signup_panel.add(signup_email);
signup_panel.add(tf_signup_email);
signup_panel.add(signup_password);
signup_panel.add(tf_signup_password);
signup_panel.add(signup_confirm_password);
signup_panel.add(tf_signup_confirm_password);
signup_panel.add(btn_signup);
login_panel.add(login_email);
login_panel.add(login_password);
login_panel.add(tf_login_email);
login_panel.add(tf_login_password);
login_panel.add(btn_login);
signup_panel.setLayout(null);
login_panel.setLayout(null);
registration.setLayout(null);
front.setLayout(null);
front.setBackground(Color.BLUE);
frame.add(front);
frame.add(registration);
btn_signup.addActionListener(this);
btn_login.addActionListener(this);
login.addActionListener(this);
signup.addActionListener(this);
frame.setExtendedState(JFrame.MAXIMIZED_BOTH);
frame.setSize(600, 400);
frame.setLayout(new GridLayout(1, 2));
frame.setTitle("Welcome Page");
frame.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == signup) {
JInternalFrame f = new JInternalFrame();
f.add(signup_panel);
f.setSize(600, 800);
f.setTitle("Signup Form");
f.setClosable(true);
signup.disable();
front.add(f);
f.setVisible(true);
}
if (e.getSource() == login) {
JInternalFrame f = new JInternalFrame();
f.add(login_panel);
f.setSize(600, 800);
f.setTitle("Login Form");
f.setClosable(true);
front.add(f);
f.setVisible(true);
}
if (e.getSource() == btn_signup) {
SignUp();
}
if (e.getSource() == btn_login) {
Login();
}
}
private void SignUp() {
int x = 0;
Connection con = ConnectionProvider.getConnection();
String Username = tf_signup_username.getText();
String Email = tf_signup_email.getText();
char[] s1 = tf_signup_password.getPassword();
String Password = new String(s1);
char[] s2 = tf_signup_confirm_password.getPassword();
String CPassword = new String(s2);
if (Password.equals(CPassword)) {
try {
PreparedStatement ps = con.prepareStatement("insert into user(username,email,password) values(?,?,?)");
ps.setString(1, Username);
ps.setString(2, Email);
ps.setString(3, CPassword);
ps.executeUpdate();
x++;
if (x > 0) {
JOptionPane.showMessageDialog(btn_signup, "Data Saved Successfully");
}
} catch (Exception ex) {
System.out.println(ex);
}
} else {
JOptionPane.showMessageDialog(btn_signup, "Password Does Not Match");
}
}
private void Login() {
Connection con = ConnectionProvider.getConnection();
JFrame f1 = new JFrame();
JLabel l, l0;
String Email = tf_login_email.getText();
char[] p = tf_login_password.getPassword();
String Password = new String(p);
if (Email.equals("admin@gmail.com") && Password.equals("Admin@123")) {
new AdminPage();
} else {
try {
PreparedStatement ps = con.prepareStatement("select username from user where email=? and password=?");
ps.setString(1, Email);
ps.setString(2, Password);
ResultSet rs = ps.executeQuery();
if (rs.next()) {
new UserProfile(rs.getString(1));
frame.setVisible(false);
} else {
JOptionPane.showMessageDialog(null,
"Incorrect Email-Id or Password.."
+ "Try Again with correct detail");
}
} catch (Exception ex) {
System.out.println(ex);
}
}
}
}

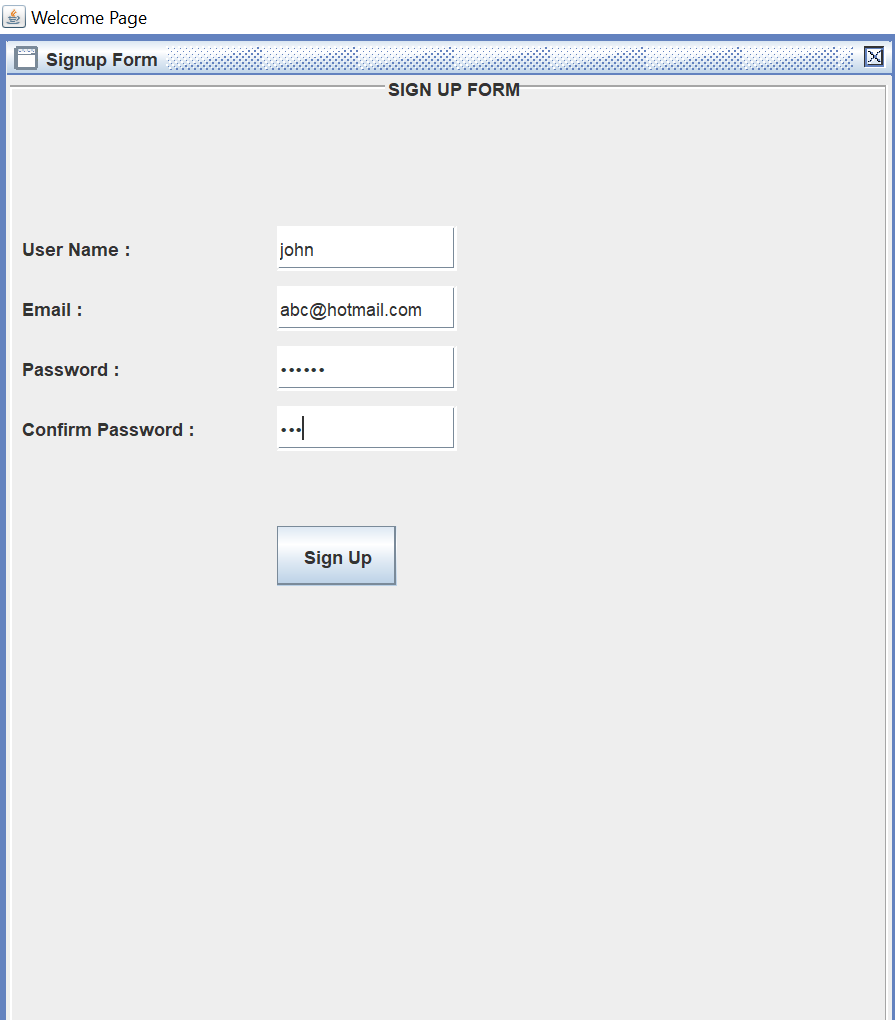
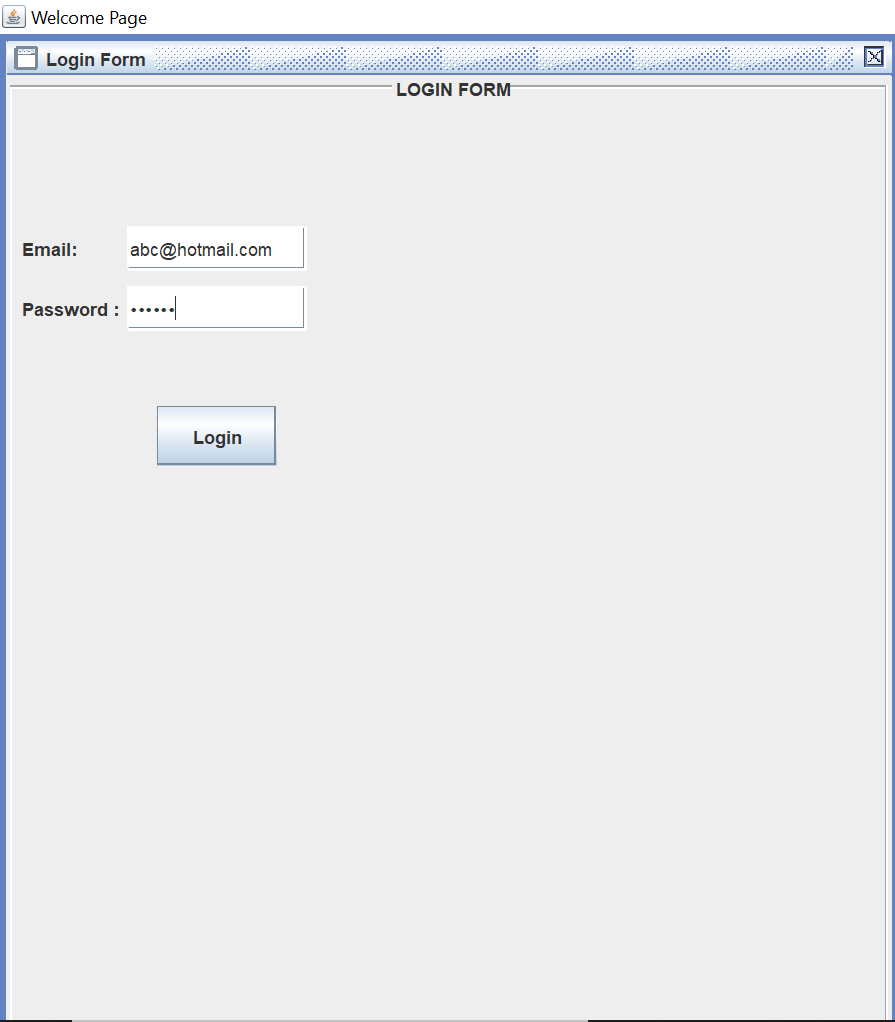
Create UserProfile.java to display User information
package bus.ticket.reservation.system;
import java.awt.Color;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JDesktopPane;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
/**
*
* @author BHANU UDAY
*/
public class UserProfile implements ActionListener {
JDesktopPane desktop;
JFrame f;
JPanel panel, top_panel;
JLabel l = new JLabel();
JMenu book_ticket, view_history, logout, exit;
JMenuItem btn_ticket, btn_history, btn_exit, btn_logout, i5;
UserProfile(String Username) {
f = new JFrame();
panel = new JPanel();
top_panel = new JPanel();
JMenuBar mb = new JMenuBar();
desktop = new JDesktopPane();
book_ticket = new JMenu("Book Ticket");
view_history = new JMenu("View Previous Booket Ticket");
logout = new JMenu("Logout");
exit = new JMenu("Exit");
mb.setBackground(Color.blue);
book_ticket.setForeground(Color.white);
view_history.setForeground(Color.white);
logout.setForeground(Color.white);
exit.setForeground(Color.white);
btn_ticket = new JMenuItem("Book Ticket");
btn_history = new JMenuItem("History");
btn_exit = new JMenuItem("Exit");
btn_logout = new JMenuItem("Logout");
i5 = new JMenuItem("i");
book_ticket.add(btn_ticket);
view_history.add(btn_history);
exit.add(btn_exit);
logout.add(btn_logout);
f.setContentPane(desktop);
mb.add(book_ticket);
mb.add(view_history);
mb.add(logout);
mb.add(exit);
f.setJMenuBar(mb);
btn_ticket.addActionListener(this);
btn_history.addActionListener(this);
btn_logout.addActionListener(this);
btn_exit.addActionListener(this);
f.setExtendedState(JFrame.MAXIMIZED_BOTH);
f.setTitle("Welcome " + Username);
f.setSize(300, 300);
f.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btn_ticket) {
SelectBus b = new SelectBus();
desktop.add(b);
}
if (e.getSource() == btn_history) {
History h = new History();
desktop.add(h);
}
if (e.getSource() == btn_logout) {
new Registration();
f.dispose();
}
if (e.getSource() == btn_exit) {
f.dispose();
}
}
public static void main(String s[]) {
System.out.println("connection" + ConnectionProvider.getConnection());
new UserProfile("bhanu");
}
}

Select.java class to select bus :
package bus.ticket.reservation.system;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableColumn;
/**
*
* @author BHANU UDAY
*/
public class SelectBus extends JInternalFrame implements ActionListener {
// JFrame f;
JPanel select_bus,list_of_available_buses;
JDesktopPane desktop;
ResultSetTableModel tableModel;
JLabel source,destination;
JTextField tf_source,tf_destination;
JButton btn_check;
JTable available_buses;
DefaultTableModel dm=new DefaultTableModel();
String[] col ={"BusID","Source","Destination","TotalSeats"};
private JPanel northPanel = new JPanel();
//for creating the Center Panel
private JPanel centerPanel = new JPanel();
//for creating the label
private JLabel northLabel = new JLabel("THE LIST FOR THE BUSES");
//for creating the button
private JButton printButton;
//for creating the table
private JTable table;
//for creating the TableColumn
private TableColumn column = null;
//for creating the JScrollPane
private JScrollPane scrollPane;
public SelectBus() {
super("Select Bus",true,true,true);
//f = new JFrame();
select_bus = new JPanel();
list_of_available_buses = new JPanel();
source = new JLabel("From : ");
destination = new JLabel("To : ");
tf_source = new JTextField();
tf_destination = new JTextField();
desktop = new JDesktopPane();
setContentPane(desktop);
dm.addColumn("Id");
dm.addColumn("source");
dm.addColumn("destination");
dm.addColumn("total seats");
dm.setColumnIdentifiers(col);
available_buses = new JTable(dm);
//dm.setColumnIdentifiers(col);
Container cnt = getContentPane();
btn_check = new JButton("Check");
source.setBounds(20,20,100,20);
destination.setBounds(20,60,100,20);
tf_source.setBounds(120,20,100,20);
tf_destination.setBounds(120,60,100,20);
btn_check.setBounds(100,100,80,40);
select_bus.add(source);
select_bus.add(tf_source);
select_bus.add(destination);
select_bus.add(tf_destination);
select_bus.add(btn_check);
list_of_available_buses.setBackground(Color.BLUE);
//list_of_available_buses.add(available_buses);
add(select_bus);
btn_check.addActionListener(this);
select_bus.setSize(1000,300);
select_bus.setLayout(null);
setVisible(true);
setLayout(new GridLayout(1,2));
setSize(600,900);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btn_check) {
JFrame in = new JFrame("list of available buses");
String Source = tf_source.getText();
String Destination = tf_destination.getText();
Connection con = ConnectionProvider.getConnection();
String Query = "Select busID,source,destination,numberofseats from buses where source ='" + Source + "' and destination ='"+Destination +"';";
System.out.println(Query);
try {
tableModel = new ResultSetTableModel(/*JDBC_DRIVER,*/con, Query);
//for setting the Query
try {
tableModel.setQuery(Query);
} catch (SQLException sqlException) {
}
} catch (ClassNotFoundException classNotFound) {
System.out.println(classNotFound.toString());
} catch (SQLException sqlException) {
System.out.println(sqlException.toString());
}
//Container cp = in.getContentPane();
table = new JTable(tableModel);
//for setting the size for the table
table.setPreferredScrollableViewportSize(new Dimension(990, 200));
//for setting the font
table.setFont(new Font("Tahoma", Font.PLAIN, 12));
//for setting the scrollpane to the table
scrollPane = new JScrollPane(table);
//for setting the size for the table columns
northLabel.setFont(new Font("Tahoma", Font.BOLD, 14));
//for setting the layout to the panel
northPanel.setLayout(new FlowLayout(FlowLayout.CENTER));
//for adding the label to the panel
northPanel.add(northLabel);
//for adding the panel to the container
in.add(northPanel);
//for setting the layout to the panel
centerPanel.setLayout(new BorderLayout());
//for creating an image for the button
//ImageIcon printIcon = new ImageIcon(ClassLoader.getSystemResource("images/Print16.gif"));
//for adding the button to the panel
printButton = new JButton("print the buses");
//for setting the tip text
printButton.setToolTipText("Print");
//for setting the font to the button
printButton.setFont(new Font("Tahoma", Font.PLAIN, 12));
//for adding the button to the panel
centerPanel.add(printButton, BorderLayout.NORTH);
//for adding the scrollpane to the panel
centerPanel.add(scrollPane, BorderLayout.CENTER);
//for setting the border to the panel
centerPanel.setBorder(BorderFactory.createTitledBorder("Items:"));
//for adding the panel to the container
in.add(centerPanel);
//add(list_of_available_buses);
//list_of_available_buses.setLayout(new FlowLayout());
//list_of_available_buses.setSize(500,500);
//for adding the actionListener to the button
/*printButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent ae) {
Thread runner = new Thread() {
public void run() {
try {
PrinterJob prnJob = PrinterJob.getPrinterJob();
//prnJob.setPrintable(new PrintingItems(DEFAULT_QUERY));
if (!prnJob.printDialog())
return;
setCursor(Cursor.getPredefinedCursor(Cursor.WAIT_CURSOR));
prnJob.print();
setCursor(Cursor.getPredefinedCursor(Cursor.DEFAULT_CURSOR));
}
catch (PrinterException ex) {
System.out.println("Printing error: " + ex.toString());
}
}
};
runner.start();
}
});*/
//for setting the visible to true
//desk.top.add(b);
in.setVisible(true);
in.setSize(600, 600);
table.addMouseListener(new MouseListener() {
@Override
public void mouseReleased(MouseEvent e) {
}
@Override
public void mousePressed(MouseEvent e) {
int index = table.getSelectedRow();
String id = tableModel.getValueAt(index, 0).toString();
String s = tableModel.getValueAt(index, 1).toString();
String d = tableModel.getValueAt(index, 2).toString();
String t = tableModel.getValueAt(index, 3).toString();
//String selectedCellValue = (String) table.getValueAt(table.getSelectedRow() , table.getSelectedColumn());
System.out.println(id +" "+s+" "+d+" "+t);
BookTicket b = new BookTicket(id,s,d,t);
// desktop.add(b);
}
@Override
public void mouseExited(MouseEvent e) {
}
@Override
public void mouseEntered(MouseEvent e) {
}
@Override
public void mouseClicked(MouseEvent e) {
}
});
}
}
public static void main(String s[])
{
System.out.println("connection"+ ConnectionProvider.getConnection());
new SelectBus();
}
}
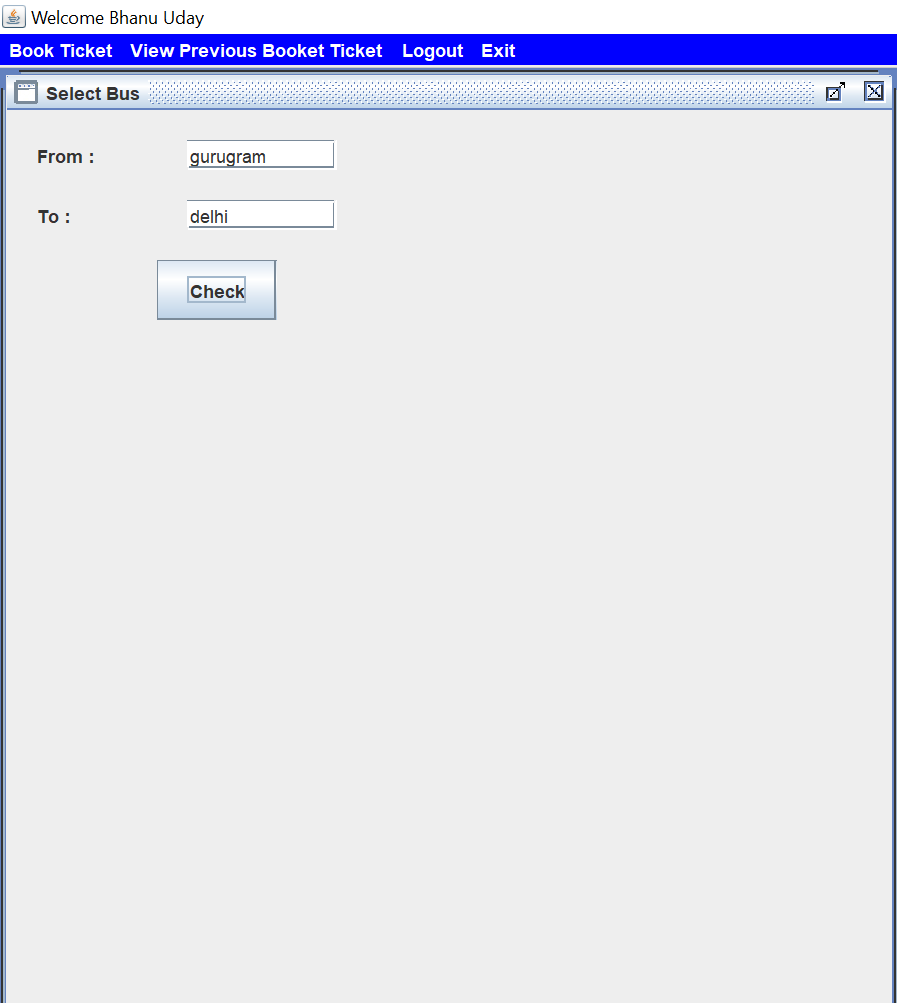

BookTicket.java
package bus.ticket.reservation.system;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.PreparedStatement;
import javax.swing.JInternalFrame;
import javax.swing.*;
/**
*
* @author BHANU UDAY
*/
public class BookTicket extends JFrame implements ActionListener {
JLabel customer_name, seat_number, numberofseats;
JTextField txt_c_name;
JPanel seat_panel;
JPanel p;
JLabel source, destination, seatnumber, name, price;
JTextField tf_source, tf_destination, tf_seatnumber, tf_name, tf_price;
JButton btn_book;
String ID;
public BookTicket(String id, String Source, String Destination, String totalseats) {
//super("Book Ticket", true, true, true);
p = new JPanel();
ID = id;
source = new JLabel("From : ");
source.setText(Source);
destination = new JLabel("To : ");
destination.setText(Destination);
name = new JLabel("Customer Name");
seatnumber = new JLabel(" Enter Seat Number ");
price = new JLabel("Price : ");
tf_source = new JTextField();
tf_name = new JTextField();
tf_source.setText(Source);
tf_destination = new JTextField();
tf_destination.setText(Destination);
tf_seatnumber = new JTextField();
tf_price = new JTextField();
String y = "1000";
tf_price.setText(y);
btn_book = new JButton("Book Ticket");
source.setBounds(20, 20, 100, 30);
destination.setBounds(20, 60, 100, 30);
seatnumber.setBounds(20, 100, 100, 30);
name.setBounds(20, 140, 100, 30);
price.setBounds(20, 180, 100, 30);
tf_source.setBounds(140, 20, 100, 30);
tf_destination.setBounds(140, 60, 100, 30);
tf_seatnumber.setBounds(140, 100, 100, 30);
tf_name.setBounds(140, 140, 100, 30);
tf_price.setBounds(140, 180, 100, 30);
btn_book.setBounds(80, 220, 120, 30);
p.add(source);
p.add(destination);
p.add(seatnumber);
p.add(tf_source);
p.add(tf_destination);
p.add(tf_seatnumber);
p.add(btn_book);
p.add(name);
p.add(tf_name);
p.add(price);
p.add(tf_price);
btn_book.addActionListener(this);
add(p);
p.setLayout(null);
setVisible(true);
setSize(600, 600);
//JInternalFrame f = new JInternalFrame();
setVisible(true);
setSize(600, 600);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btn_book) {
int x = 0;
Connection con = ConnectionProvider.getConnection();
String Price = tf_price.getText();
//String Destination = tf_destination.getText();
String SeatNumber = tf_seatnumber.getText();
String CName = tf_name.getText();
try {
PreparedStatement ps = con.prepareStatement("insert into ticket(busID,seatNumber,cName,total_price) values(?,?,?,?)");
ps.setString(1, ID);
ps.setString(2, SeatNumber);
ps.setString(3, CName);
ps.setString(4, Price);
ps.executeUpdate();
x++;
if (x > 0) {
JOptionPane.showMessageDialog(btn_book, "Ticket Book");
}
} catch (Exception ex) {
System.out.println(ex);
}
} else {
JOptionPane.showMessageDialog(btn_book, "Something went Wrong");
}
}
}
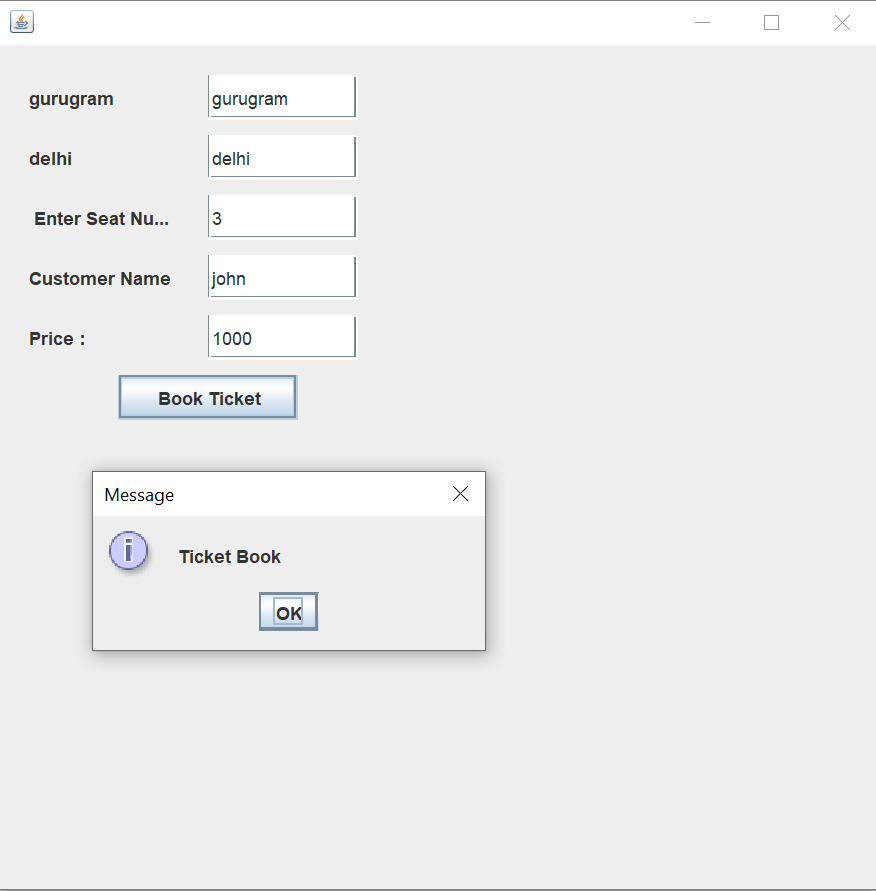
History.java
package bus.ticket.reservation.system;
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.Cursor;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.print.PrinterException;
import java.awt.print.PrinterJob;
import java.sql.Connection;
import java.sql.SQLException;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JInternalFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.TableColumn;
/**
*
* @author BHANU UDAY
*/
public class History extends JInternalFrame {
private JPanel northPanel = new JPanel();
//for creating the Center Panel
private JPanel centerPanel = new JPanel();
//for creating the label
private JLabel northLabel = new JLabel("THE LIST FOR THE ITEMS");
//for creating the button
private JButton printButton;
//for creating the table
private JTable table;
//for creating the TableColumn
private TableColumn column = null;
//for creating the JScrollPane
private JScrollPane scrollPane;
//for creating an object for the ResultSetTableModel class
private ResultSetTableModel tableModel;
private Connection DATABASE_URL = ConnectionProvider.getConnection();
private static final String DEFAULT_QUERY = "select * from ticket";
public History() {
super("All Buses", true, true, true);
//available_buses.setAutoResizeMode(JTable.AUTO_RESIZE_ALL_COLUMNS);
// available_buses.setFillsViewportHeight(true);
Container cp = getContentPane();
try {
tableModel = new ResultSetTableModel(/*JDBC_DRIVER,*/DATABASE_URL, DEFAULT_QUERY);
//for setting the Query
try {
tableModel.setQuery(DEFAULT_QUERY);
} catch (SQLException sqlException) {
}
} catch (ClassNotFoundException classNotFound) {
System.out.println(classNotFound.toString());
} catch (SQLException sqlException) {
System.out.println(sqlException.toString());
}
//for setting the table with the information
table = new JTable(tableModel);
//for setting the size for the table
table.setPreferredScrollableViewportSize(new Dimension(990, 200));
//for setting the font
table.setFont(new Font("Tahoma", Font.PLAIN, 12));
//for setting the scrollpane to the table
scrollPane = new JScrollPane(table);
//for setting the size for the table columns
northLabel.setFont(new Font("Tahoma", Font.BOLD, 14));
//for setting the layout to the panel
northPanel.setLayout(new FlowLayout(FlowLayout.CENTER));
//for adding the label to the panel
northPanel.add(northLabel);
//for adding the panel to the container
cp.add("North", northPanel);
//for setting the layout to the panel
centerPanel.setLayout(new BorderLayout());
//for creating an image for the button
//ImageIcon printIcon = new ImageIcon(ClassLoader.getSystemResource("images/Print16.gif"));
//for adding the button to the panel
printButton = new JButton("print the buses");
//for setting the tip text
printButton.setToolTipText("Print");
//for setting the font to the button
printButton.setFont(new Font("Tahoma", Font.PLAIN, 12));
//for adding the button to the panel
centerPanel.add(printButton, BorderLayout.NORTH);
//for adding the scrollpane to the panel
centerPanel.add(scrollPane, BorderLayout.CENTER);
//for setting the border to the panel
centerPanel.setBorder(BorderFactory.createTitledBorder("Items:"));
//for adding the panel to the container
cp.add("Center", centerPanel);
//for adding the actionListener to the button
printButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent ae) {
Thread runner = new Thread() {
public void run() {
try {
PrinterJob prnJob = PrinterJob.getPrinterJob();
prnJob.setPrintable(new PrintingBuses(DEFAULT_QUERY,DATABASE_URL));
if (!prnJob.printDialog())
return;
setCursor(Cursor.getPredefinedCursor(Cursor.WAIT_CURSOR));
prnJob.print();
setCursor(Cursor.getPredefinedCursor(Cursor.DEFAULT_CURSOR));
}
catch (PrinterException ex) {
System.out.println("Printing error: " + ex.toString());
}
}
};
runner.start();
}
});
//for setting the visible to true
setVisible(true);
//to show the frame
pack();
}
}
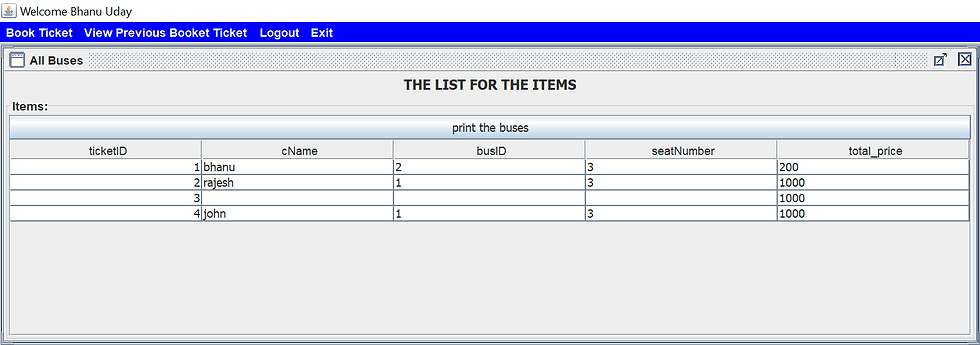
Now Create Admin Panel as AdminPage.java
package bus.ticket.reservation.system;
import java.awt.Color;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JDesktopPane;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
/**
*
* @author BHANU UDAY
*/
public class AdminPage implements ActionListener {
JDesktopPane desktop;
JFrame f;
JPanel panel, top_panel;
JLabel l = new JLabel();
JMenu bus, customer, logout, exit;
JMenuItem addBus, viewBus, removeBus, updateBus, viewCustomers, exit_admin, logout_admin;
public AdminPage() {
f = new JFrame();
panel = new JPanel();
top_panel = new JPanel();
JMenuBar mb = new JMenuBar();
bus = new JMenu("Bus");
customer = new JMenu("Customers");
logout = new JMenu("Logout");
exit = new JMenu("Exit");
mb.setBackground(Color.blue);
bus.setForeground(Color.white);
customer.setForeground(Color.white);
logout.setForeground(Color.white);
exit.setForeground(Color.white);
addBus = new JMenuItem("Add");
addBus.setBackground(Color.WHITE);
viewBus = new JMenuItem("View Buses");
removeBus = new JMenuItem("Remove Bus");
updateBus = new JMenuItem("Update Bus");
exit_admin = new JMenuItem("Exit");
logout_admin = new JMenuItem("Logout");
viewCustomers = new JMenuItem("View All Customers");
desktop = new JDesktopPane();
f.setContentPane(desktop);
bus.add(addBus);
bus.add(viewBus);
bus.add(removeBus);
bus.add(updateBus);
customer.add(viewCustomers);
exit.add(exit_admin);
logout.add(logout_admin);
mb.add(bus);
mb.add(customer);
mb.add(logout);
mb.add(exit);
f.setJMenuBar(mb);
addBus.addActionListener(this);
viewBus.addActionListener(this);
removeBus.addActionListener(this);
updateBus.addActionListener(this);
viewCustomers.addActionListener(this);
exit_admin.addActionListener(this);
logout_admin.addActionListener(this);
f.setExtendedState(JFrame.MAXIMIZED_BOTH);
f.setTitle("Welcome ");
f.setSize(300, 300);
f.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addBus) {
AddBus a = new AddBus();
desktop.add(a);
}
if (e.getSource() == viewBus) {
ViewBus v = new ViewBus();
desktop.add(v);
}
if (e.getSource() == removeBus) {
RemoveBus r = new RemoveBus();
desktop.add(r);
}
if (e.getSource() == updateBus) {
UpdateBus u = new UpdateBus();
desktop.add(u);
}
if (e.getSource() == logout_admin) {
new Registration();
f.dispose();
}
if (e.getSource() == exit_admin) {
f.dispose();
}
}
}
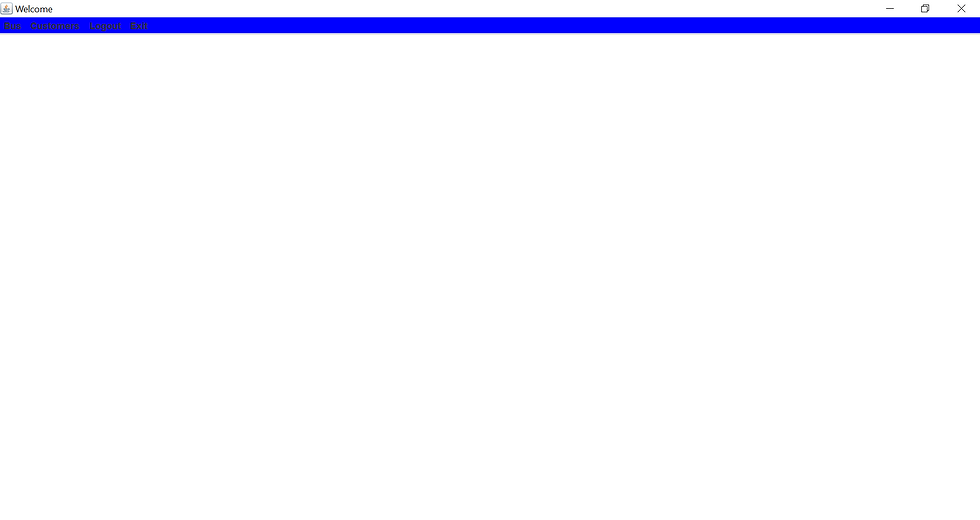
Now Create AddBuss.java
package bus.ticket.reservation.system;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.PreparedStatement;
import javax.swing.*;
/**
*
* @author BHANU UDAY
*/
public class AddBus extends JInternalFrame implements ActionListener {
JPanel p;
JLabel source, destination, total_seats;
JTextField tf_source, tf_destination, tf_total_seats;
JButton btn_add;
public AddBus() {
//f = new JFrame();
super("Add Bus", true, true, true);
p = new JPanel();
source = new JLabel("From : ");
destination = new JLabel("To : ");
total_seats = new JLabel(" Total Seats : ");
tf_source = new JTextField();
tf_destination = new JTextField();
tf_total_seats = new JTextField();
btn_add = new JButton("Add Bus");
source.setBounds(20, 20, 100, 30);
destination.setBounds(20, 60, 100, 30);
total_seats.setBounds(20, 100, 100, 30);
tf_source.setBounds(140, 20, 100, 30);
tf_destination.setBounds(140, 60, 100, 30);
tf_total_seats.setBounds(140, 100, 100, 30);
btn_add.setBounds(80, 140, 120, 30);
p.add(source);
p.add(destination);
p.add(total_seats);
p.add(tf_source);
p.add(tf_destination);
p.add(tf_total_seats);
p.add(btn_add);
btn_add.addActionListener(this);
add(p);
p.setLayout(null);
setVisible(true);
setSize(600, 600);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btn_add) {
int x = 0;
Connection con = ConnectionProvider.getConnection();
String Source = tf_source.getText();
String Destination = tf_destination.getText();
String TotalSeat = tf_total_seats.getText();
try {
PreparedStatement ps = con.prepareStatement("insert into buses(source,destination,numberofseats) values(?,?,?)");
ps.setString(1, Source);
ps.setString(2, Destination);
ps.setString(3, TotalSeat);
ps.executeUpdate();
x++;
if (x > 0) {
JOptionPane.showMessageDialog(btn_add, "Bus Added Successfully");
}
} catch (Exception ex) {
System.out.println(ex);
}
} else {
JOptionPane.showMessageDialog(btn_add, "Something went Wrong");
}
}
}
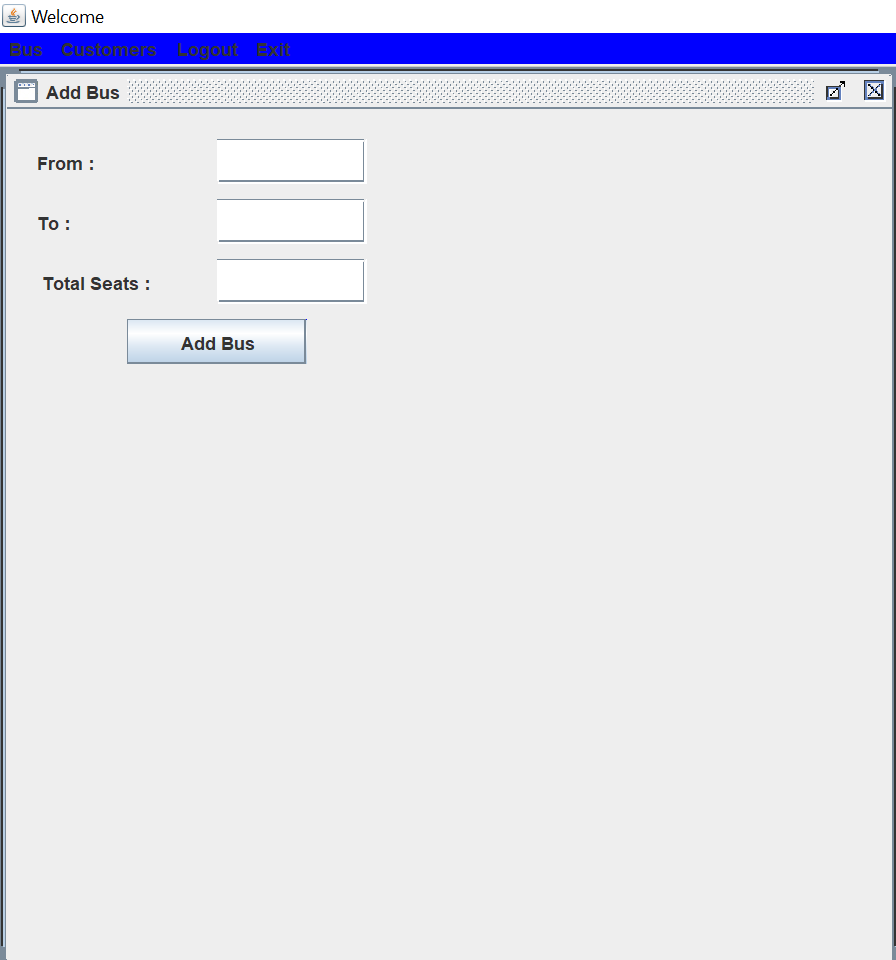
Now Create ViewBus.java
package bus.ticket.reservation.system;
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.Cursor;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.print.PrinterException;
import java.awt.print.PrinterJob;
import java.sql.Connection;
import java.sql.SQLException;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JInternalFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.TableColumn;
/**
*
* @author BHANU UDAY
*/
public class ViewBus extends JInternalFrame {
private JPanel northPanel = new JPanel();
//for creating the Center Panel
private JPanel centerPanel = new JPanel();
//for creating the label
private JLabel northLabel = new JLabel("THE LIST FOR THE ITEMS");
//for creating the button
private JButton printButton;
//for creating the table
private JTable table;
//for creating the TableColumn
private TableColumn column = null;
//for creating the JScrollPane
private JScrollPane scrollPane;
//for creating an object for the ResultSetTableModel class
private ResultSetTableModel tableModel;
private Connection DATABASE_URL = ConnectionProvider.getConnection();
private static final String DEFAULT_QUERY = "select * from buses";
public ViewBus() {
super("All Buses", true, true, true);
//available_buses.setAutoResizeMode(JTable.AUTO_RESIZE_ALL_COLUMNS);
// available_buses.setFillsViewportHeight(true);
Container cp = getContentPane();
try {
tableModel = new ResultSetTableModel(/*JDBC_DRIVER,*/DATABASE_URL, DEFAULT_QUERY);
//for setting the Query
try {
tableModel.setQuery(DEFAULT_QUERY);
} catch (SQLException sqlException) {
}
} catch (ClassNotFoundException classNotFound) {
System.out.println(classNotFound.toString());
} catch (SQLException sqlException) {
System.out.println(sqlException.toString());
}
//for setting the table with the information
table = new JTable(tableModel);
//for setting the size for the table
table.setPreferredScrollableViewportSize(new Dimension(990, 200));
//for setting the font
table.setFont(new Font("Tahoma", Font.PLAIN, 12));
//for setting the scrollpane to the table
scrollPane = new JScrollPane(table);
//for setting the size for the table columns
northLabel.setFont(new Font("Tahoma", Font.BOLD, 14));
//for setting the layout to the panel
northPanel.setLayout(new FlowLayout(FlowLayout.CENTER));
//for adding the label to the panel
northPanel.add(northLabel);
//for adding the panel to the container
cp.add("North", northPanel);
//for setting the layout to the panel
centerPanel.setLayout(new BorderLayout());
//for creating an image for the button
//ImageIcon printIcon = new ImageIcon(ClassLoader.getSystemResource("images/Print16.gif"));
//for adding the button to the panel
printButton = new JButton("print the buses");
//for setting the tip text
printButton.setToolTipText("Print");
//for setting the font to the button
printButton.setFont(new Font("Tahoma", Font.PLAIN, 12));
//for adding the button to the panel
centerPanel.add(printButton, BorderLayout.NORTH);
//for adding the scrollpane to the panel
centerPanel.add(scrollPane, BorderLayout.CENTER);
//for setting the border to the panel
centerPanel.setBorder(BorderFactory.createTitledBorder("Items:"));
//for adding the panel to the container
cp.add("Center", centerPanel);
//for adding the actionListener to the button
printButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent ae) {
Thread runner = new Thread() {
public void run() {
try {
PrinterJob prnJob = PrinterJob.getPrinterJob();
prnJob.setPrintable(new PrintingBuses(DEFAULT_QUERY, DATABASE_URL));
if (!prnJob.printDialog())
return;
setCursor(Cursor.getPredefinedCursor(Cursor.WAIT_CURSOR));
prnJob.print();
setCursor(Cursor.getPredefinedCursor(Cursor.DEFAULT_CURSOR));
}
catch (PrinterException ex) {
System.out.println("Printing error: " + ex.toString());
}
}
};
runner.start();
}
});
//for setting the visible to true
setVisible(true);
//to show the frame
pack();
}
public static void main(String s[]) {
System.out.println("connection" + ConnectionProvider.getConnection());
new SelectBus();
}
}
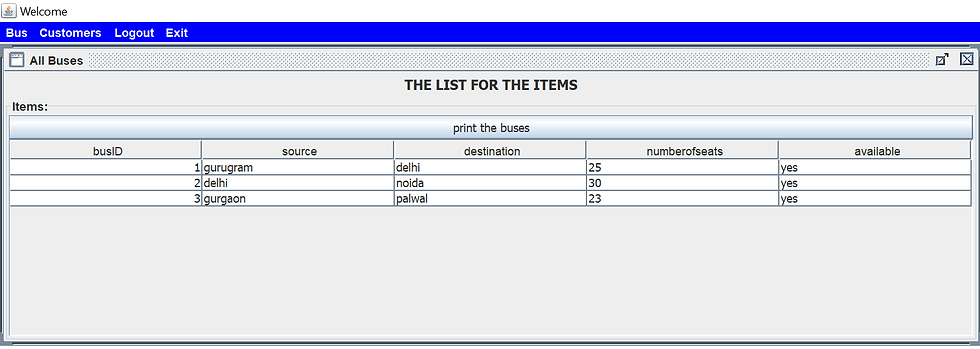
RemoveBus.java
package bus.ticket.reservation.system;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.PreparedStatement;
import javax.swing.*;
/**
*
* @author BHANU UDAY
*/
public class RemoveBus extends JInternalFrame implements ActionListener {
JPanel p;
JLabel id;
JTextField tf_id;
JButton btn_delete;
public RemoveBus() {
super("Add Bus", true, true, true);
//f = new JFrame();
p = new JPanel();
id = new JLabel("Bus Id : ");
tf_id = new JTextField();
btn_delete = new JButton("Delete Bus");
id.setBounds(20, 20, 100, 30);
tf_id.setBounds(140, 20, 100, 30);
btn_delete.setBounds(80, 60, 80, 40);
p.add(id);
p.add(tf_id);
p.add(btn_delete);
btn_delete.addActionListener(this);
add(p);
p.setLayout(null);
setVisible(true);
setSize(600, 600);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btn_delete) {
Connection con = ConnectionProvider.getConnection();
try {
PreparedStatement ps = con.prepareStatement("delete from buses where busID =" + tf_id.getText());
ps.executeUpdate();
JOptionPane.showMessageDialog(btn_delete, "Deleted Succesfully");
} catch (Exception ex) {
System.out.println(ex);
}
} else {
JOptionPane.showMessageDialog(btn_delete, "Something went Wrong");
}
}
}
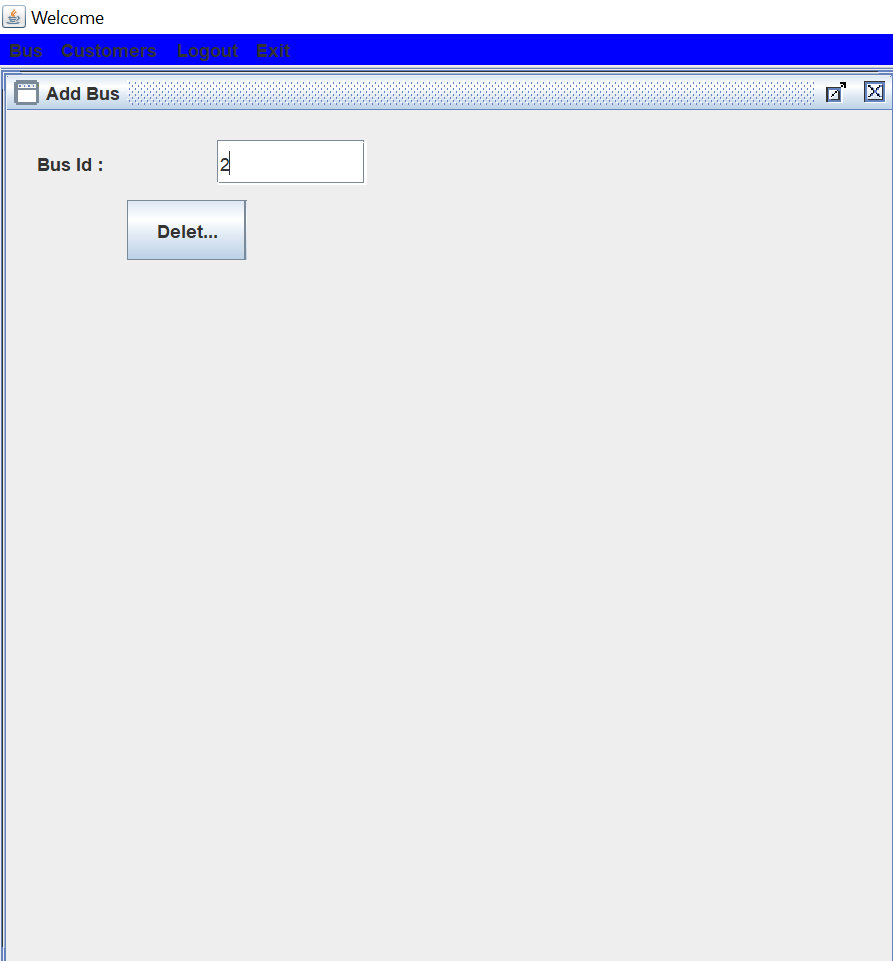
UpdateBus.java
package bus.ticket.reservation.system;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.*;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JInternalFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
/**
*
* @author BHANU UDAY
*/
public class UpdateBus extends JInternalFrame implements ActionListener {
JFrame f;
JPanel p;
JLabel source, destination, total_seats;
JTextField tf_source, tf_destination, tf_total_seats;
JButton btn_update;
JLabel id;
JTextField tf_id;
JButton btn_search;
public UpdateBus() {
super("Add Bus", true, true, true);
//f = new JFrame();
p = new JPanel();
source = new JLabel("From : ");
destination = new JLabel("To : ");
total_seats = new JLabel(" Total Seats : ");
tf_source = new JTextField();
tf_destination = new JTextField();
tf_total_seats = new JTextField();
btn_update = new JButton("Update Bus");
id = new JLabel("Bus Id : ");
tf_id = new JTextField();
btn_search = new JButton("Search Bus");
id.setBounds(20, 20, 100, 30);
tf_id.setBounds(140, 20, 100, 30);
btn_search.setBounds(80, 60, 140, 40);
source.setBounds(20, 100, 100, 30);
destination.setBounds(20, 140, 100, 30);
total_seats.setBounds(20, 180, 100, 30);
tf_source.setBounds(140, 100, 100, 30);
tf_destination.setBounds(140, 140, 100, 30);
tf_total_seats.setBounds(140, 180, 100, 30);
btn_update.setBounds(80, 220, 100, 40);
p.add(id);
p.add(tf_id);
p.add(btn_search);
p.add(source);
p.add(destination);
p.add(total_seats);
p.add(tf_source);
p.add(tf_destination);
p.add(tf_total_seats);
p.add(btn_update);
btn_update.addActionListener(this);
btn_search.addActionListener(this);
add(p);
p.setLayout(null);
setVisible(true);
setSize(600, 600);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btn_search) {
int x = 0;
Connection con = ConnectionProvider.getConnection();
String _id = tf_id.getText();
try {
PreparedStatement ps = con.prepareStatement("select * from buses where busID = ?");
ps.setString(1, _id);
ResultSet rs = ps.executeQuery();
if (rs.next()) {
tf_source.setText(rs.getString("source"));
tf_destination.setText(rs.getString("destination"));
tf_total_seats.setText(rs.getString("numberofseats"));
}
} catch (Exception ex) {
System.out.println(ex);
}
}
//desktop.add(b);
if (e.getSource() == btn_update) {
int x = 0;
Connection con = ConnectionProvider.getConnection();
String Source = tf_source.getText();
String Destination = tf_destination.getText();
String TotalSeats = tf_total_seats.getText();
try {
PreparedStatement ps = con.prepareStatement("update buses set source ='" + Source + "',destination ='" + Destination + "',numberofseats ='" + TotalSeats + "'where busID = " + tf_id.getText());
ps.executeUpdate();
} catch (Exception ex) {
System.out.println(ex);
}
}
}
public static void main(String s[]) {
System.out.println("connection ; " + ConnectionProvider.getConnection());
new UpdateBus();
}
}
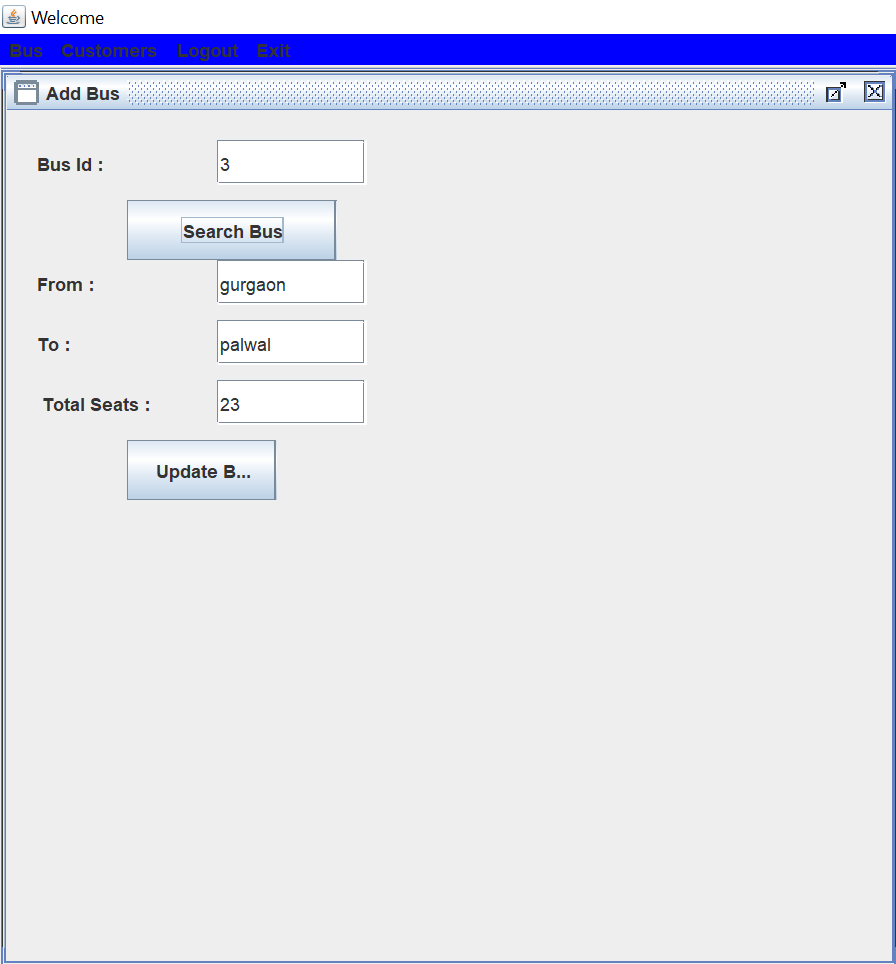
ResultSetTableModel
package bus.ticket.reservation.system;
import static java.lang.String.valueOf;
import javax.swing.table.AbstractTableModel;
import java.sql.*;
/**
* ResultSet rows and columns are counted from 1 and JTable rows and columns are
* counted from 0. When processing ResultSet rows or columns for use in a
* JTable, it is necessary to add 1 to the row or column number to manipulate
* the appropriate ResultSet column (i.e., JTable column 0 is ResultSet column 1
* and JTable row 0 is ResultSet row 1)
*/
public class ResultSetTableModel extends AbstractTableModel {
private Connection connection;
private Statement statement;
private ResultSet resultSet;
private ResultSetMetaData metaData;
private int numberOfRows;
// keep track of database connection status
private boolean connectedToDatabase = false;
// private Databases d = new Databases();
// initialize resultSet and obtain its meta data object;
// determine number of rows
public ResultSetTableModel(/*String driver, */Connection url, String query) throws SQLException, ClassNotFoundException {
//Class.forName(driver); // load database driver class
connection = url; // connect to database
// create Statement to query database
statement = connection.createStatement(ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_READ_ONLY);
connectedToDatabase = true; // update database connection status
setQuery(query); // set query and execute it
}
// get class that represents column type
public Class getColumnClass(int column) throws IllegalStateException {
// ensure database connection is available
if (!connectedToDatabase) {
throw new IllegalStateException("Not Connected to Database");
}
// determine Java class of column
try {
String className = metaData.getColumnClassName(column + 1);
return Class.forName(className); // return Class object that represents className
} // catch SQLExceptions and ClassNotFoundExceptions
catch (Exception exception) {
exception.printStackTrace();
}
// if problems occur above, assume type Object
return Object.class;
}
// get number of columns in ResultSet
public int getColumnCount() throws IllegalStateException {
// ensure database connection is available
if (!connectedToDatabase) {
throw new IllegalStateException("Not Connected to Database");
}
// determine number of columns
try {
return metaData.getColumnCount();
} // catch SQLExceptions and print error message
catch (SQLException sqlException) {
sqlException.printStackTrace();
}
// if problems occur above, return 0 for number of columns
return 0;
}
// get name of a particular column in ResultSet
public String getColumnName(int column) throws IllegalStateException {
// ensure database connection is available
if (!connectedToDatabase) {
throw new IllegalStateException("Not Connected to Database");
}
// determine column name
try {
return metaData.getColumnName(column + 1);
} // catch SQLExceptions and print error message
catch (SQLException sqlException) {
sqlException.printStackTrace();
}
// if problems, return empty string for column name
return "";
}
// return number of rows in ResultSet
public int getRowCount() throws IllegalStateException {
// ensure database connection is available
if (!connectedToDatabase) {
throw new IllegalStateException("Not Connected to Database");
}
return numberOfRows;
}
// obtain value in particular row and column
public Object getValueAt(int row, int column) throws IllegalStateException {
// ensure database connection is available
if (!connectedToDatabase) {
throw new IllegalStateException("Not Connected to Database");
}
// obtain a value at specified ResultSet row and column
try {
resultSet.absolute(row + 1);
return resultSet.getObject(column + 1);
} // catch SQLExceptions and print error message
catch (SQLException sqlException) {
sqlException.printStackTrace();
}
// if problems, return empty string object
return "";
}
// set new database query string
public void setQuery(String query) throws SQLException, IllegalStateException {
// ensure database connection is available
if (!connectedToDatabase) {
throw new IllegalStateException("Not Connected to Database");
}
// specify query and execute it
resultSet = statement.executeQuery(query);
// obtain meta data for ResultSet
metaData = resultSet.getMetaData();
// determine number of rows in ResultSet
resultSet.last(); // move to last row
numberOfRows = resultSet.getRow(); // get row number
fireTableStructureChanged(); // notify JTable that model has changed
}
// close Statement and Connection
public void disconnectFromDatabase() {
// close Statement and Connection
try {
statement.close();
connection.close();
} // catch SQLExceptions and print error message
catch (SQLException sqlException) {
sqlException.printStackTrace();
} // update database connection status
finally {
connectedToDatabase = false;
}
}
} // end class ResultSetTableModel
PrintingBuses.java
package bus.ticket.reservation.system;
//import the packages for using the classes in them into the program
import javax.swing.*;
import java.awt.*;
import java.awt.print.PageFormat;
import java.awt.print.Printable;
import java.awt.print.PrinterException;
import java.sql.*;
import java.util.StringTokenizer;
import java.util.Vector;
public class PrintingBuses extends JDialog implements Printable {
/***************************************************************************
*** declaration of the private variables used in the program ***
***************************************************************************/
//for setting the connection and statement
private Connection connection = null;
private Statement statement = null;
private ResultSet resultset = null;
//for creating the text area
private JTextArea textArea = new JTextArea();
//for creating the vector to use it in the print
private Vector lines;
public static final int TAB_SIZE = 5;
//constructor of JLibrary
public PrintingBuses(String query, Connection url) {
//super("Printing Books", false, true, false, true);
//for getting the graphical user interface components display area
Container cp = getContentPane();
//for setting the font
textArea.setFont(new Font("Tahoma", Font.PLAIN, 9));
//for adding the textarea to the container
cp.add(textArea);
/***************************************************************
* for making the connection,creating the statement and update *
* the table in the database. After that,closing the statement *
* and connection. There is catch block SQLException for error *
***************************************************************/
try {
connection = url;
statement = connection.createStatement();
resultset = statement.executeQuery(query);
textArea.append("=============== Buses Information ===============\n\n");
while (resultset.next()) {
textArea.append("Bus Id: " + resultset.getString("BusID") + "\n" +
"Source(From): " + resultset.getString("source") + "\n" +
"Destination(To): " + resultset.getString("destination") + "\n" +
"Total Seats : " + resultset.getString("numberofseats") + "\n" +
"available: " + resultset.getString("available") + "\n\n");
}
textArea.append("=============== Bus Information ===============");
resultset.close();
statement.close();
connection.close();
}
catch (SQLException SQLe) {
System.out.println(SQLe.toString());
}
//for setting the visible to true
setVisible(true);
setBounds(200,100,400,400);
//to show the frame
pack();
}
public int print(Graphics pg, PageFormat pageFormat, int pageIndex) throws PrinterException {
pg.translate((int) pageFormat.getImageableX(), (int) pageFormat.getImageableY());
int wPage = (int) pageFormat.getImageableWidth();
int hPage = (int) pageFormat.getImageableHeight();
pg.setClip(0, 0, wPage, hPage);
pg.setColor(textArea.getBackground());
pg.fillRect(0, 0, wPage, hPage);
pg.setColor(textArea.getForeground());
Font font = textArea.getFont();
pg.setFont(font);
FontMetrics fm = pg.getFontMetrics();
int hLine = fm.getHeight();
if (lines == null)
lines = getLines(fm, wPage);
int numLines = lines.size();
int linesPerPage = Math.max(hPage / hLine, 1);
int numPages = (int) Math.ceil((double) numLines / (double) linesPerPage);
if (pageIndex >= numPages) {
lines = null;
return NO_SUCH_PAGE;
}
int x = 0;
int y = fm.getAscent();
int lineIndex = linesPerPage * pageIndex;
while (lineIndex < lines.size() && y < hPage) {
String str = (String) lines.get(lineIndex);
pg.drawString(str, x, y);
y += hLine;
lineIndex++;
}
return PAGE_EXISTS;
}
protected Vector getLines(FontMetrics fm, int wPage) {
Vector v = new Vector();
String text = textArea.getText();
String prevToken = "";
StringTokenizer st = new StringTokenizer(text, "\n\r", true);
while (st.hasMoreTokens()) {
String line = st.nextToken();
if (line.equals("\r"))
continue;
// StringTokenizer will ignore empty lines, so it's a bit tricky to get them...
if (line.equals("\n") && prevToken.equals("\n"))
v.add("");
prevToken = line;
if (line.equals("\n"))
continue;
StringTokenizer st2 = new StringTokenizer(line, " \t", true);
String line2 = "";
while (st2.hasMoreTokens()) {
String token = st2.nextToken();
if (token.equals("\t")) {
int numSpaces = TAB_SIZE - line2.length() % TAB_SIZE;
token = "";
for (int k = 0; k < numSpaces; k++)
token += " ";
}
int lineLength = fm.stringWidth(line2 + token);
if (lineLength > wPage && line2.length() > 0) {
v.add(line2);
line2 = token.trim();
continue;
}
line2 += token;
}
v.add(line2);
}
return v;
}
}
That's all. this is basic model of bus ticket reservation model.
you can add more components
> Add Routes of the bus
> user can all more than one seat
> Better UI interface
>User Cancel tickets
> User Can reserve tickets for future.
> add payment gateway(complex in swing)
Thank you for reading. If any query comment below.