Object detection in machine learning
Object detection is widely used in many fields. For example, in self-driving technology, we need to plan routes by identifying the locations of vehicles, pedestrians, roads, and obstacles in the captured video image. Robots often perform this type of task to detect targets of interest.
First import Libraries
First import all the libraries related to object detection
#import libraries
%matplotlib inline
import d2l
from mxnet import image, npx
npx.set_np()
After this load the data
#load dataset
d2l.set_figsize((3.5, 2.5))
img = image.imread('imagepath/catdog.jpg').asnumpy()
d2l.plt.imshow(img);
Output:
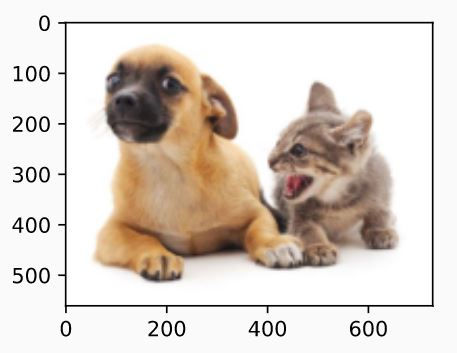
Bounding Box
This is one object detection technology that is used to detect the object by x and y-axis.
# bbox is the abbreviation for bounding box
dog_bbox, cat_bbox = [60, 45, 378, 516], [400, 112, 655, 493]
We can draw the bounding box in the image to check if it is accurate.
# Saved in the d2l package for later use
def bbox_to_rect(bbox, color):
"""Convert bounding box to matplotlib format."""
return d2l.plt.Rectangle(
xy=(bbox[0], bbox[1]), width=bbox[2]-bbox[0], height=bbox[3]-bbox[1],
fill=False, edgecolor=color, linewidth=2)
After loading the bounding box on the image, we can see that the main outline of the target is basically inside the box.
#show image in frame
fig = d2l.plt.imshow(img)
fig.axes.add_patch(bbox_to_rect(dog_bbox, 'blue'))
fig.axes.add_patch(bbox_to_rect(cat_bbox, 'red'));
Output:
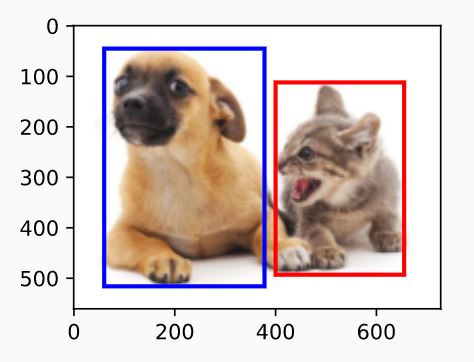
Get your project or assignment completed by Deep learning expert and experienced developers and researchers.
OR
If you have project files, You can send at codersarts@gmail.com directly