System Call - request to the kernel layer to perform a task that must be accomplished by the operating system.
Some categories of system calls:
I/O
creating files and directories
reading/writing files
positioning in files
reading directories
Process Control
creating processes and threads
terminating processes and threads
obtaining process ID
Communication
manipulating pipes
manipulating sockets
managing shared memory
Linux layers diagram
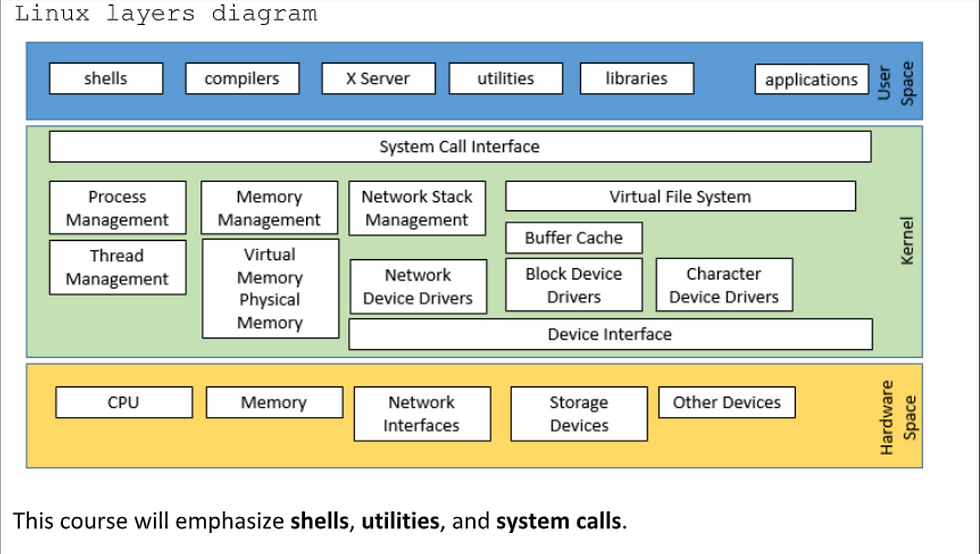
standard stream I/O
You used standard stream I/O functions (scanf, fgets, printf, fopen, fclose). These functions
were intended to make I/O easier than the low level functions by handling:
data conversions (e.g., printf format codes convert from C data types to character output)
buffer input data, allowing multiple calls to access data that is physically read with a single input request
buffer output data, allowing multiple calls to print data although a physical write is done for larger quantities
efficiently read/write data
Under the covers of those functions are low level I/O operations which do the real I/O work.
We will discuss the internal representations of files and directories, standard I/O functions, file descriptors, and then discuss the low level I/O operations.
==========. ================= ================================================================
Function Category Purpose
========== ================== ===============================================================
scanf std i/o (stream). stream input using format codes.
fscanf std i/o (stream). Note that sscanf gets its data from a string variable.
sscanf std i/o (stream)
gets std i/o (stream) stream input of text lines
fgets. std i/o (stream). stream input of text lines
printf std i/o (stream) stream output using format codes
fprintf std i/o (stream) stream output using format codes
getc std i/o (stream) get next char from a stream
fgetc std i/o (stream) get next char from a stream
putc. std i/o (stream) put a char to a stream
fputc std i/o (stream) put a char to a stream
fopen std i/o open a file for buffered i/o
fclose std i/o close a file opened by fopen
fread binary read binary input of one or more logical records
fwrite binary write binary output of one or more logical records
fseek binary position changes file position to a location relative to a
number of bytes from the beginning of the file
open unix low level i/o. opens a file
close unix low level i/o closes a file
read unix low level i/o reads a specified number of bytes at the current position
write unix low level i/o writes a specified number of bytes at the current position
lseek unix low level i/o similar to fseek
stat unix low returns the stat structure for a file which includes
fstat level i/o inodeNr, file type, file mode, number of links, size, etc.
opendir unix low level i/o. opens the specified directory for reading
readdir unix low level i/o reads the next directory entry.
closedir unix low level i/o. closes a directory