Advanced Data Processing: Integrating Apache Kafka with Spark Streaming
- Codersarts
- Oct 22, 2024
- 5 min read
Are you looking to elevate your data processing skills to handle real-time, high-throughput data streams? Integrating Apache Kafka with Apache Spark Streaming unlocks powerful capabilities for advanced data processing and real-time analytics.
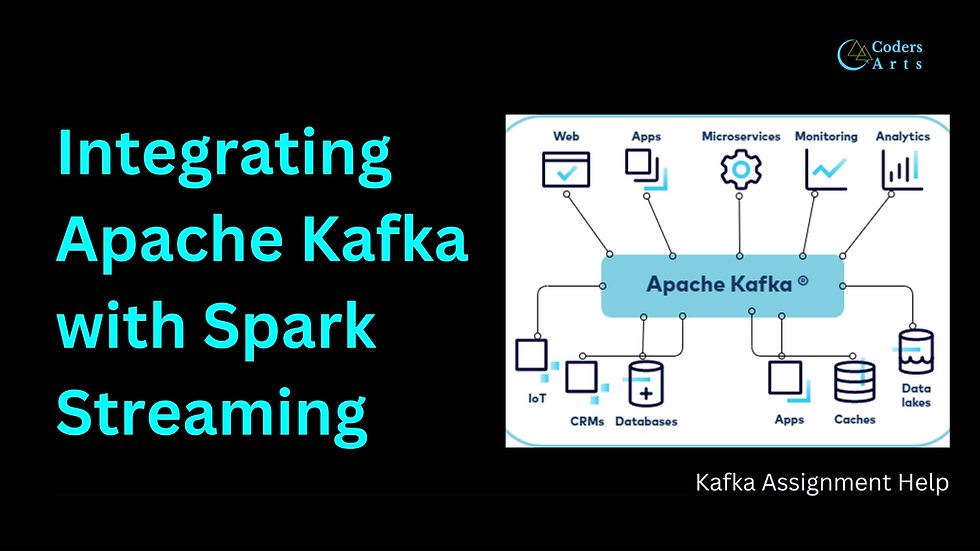
This comprehensive guide will walk you through an assignment focused on making machine learning predictions on streaming data, using the synergy of Kafka and Spark.
Introduction
By integrating Kafka with Spark Streaming, you can build robust applications capable of ingesting, processing, and analyzing data in real time, even applying machine learning models to generate insights on the fly.
Assignment Objectives
Goals
Data Integration: Set up Kafka and Spark to work together seamlessly.
Data Processing: Ingest and process streaming data representing user activities.
Machine Learning Application: Apply a pre-trained machine learning model to the streaming data for real-time predictions.
Expected Outcomes
Gain hands-on experience in integrating Kafka with Spark Streaming.
Understand how to consume and process data streams in real time.
Learn how to apply machine learning models to streaming data.
Enhance your skills in building scalable, real-time data processing pipelines.
Step-by-Step Guide
1. Environment Setup
Prerequisites
Java Development Kit (JDK): Java 8 or later.
Scala: Recommended for Spark (optional if using PySpark).
Python: For PySpark (if preferred).
Apache Kafka: Latest stable version.
Apache Spark: Version compatible with your Kafka installation.
Installing Apache Kafka
Download Kafka: Get the latest version from the official website.
Extract Files: Unzip the downloaded archive to your desired directory.
Start ZooKeeper (Kafka's coordination service):
bin/zookeeper-server-start.sh config/zookeeper.properties
Start Kafka Broker:
bin/kafka-server-start.sh config/server.properties
Installing Apache Spark
Download Spark: Obtain the latest version from the official website.
Extract Files: Unzip the archive to your preferred location.
Set Environment Variables:
SPARK_HOME: Path to your Spark directory.
PATH: Add $SPARK_HOME/bin to your system path.
Configuring Dependencies
Ensure that Kafka and Spark are compatible. Check the documentation for any specific version compatibility issues.
Include the necessary dependencies for Kafka and Spark integration in your build tool (e.g., Maven, SBT, or Gradle).
Example (using Maven for Scala project):
<dependencies>
<!-- Spark Streaming -->
<dependency>
<groupId>org.apache.spark</groupId>
<artifactId>spark-streaming_2.12</artifactId>
<version>3.1.2</version>
</dependency>
<!-- Spark Streaming Kafka Integration -->
<dependency>
<groupId>org.apache.spark</groupId>
<artifactId>spark-streaming-kafka-0-10_2.12</artifactId>
<version>3.1.2</version>
</dependency>
<!-- Kafka Clients -->
<dependency>
<groupId>org.apache.kafka</groupId>
<artifactId>kafka-clients</artifactId>
<version>2.8.0</version>
</dependency>
</dependencies>
2. Simulating Streaming Data
Creating a Kafka Producer for User Activity Data
We will simulate user activities (e.g., website clicks, page views) and send this data to a Kafka topic.
Create Kafka Topic
bin/kafka-topics.sh --create --topic user-activities --bootstrap-server localhost:9092 --replication-factor 1 --partitions 1
Producer Implementation in Python
from kafka import KafkaProducer
import json
import time
import random
producer = KafkaProducer(
bootstrap_servers=['localhost:9092'],
value_serializer=lambda v: json.dumps(v).encode('utf-8')
)
user_ids = [f"user_{i}" for i in range(1, 101)]
activities = ['click', 'view', 'purchase', 'add_to_cart']
while True:
data = {
'user_id': random.choice(user_ids),
'activity': random.choice(activities),
'timestamp': int(time.time() * 1000)
}
producer.send('user-activities', data)
print(f"Sent: {data}")
time.sleep(0.5)
Explanation
Data Fields:
user_id: Simulated user identifier.
activity: Type of user activity.
timestamp: Event time in milliseconds.
Serialization: Data is serialized to JSON format before sending.
3. Developing the Spark Streaming Application
Setting Up Spark Streaming to Consume Kafka Data
Initializing Spark Streaming Context
from pyspark.sql import SparkSession
from pyspark.streaming import StreamingContext
from pyspark.streaming.kafka import KafkaUtils
spark = SparkSession.builder \
.appName("KafkaSparkStreaming") \
.getOrCreate()
sc = spark.sparkContext
ssc = StreamingContext(sc, batchDuration=5)
Consuming Data from Kafka
kafka_params = {
"metadata.broker.list": "localhost:9092"
}
kafka_stream = KafkaUtils.createDirectStream(
ssc,
topics=['user-activities'],
kafkaParams=kafka_params
)
Data Deserialization and Schema Definition
import json
from pyspark.sql.types import StructType, StructField, StringType, LongType
def parse_json(record):
data = json.loads(record[1])
return (data['user_id'], data['activity'], data['timestamp'])
schema = StructType([
StructField("user_id", StringType(), True),
StructField("activity", StringType(), True),
StructField("timestamp", LongType(), True)
])
parsed_stream = kafka_stream.map(parse_json)
4. Implementing Machine Learning
Using a Pre-Trained Model
For this assignment, we'll use a pre-trained Logistic Regression model to predict the likelihood of a user making a purchase based on their activity.
Loading the Pre-Trained Model
from pyspark.ml.classification import LogisticRegressionMode
model = LogisticRegressionModel.load("path/to/pretrained/model")
Applying the Model to Streaming Data
from pyspark.sql import Row
def process_rdd(time, rdd):
if not rdd.isEmpty():
df = spark.createDataFrame(rdd, schema)
# Feature Engineering
df_features = feature_engineering(df)
# Predictions
predictions = model.transform(df_features)
# Output Results
predictions.select("user_id", "activity", "probability", "prediction").show()
parsed_stream.foreachRDD(process_rdd)
Feature Engineering Function
def feature_engineering(df):
# Example: Convert categorical 'activity' to numerical features
from pyspark.ml.feature import StringIndexer
indexer = StringIndexer(inputCol="activity", outputCol="activityIndex")
df = indexer.fit(df).transform(df)
return df
5. Outputting Predictions
Writing Predictions Back to Kafka
def send_to_kafka(row):
producer = KafkaProducer(bootstrap_servers=['localhost:9092'])
data = {
'user_id': row['user_id'],
'prediction': row['prediction'],
'probability': row['probability'],
'timestamp': row['timestamp']
}
producer.send('predictions', json.dumps(data).encode('utf-8'))
predictions.foreach(send_to_kafka)
Storing in a Database (e.g., Cassandra)
Alternatively, you can store the predictions in a NoSQL database like Cassandra.
def save_to_cassandra(df):
df.write \
.format("org.apache.spark.sql.cassandra") \
.options(table="user_predictions", keyspace="streaming_data") \
.mode("append") \
.save()
6. Optimization Techniques
Performance Tuning for Spark Streaming
Batch Intervals: Adjust batchDuration to balance between latency and throughput.
Resource Allocation: Allocate sufficient executor memory and cores.
Use spark-submit options like --executor-memory and --total-executor-cores.
Configuration Settings
Backpressure: Enable Spark Streaming backpressure mechanism to adjust processing rates.
spark.conf.set("spark.streaming.backpressure.enabled", True)
Checkpointing: Use checkpointing to handle stateful transformations and recover from failures.
ssc.checkpoint("path/to/checkpoint/dir")
7. Error Handling
Handling Exceptions in Data Processing
Implement try-except blocks in your processing functions.
def process_rdd(time, rdd):
try:
if not rdd.isEmpty():
# Processing logic
pass
except Exception as e:
print(f"Error processing RDD at time {time}: {e}")
Robust Error Handling Mechanisms
Logging: Use a logging framework to record errors and system states.
import logging
logging.basicConfig(level=logging.ERROR)
Graceful Degradation: Implement fallback mechanisms for when components fail.
Data Validation: Validate incoming data to handle malformed records.
Challenges and Expert Solutions
Latency Issues
Challenge: High latency in processing streaming data.
Solution:
Optimize batch intervals.
Reduce processing time by simplifying computations.
Scale resources horizontally.
Data Consistency
Challenge: Ensuring data consistency between Kafka and Spark.
Solution:
Use exactly-once semantics provided by Kafka and Spark.
Enable Kafka's enable.auto.commit cautiously.
Model Scalability
Challenge: Machine learning model not scaling with data volume.
Solution:
Use models that support distributed computation.
Consider model compression techniques.
Expert Recommendations
Monitoring: Implement monitoring tools like Spark UI and Kafka Manager.
Security: Secure data pipelines using SSL encryption and authentication mechanisms.
Documentation: Maintain clear documentation for configurations and codebases.
How Codersarts Can Help
At Codersarts, we offer expert assistance to help you master the integration of Apache Kafka with Spark Streaming.
Our Services
Integration Support: Guidance on setting up and configuring Kafka-Spark integration.
Machine Learning Assistance: Help with implementing and optimizing machine learning models in Spark.
Code Review: Professional code review to enhance efficiency and reliability.
Troubleshooting: Assistance in resolving technical challenges and performance issues.
Customized Training: One-on-one tutoring sessions tailored to your learning needs.
Why Choose Codersarts
Experienced Professionals: Work with experts who have hands-on experience in real-time data processing.
Personalized Solutions: Receive assistance tailored to your specific project requirements.
24/7 Availability: Our team is available around the clock to support you.
Conclusion
Integrating Apache Kafka with Spark Streaming empowers you to build advanced data processing pipelines capable of handling real-time, high-throughput data. By applying machine learning models to streaming data, you can generate immediate insights and predictions, a critical capability in sectors like finance, e-commerce, and IoT.
Mastering these technologies not only enhances your technical skillset but also opens doors to exciting career opportunities in data engineering and analytics.
Ready to take your data processing skills to the next level? Whether you're facing challenges with integration, data processing, or machine learning implementation, Codersarts is here to help you succeed.
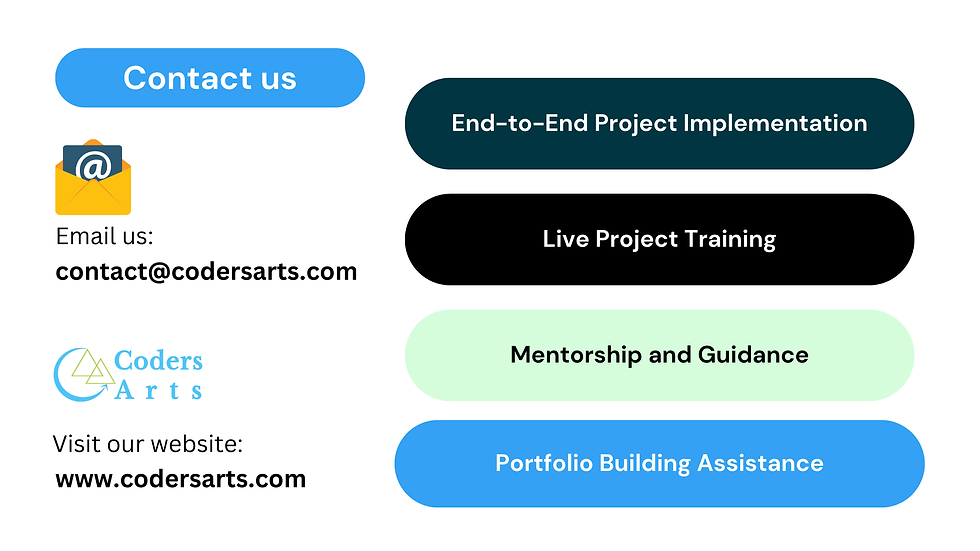
Keywords: Kafka and Spark integration, streaming data processing, machine learning with Spark, Apache Kafka assignments, Spark Streaming help
Comments