Spring Boot is built on top of the Spring Framework, which uses the Model-View-Controller (MVC) architecture to structure web applications. In MVC, the application is divided into three interconnected components:
Model: This component represents the business data and logic of the application. It includes classes and objects that encapsulate the data and methods to manipulate it.
View: This component is responsible for rendering the user interface of the application. It includes HTML, CSS, and JavaScript files that define the layout and appearance of the web pages.
Controller: This component is the intermediate layer between the Model and View components. It handles user requests, updates the Model based on user input, and selects the appropriate View to render the response.
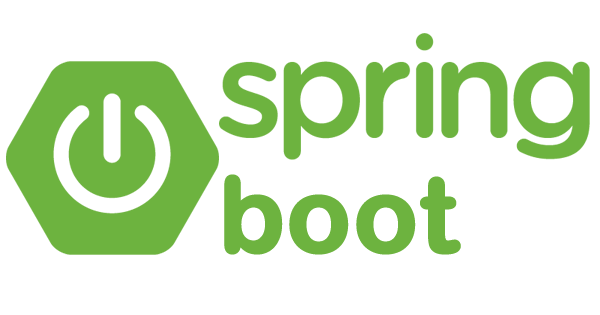
In Spring Boot, the MVC architecture is implemented using the Spring MVC module. The Spring MVC module provides a number of features to support the development of web applications, including:
Request mapping: Allows developers to map HTTP requests to methods in the Controller.
Model and View support: Provides classes to represent Model and View components and manage their interactions.
Data binding: Automatically maps request parameters to Model properties and vice versa.
Form handling: Provides support for rendering and processing HTML forms.
Validation: Allows developers to validate user input and display error messages.
File uploading: Provides support for uploading and processing files.
Overall, the Spring Boot MVC architecture is designed to provide a flexible and scalable approach to building web applications, making it a popular choice for developers.
Here's an example of a simple RESTful API implemented using Spring Boot:
Create a new Spring Boot project using your preferred IDE.
Add the following dependencies to your pom.xml file:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
</dependencies>
3. Create a new Java class called Employee to represent our resource:
@Data
@AllArgsConstructor
@NoArgsConstructor
@Entity
@Table(name = "employee")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String department;
private double salary;
}
4. Create a new interface called EmployeeRepository that extends JpaRepository:
public interface EmployeeRepository extends JpaRepository<Employee, Long> {
}
5. Create a new REST controller called EmployeeController:
@RestController
@RequestMapping("/api/v1")
public class EmployeeController {
@Autowired
private EmployeeRepository employeeRepository;
@GetMapping("/employees")
public List<Employee> getAllEmployees() {
return employeeRepository.findAll();
}
@GetMapping("/employees/{id}")
public ResponseEntity<Employee> getEmployeeById(@PathVariable(value = "id") Long employeeId)
throws ResourceNotFoundException {
Employee employee = employeeRepository.findById(employeeId)
.orElseThrow(() -> new ResourceNotFoundException("Employee not found for this id :: " + employeeId));
return ResponseEntity.ok().body(employee);
}
@PostMapping("/employees")
public Employee createEmployee(@Valid @RequestBody Employee employee) {
return employeeRepository.save(employee);
}
@PutMapping("/employees/{id}")
public ResponseEntity<Employee> updateEmployee(@PathVariable(value = "id") Long employeeId,
@Valid @RequestBody Employee employeeDetails) throws ResourceNotFoundException {
Employee employee = employeeRepository.findById(employeeId)
.orElseThrow(() -> new ResourceNotFoundException("Employee not found for this id :: " + employeeId));
employee.setName(employeeDetails.getName());
employee.setDepartment(employeeDetails.getDepartment());
employee.setSalary(employeeDetails.getSalary());
final Employee updatedEmployee = employeeRepository.save(employee);
return ResponseEntity.ok(updatedEmployee);
}
@DeleteMapping("/employees/{id}")
public Map<String, Boolean> deleteEmployee(@PathVariable(value = "id") Long employeeId)
throws ResourceNotFoundException {
Employee employee = employeeRepository.findById(employeeId)
.orElseThrow(() -> new ResourceNotFoundException("Employee not found for this id :: " + employeeId));
employeeRepository.delete(employee);
Map<String, Boolean> response = new HashMap<>();
response.put("deleted", Boolean.TRUE);
return response;
}
}
6. Run the application and use a tool like Postman to test the API endpoints:
GET http://localhost:8080/api/v1/employees
GET http://localhost:8080/api/v1/employees/{id}
POST http://localhost:8080/api/v1/employees
PUT http://localhost:8080/api/v1/employees/{id}
DELETE http://localhost:8080/api/v1/employees/{id}
Need help in your spring boot project work??
For any Spring boot project assistance or job support connect with codersarts. At codersarts you get project help and job support revolving around technologies like Java, Spring Boot, Angular, React, ML and so on. Take me to codersarts

Comments