Ensemble Selection: Techniques and Implementation in Python
- Pushkar Nandgaonkar
- Feb 25, 2023
- 3 min read
In the field of machine learning, the goal is to create models that can make accurate predictions on new data. Ensemble learning is a powerful technique that combines multiple models to achieve better predictive performance. Ensemble selection is a subfield of ensemble learning that focuses on selecting a subset of models to use in the final ensemble. In this article, we will provide an overview of ensemble selection, discuss different techniques for selecting models, and show how to implement ensemble selection in Python.
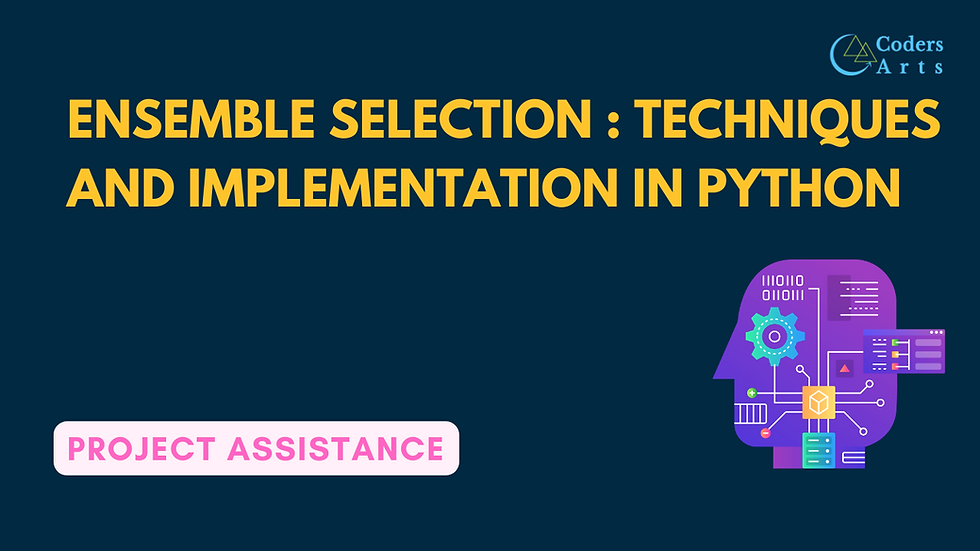
Overview of Ensemble Selection
Ensemble selection is the process of selecting a subset of models from a larger pool of models to use in the final ensemble. The goal of ensemble selection is to find the best combination of models that maximizes the predictive performance of the ensemble. Ensemble selection is particularly useful when there are many models available, and it is not practical to use all of them in the final ensemble.
There are two main approaches to ensemble selection: random selection and deterministic selection. Random selection involves randomly selecting a subset of models from the larger pool of models. Deterministic selection involves using a selection algorithm to choose the best subset of models based on some criterion.
Techniques for Ensemble Selection
There are several techniques for ensemble selection, including random subset selection, greedy selection, and genetic algorithms.
Random Subset Selection
Random subset selection is the simplest technique for ensemble selection. It involves randomly selecting a subset of models from the larger pool of models. The size of the subset can be fixed or can vary depending on the size of the pool of models. Random subset selection is easy to implement and can be used as a baseline method for comparing the performance of other ensemble selection techniques.
Greedy Selection
Greedy selection is a technique that involves iteratively adding models to the ensemble based on their individual performance. The process starts with an empty ensemble and iteratively adds the model that improves the performance of the ensemble the most. The process continues until a predetermined stopping criterion is met. Greedy selection can be used with different performance measures, such as accuracy, AUC, or F1 score.
Genetic Algorithms
Genetic algorithms are a class of optimization algorithms inspired by the process of natural selection. In the context of ensemble selection, genetic algorithms involve treating each model as an individual in a population and using a fitness function to evaluate the performance of each individual. The genetic algorithm then uses selection, crossover, and mutation operators to generate a new population of models. The process continues until a predetermined stopping criterion is met.
How to Implement Ensemble Selection in Python Implementing ensemble selection in Python can be done using various libraries and frameworks. One popular library is mlxtend, which provides a range of tools for machine learning and data analysis.
Here's an example of how to perform ensemble selection using mlxtend:
from mlxtend.classifier import EnsembleSelectionClassifier
from sklearn.tree import DecisionTreeClassifier
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
# Load the dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Define the base models
model_1 = DecisionTreeClassifier()
model_2 = RandomForestClassifier(n_estimators=10)
# Define the ensemble selection classifier
ensemble = EnsembleSelectionClassifier(classifiers=[model_1, model_2], k_best=2, refit=True)
# Train the ensemble classifier
ensemble.fit(X_train, y_train)
# Evaluate the ensemble classifier on the testing set
score = ensemble.score(X_test, y_test)
print("Ensemble selection score:", score)
In this example, we load the iris dataset and split it into training and testing sets. We then define two base models, a decision tree classifier and a random forest classifier. We create an EnsembleSelectionClassifier object with the two base models, and set k_best to 2, which means that we want to select the top 2 models from the pool of candidates. We fit the ensemble classifier on the training set and evaluate its performance on the testing set.
Conclusion
Conclusion Ensemble learning is a powerful technique for improving the accuracy and robustness of machine learning models. By combining multiple models, ensemble learning can reduce overfitting, increase predictive power, and improve generalization to new data. In this article, we covered various ensemble learning methods, including bagging, boosting, stacking, and ensemble selection, along with their advantages, disadvantages, and implementation details in Python. With the right combination of ensemble methods and model selection techniques, you can create highly accurate and reliable machine learning models for a wide range of applications.

Kommentare