Exception Handling, try, catch, throw, and finally
- Abhay Tiwari
- Dec 14, 2022
- 2 min read
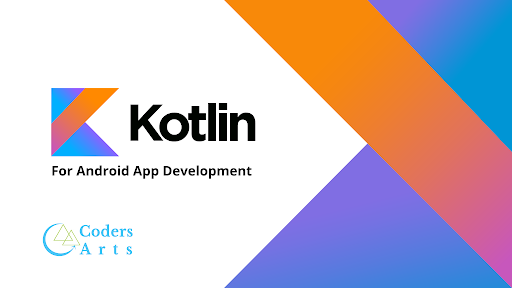
Exception handling is a technique, using which we can handle errors and prevent run time crashes that can stop our program.
There are two types of Exceptions –
Checked Exception – Exceptions that are typically set on methods and checked at the compile time, for example, IOException, FileNotFoundException, etc
Unchecked Exception – Exceptions that are generally due to logical errors and checked at the run time, for example, NullPointerException, ArrayIndexOutOfBoundException, etc
Kotlin program of throwing an arithmetic exception –
fun main(args : Array<String>){
var num = 10 / 0 // throws exception
println(num)
}
Output:
Exception in thread "main" java.lang.ArithmeticException: / by zero
Kotlin try-catch block –
This block must be written within the main or other methods. Try block should be followed by either catch block or finally block or both.
The syntax for try-catch block –
try {
// code that can throw exception
} catch(e: ExceptionName) {
// catch the exception and handle it
}
Kotlin program of arithmetic exception handling using try-catch block –
import kotlin.ArithmeticException
fun main(args : Array<String>){
try{
var num = 10 / 0
}
catch(e: ArithmeticException){
// caught and handles it
println("Divide by zero not allowed")
}
}
Output:
Divide by zero not allowed
Kotlin program of using finally block with try-catch block-
fun main (args: Array<String>){
try {
var int = 10 / 0
println(int)
} catch (e: ArithmeticException) {
println(e)
} finally {
println("This block always executes")
}
}
Hope you understand the exception handling and syntax of kotlin in the next blog we are going to learn collections in kotlin.
Thank you
The journey of solving bug and completing project on time in Kotlin can be challenging and lonely. If you need help regarding other sides to Kotlin, we’re here for you!
Comments