Finding the Longest Common Prefix: A Solution in Java
- Swapnil V
- Jun 28, 2023
- 2 min read
Introduction
In many programming situations, we are often faced with the need to find the longest common prefix between strings. Whether analyzing DNA sequences, finding similar filenames, or dealing with data in different formats, the longest common prefix problem proves to be a useful challenge. We explore a Java solution to this problem, discuss the algorithm, and provide a step-by-step implementation. The longest common prefix problem involves finding the longest string common to a given set of strings. This is a classic string-matching problem that can have a variety of real-world applications. The task is to identify the longest prefix shared by all the strings in the set.
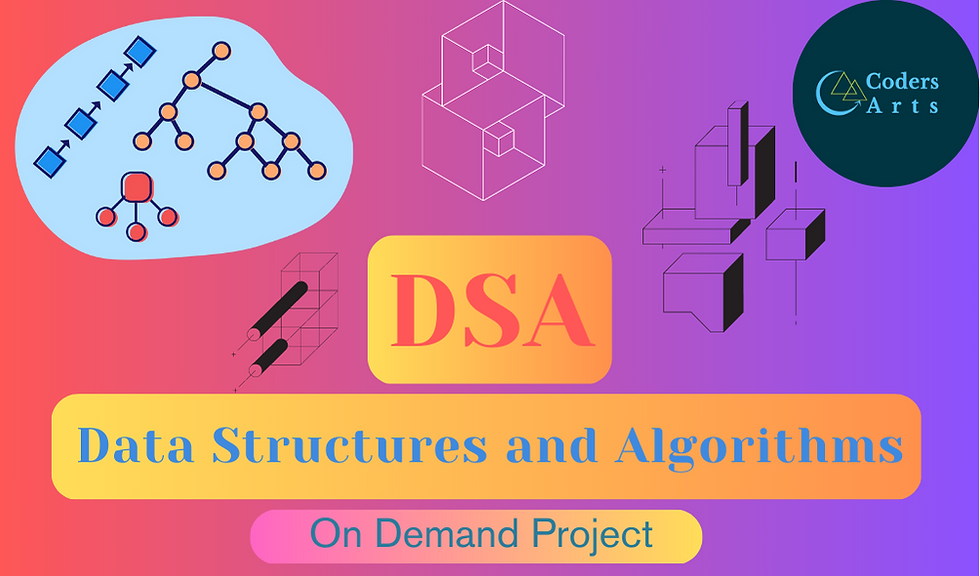
Algorithm:
To solve the longest common prefix problem, we can use a simple algorithm that iterates over strings and gradually save the common prefix.
Sort the array of strings order using `Arrays. sort`.
Assign the first string in the sorted array `strs[0]` to the variable `str1`.
Assign the last string in the sorted array `strs[strs.length-1]` to the variable `str2`.
Initialize a variable `index` to 0.
Enter the while loop that continues as long as the index is within the limits of both `str1` and `str2`.
Inside the loop, compare the characters at the current index of str1 and str2. If they are equal, increase the index value.
If the characters are not equal, break out of the loop.
After the loop finishes, return the substring of str2 from index 0 to index as the longest common prefix.
Code:
class Main {
public String longestCommonPrefix(String[] strs) {
// To sort the array
Arrays.sort(strs);
// After sorting first string
String str1=strs[0];
// After sorting last string
String str2=strs[strs.length-1];
//To iterate through string
int index=0;
while(index<str1.length() && index<str2.length()){
// To check whether characters are same or not
if(str1.charAt(index)==str2.charAt(index)){
index++;
}else{
break;
}
}
// Return the common substring starting from 0.
return str2.substring(0,index);
}
}
At codersArts:
Expert Assistance: Our team consists of highly skilled Java, and Spring Boot developers who have extensive experience in the field. They are proficient in the latest tools, frameworks, and technologies, ensuring top-notch solutions for your assignments, Projects.
Timely Delivery: We understand the importance of deadlines. Our experts work diligently to deliver your completed assignments, Projects on time, allowing you to submit your work without any worries.
Customized Solutions: We believe in providing personalized solutions that meet your specific requirements. Our experts carefully analyze your assignment or Project and provide tailored solutions to ensure your success.
Affordable Pricing: We offer competitive and affordable pricing to make our services accessible to students, Developers. We understand the financial constraints that students, Developers often face, and our pricing is designed to accommodate their needs.
24/7 Support: Our customer care team is available round the clock to assist you with any queries or concerns you may have. You can reach us via phone, email, or live chat.
Contact CodersArts today for reliable and professional Spring Boot, Spring Security, and Java help. Let our experts take your Java programming skills to the next level!

Comments