Flask for Beginners: Build Your First RESTful API with Flask-RESTful
- Pranav S
- Mar 13, 2023
- 2 min read
Updated: Apr 27, 2023
In this tutorial, we'll be building a simple RESTful API using Flask-RESTful. Flask-RESTful is an extension to Flask that makes it easy to build RESTful APIs. We'll be building a basic API that allows users to create and view blog posts.
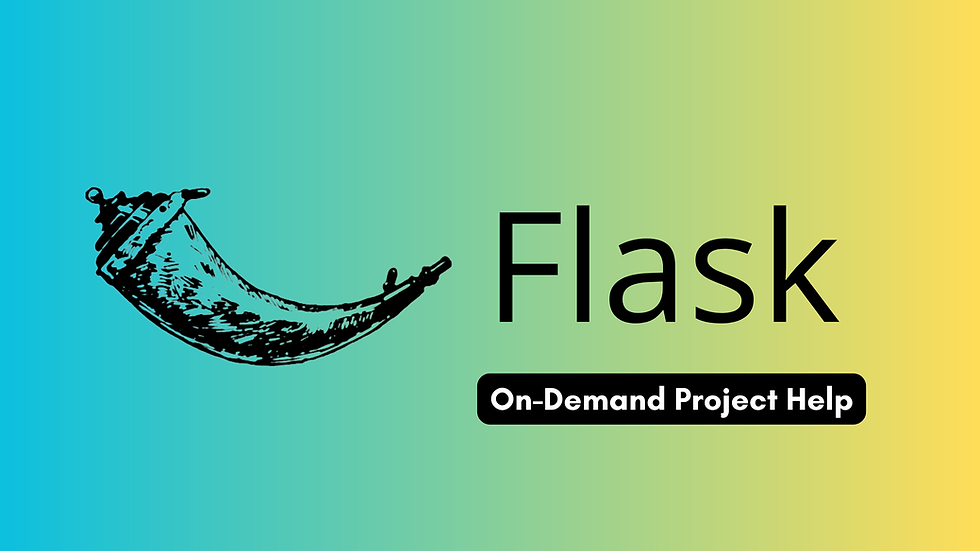
Before we get started, make sure you have Flask-RESTful installed. You can install it using pip:
pip install flask-restful
Once you have Flask-RESTful installed, you can create a new Flask app and add Flask-RESTful to it:
from flask import Flask
from flask_restful import Api
app = Flask(__name__)
api = Api(app)
Defining Resources
In Flask-RESTful, resources are objects that represent a set of actions that can be performed on a specific endpoint. For our blog API, we'll need a resource for creating blog posts and a resource for viewing all blog posts.
from flask_restful import Resource
class BlogPost(Resource):
def post(self):
# create a new blog post
pass
class BlogPostList(Resource):
def get(self):
# get all blog posts
pass
Each resource is a class that inherits from the Resource class provided by Flask-RESTful. We define a post method for the BlogPost resource and a get method for the BlogPostList resource. We'll fill in the logic for these methods later.
Registering Resources
Once we've defined our resources, we need to register them with our Flask app using the add_resource method provided by Flask-RESTful.
api.add_resource(BlogPost, '/posts')
api.add_resource(BlogPostList,'/posts')
We've registered our BlogPost resource at the /posts endpoint, and our BlogPostList resource at the same endpoint.
Implementing Resource Methods
Now that we've defined and registered our resources, we need to implement the methods that handle the logic for creating and viewing blog posts.
from flask import request
class BlogPost(Resource):
def post(self):
# create a new blog post
title = request.json.get('title')
content = request.json.get('content')
# save the blog post to the database
return{'message':'Blog post created'},201
In the post method of the BlogPost resource, we retrieve the title and content of the blog post from the request JSON, save the blog post to the database, and return a response with a message indicating that the blog post was created.
Define the get method in the BlogPostList, we have to retrieve all blog posts from the database and return them in a JSON response. similar to what we did with the BlogPost.
That's it! We've built a simple RESTful API using Flask-RESTful
To get the full implementation or need any assistance using Flask and RESTful api's feel free to contact us at contact@codersarts.com

Comments