Authentication and authorization are important parts of building secure web applications. Authentication is the process of verifying the identity of a user, while authorization is the process of determining what resources a user has access to. Flask provides several extensions that make it easy to add authentication and authorization to your application.
In this post, we'll cover how to use Flask-Login and Flask-Principal to add user authentication and role-based access control to your app.
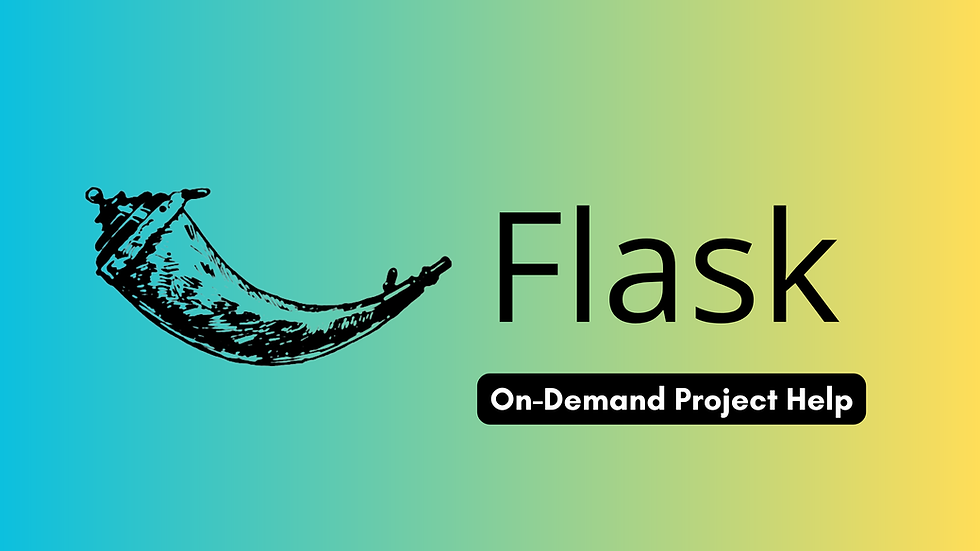
Flask-Login
Flask-Login is a popular Flask extension that provides user authentication functionality out of the box. It handles the process of logging users in and out, managing user sessions, and protecting views that require authentication.
To use Flask-Login, you'll need to install it using pip:
pip install flask-login
Next, you'll need to create a user model for your application. This model should represent a user and include fields like username, email, and password. Here's an example user model:
from flask_login import UserMixin
from werkzeug.security import generate_password_hash, check_password_hash
class User(UserMixin):
def __init__(self, id, username, email, password):
self.id = id
self.username = username
self.email = email
self.password_hash = generate_password_hash(password)
def check_password(self, password):
return check_password_hash(self.password_hash, password)
In this example, we're using the UserMixin class provided by Flask-Login, which includes several helper methods for working with user models. We're also using the generate_password_hash and check_password_hash functions from the Werkzeug library to securely store and check user passwords.
Once you've defined your user model, you'll need to create a login view that handles the process of logging users in. Do refer to previous blogs for creating this here is a general instructions for writing this login view:
Import the necessary modules and classes
Define the login route and methods
Check if the request method is POST and Get the username and password from the login form
Query the User table for the user with the given username
Check if the user exists and if the password is correct
If the login is successful, log the user in and redirect them to the next page or the homepage
If the login is unsuccessful, flash an error message use inbuilt flash function from Flask library
Render the login page template
Similarly create a logout view that handles the process of logging users out.
Flask-Principal
Flask-Principal is a Flask extension that provides role-based access control functionality. It allows you to define roles for your application and restrict access to views based on those roles.
To use Flask-Principal, you'll need to install it using pip:
pip install flask-principal
Next, you'll need to define the roles for your application. Here's an example:
from flask_principal import RoleNeed, UserNeed
admin_role = RoleNeed('admin')
moderator_role = RoleNeed('moderator')
user_role = RoleNeed('user')
In this example, we're creating three roles: admin, moderator, and user. Each role is represented by a RoleNeed object.
Next, you'll need to associate each role with the appropriate users. You can do this by defining a identity_loaded callback function that adds the user's roles to their identity. Here's an example:
from flask_principal import identity_loaded, Identity
@identity_loaded.connect_via(app)
def on_identity_loaded(sender, identity):
# Set the identity user object
user = current_user
if hasattr(user, 'id'):
identity.provides.add(UserNeed(user.id))
# Add the user's roles to the identity
if hasattr(user, 'roles'):
for role in user.roles:
identity.provides.add(RoleNeed(role.name))
In this example, we're using the identity_loaded signal provided by Flask-Principal to add the user's roles to their identity. We're also adding a UserNeed to the identity, which represents the user's unique identifier.
Finally, you'll need to use the RoleNeed objects to restrict access to views that require certain roles. You can do this by using the roles_required decorator provided by Flask-Principal. Here's an example:
from flask_principal import Permission, RoleNeed, identity_loaded, UserNeed
admin_permission = Permission(RoleNeed('admin'))
@app.route('/admin')
@admin_permission.require()
def admin():
return 'Admin Page'
In this example, we're using the Permission object provided by Flask-Principal to define a permission that requires the admin role. We're also using the roles_required decorator to restrict access to the /admin view.
Adding authentication and authorization to your Flask application is an important step towards building a secure and scalable web application. With Flask-Login and Flask-Principal, it's easy to add user authentication and role-based access control to your app. I hope this post has been helpful in getting you started with these extensions
If you need exact implementation or need help in using Flask for your project or assignment just drop a mail to contact@codersarts.com

Comments