Are you interested in building web applications but don't know where to start? Flask, a micro web framework written in Python, is a great place to begin. In this beginner-friendly guide, we'll show you how to create a simple "Hello World" of the web app using Flask in just a few minutes.
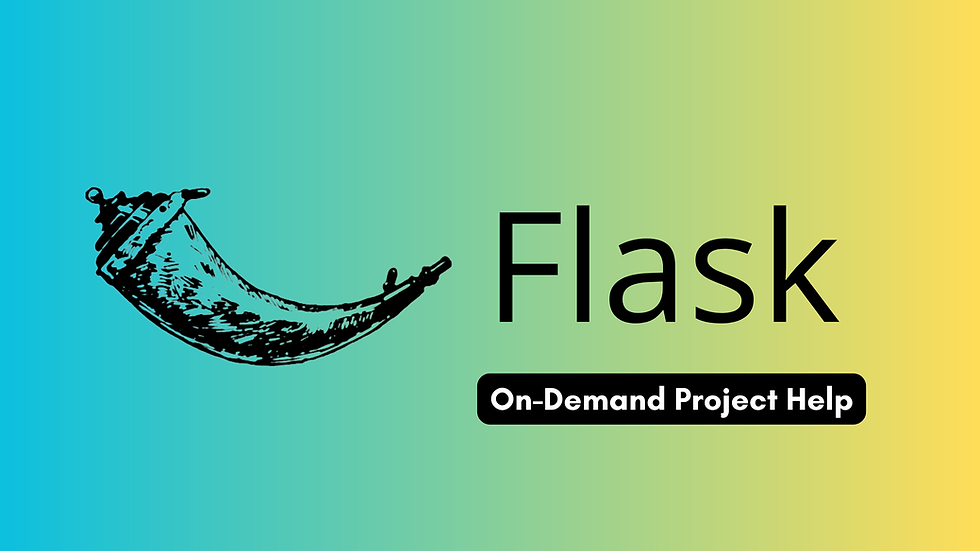
Setting up a Flask project
Before we begin, let's make sure we have everything we need. First, create a new directory for your project and navigate into it using the command prompt (Windows)/ terminal (linux). Make sure you have a proper installation of python. You can check by typing the following commands in cmd/ terminal
python --version
or
py --version
It should display what version of python you have installed. So In python it is always advisable to create virtual environments for each project separately in order to avoid clashes of library or version of library as it varies from one project to other. For this project we will create a virtual environment using python's inbuilt library "venv". (Note if python does not work for you use py instead).
python -m venv flask_venv
Here we have created a virtual environment name flask_env where we can install all the libraries needed for flask app. To activate the virtual environment you can use the following commands. ( Note I am inside the folder I want to create the venv "D:\flask_tutorials" is the name of my folder)
D:\flask_tutorials>flask_venv\Scripts\activate
Once you execute the above command you will the name of the environment in the cmd like shown below.
(flask_venv) D:\flask_tutorial>
Once you activate the environment we can install the flask library using the pip command
pip install Flask
Now we have completed the set up of environment for flask project we can start developing flask app using this environment.
Creating the Flask app
You can create a new file called "app.py" in this file write the below code "Hello World" of web app using flask. and then save the file.
from flask import Flask # Import the Flask Library
app = Flask(__name__) # Create a instance of Flask
# A Python decorator that is used to define the
# route for the web pages
# ('/') is the landing page
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ =="__main__":
app.run(debug=True)
Explanation of the above code:
First we import the Flask library
Next we create a instance of flask app and store it in a variable named app
we use a python decorator to define the route of for the app to take when accessing the site ('/'). This is the landing page when we open a web app.
By using a python function we return the text 'Hello, World!' which will be displayed on the web page
Running The Flask App
We set environment variable for Flask using the following command -
set FLASK_APP=app.py
Run the Flask app using the following command -
flask run

Open your web browser and navigate to http://localhost:5000 or https://127.0.0.1:5000 to see the "Hello, World!" message displayed on the page.
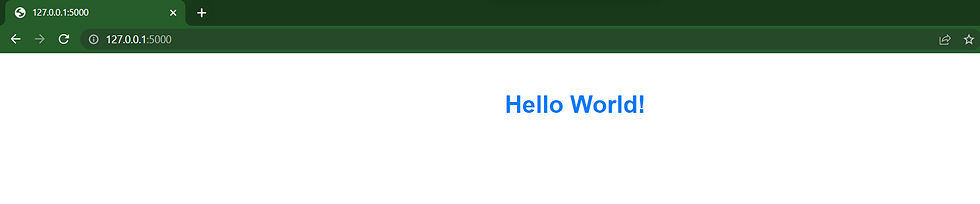
In order to make make it look better Instead of returning a "Hello, World!" we have added some html commands.
Congratulations! You have successfully created a basic Flask application.
With just a few lines of code and some basic command prompt commands, you can create a simple web app using Flask. Give it a try and see what you can build!
If you have any doubts or require any assistance in Flask related coding feel free to contact us.

Comments