Flask is a popular Python web framework that allows developers to create web applications. One of the key features of Flask is its built-in templating engine, which allows developers to create dynamic HTML pages. In this blog, we will cover how to render templates in Flask and provide an example of how to create a basic Flask application.
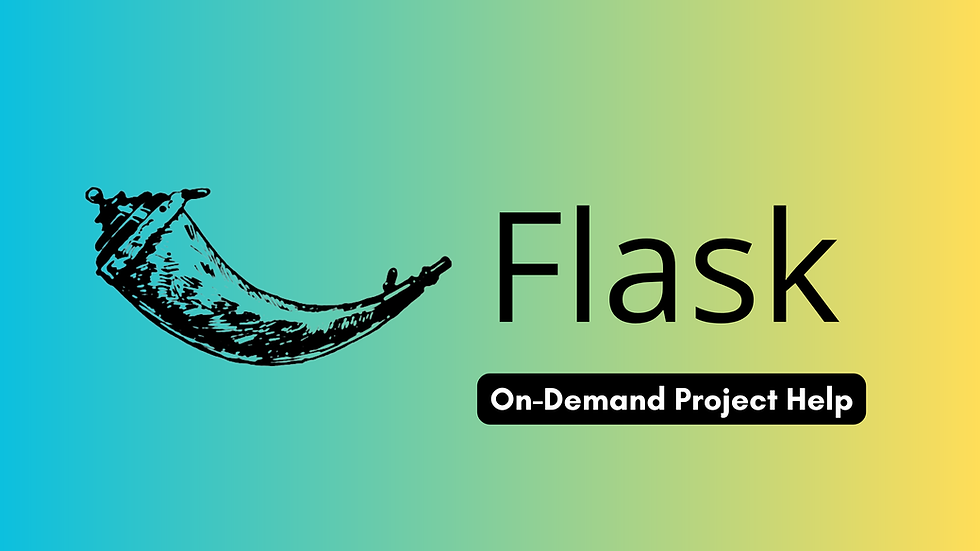
Setting up Flask
Before we dive into rendering templates, let's set up a basic Flask application. You can refer to this blog https://www.codersarts.com/post/flask-for-beginners-create-a-simple-web-app-in-minutes for setting up flask. Now we are using the same app.py here.
Creating a template
Now that we have a basic Flask application set up, let's create a template. Templates are HTML files that include placeholders for dynamic content. In Flask, templates are typically stored in a folder called templates. Create a new folder called templates in the same directory as your app.py file, and create a new file called index.html inside the templates folder. Add the following code to the index.html file:
<!DOCTYPE html>
<html>
<head>
<title>My Flask App</title>
</head>
<body>
<h1>{{ greeting }}</h1>
<p>{{ message }}</p>
</body>
</html>
This code creates a basic HTML page with a single placeholder for dynamic content. The placeholder is defined using the {{ message }} and {{ greeting }}syntax, which tells Flask to replace it with the value of a variable called message.
Rendering a template
Now that we have a template, let's modify our Flask application to render it. Update the hello_world() function first by renaming it to home() in app.py to look like this: ( Note we are importing a new function)
from flask import render_template
@app.route('/')
def home():
greeting = 'Welcome to my Flask app!'
message = 'This is a basic example of rendering a template.'
return render_template('home.html',
greeting=greeting,
message=message)
This code uses the render_template() function to render the home.html template and pass in a variable called message. The value of message is set to "Hello, World!", which will be displayed on the rendered page.
Template inheritance
One of the most powerful features of Flask templates is template inheritance. Template inheritance allows you to define a base template that contains common elements shared across multiple pages in your application, and then define specific templates that inherit from the base template and add or override content as needed.
To illustrate this, let's create a new template called base.html in the templates folder:
<!doctype html>
<html>
<head>
<title>{% block title %}{% endblock %}</title>
</head>
<body>
<header>
<nav>
<ul>
<li><a href="{{ url_for('home') }}">Home</a></li>
<li><a href="{{ url_for('about') }}">About</a></li>
</ul>
</nav>
</header>
<main>{% block content %}{% endblock %}</main>
</body>
</html>
Notice that we have defined a title block in the <title> tag. This block can be overridden by child templates that inherit from this base template.
Now let's create a new template called about.html that inherits from base.html. Create a new file called about.html in the templates folder and add the following code:
{% extends 'base.html' %}
{% block title %} About Us {% endblock %}
{% block content %}
<h1>About Us</h1>
<p>
We are a small company dedicated to creating awesome web applications.
</p>
{% endblock %}
This code uses the {% extends %} tag to inherit from the base.html template, and overrides the title and content blocks to provide custom content for the about.html page.
Now let's update our Flask application to render the about.html template. Add a new route to app.py. Similarly home.html was update to be inherited from the base.html.
Here is the view of the home page now which uses home.html which inherits from the base.html. (Note the base.html has been modified to make it look better)
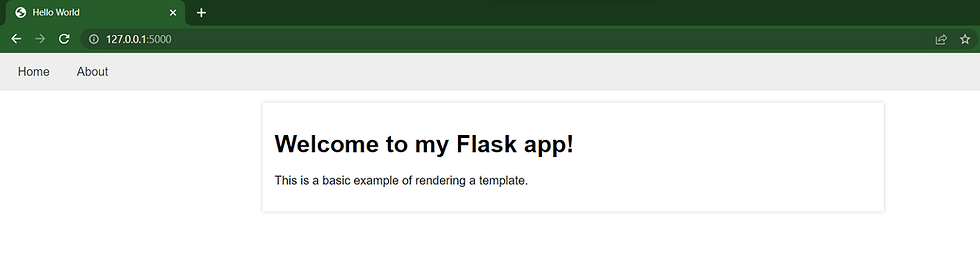
If you want the exact implementation or mentorship to help you learn Flask, feel free to contact us.

Comentarios