Flask For Beginners: Your Guide to Building Forms in Flask
- Pranav S
- Mar 11, 2023
- 3 min read
Updated: Apr 27, 2023
In the previous blog, we learned about Flask and created a simple Flask application that consisted of a home and an about page, which inherited from a base template. In this blog post, we will be focusing on one of the most important aspects of any web application, which is handling user input. Specifically, we will be discussing how to handle forms in Flask.
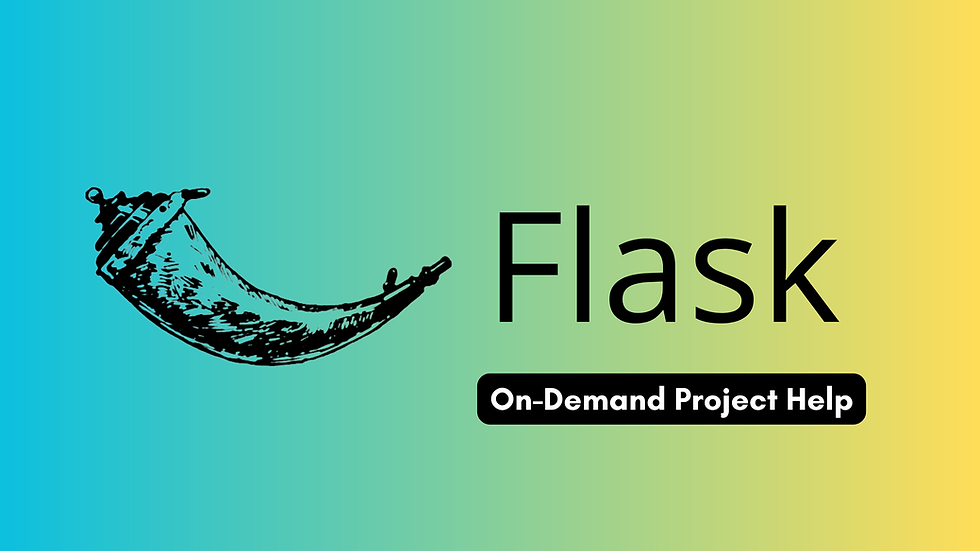
What are Forms?
Forms are a crucial part of any web application as they allow users to input data and interact with the application. Forms can be used for a variety of purposes such as collecting user information, submitting data to a database, or performing an action on the server. In Flask, we can use Flask-WTF, a Flask extension, to handle forms easily.
Installing Flask-WTF
Before we start working with Flask-WTF, we need to install it. We can install Flask-WTF using pip. Open up your terminal and run the following command: ( Make sure install it in out virtual environment)
pip install Flask-WTF
Creating a Form
To create a form, we need to define a class that inherits from FlaskForm, which is a class provided by Flask-WTF. Let's create a simple form that allows the user to input their name and email address. Create a new file called forms.py in the same directory as your Flask application and add the following code:
from flask_wtf import FlaskForm
from wtforms import StringField, SubmitField
from wtforms.validators import DataRequired,Email
class ContactForm(FlaskForm):
name = StringField('Name', validators=[DataRequired()])
email = StringField('Email', validators=[DataRequired(), Email()])
submit=SubmitField('Submit')
In this code, we import FlaskForm from Flask-WTF, as well as StringField and SubmitField from wtforms. StringField represents a text input field, and SubmitField represents a submit button. We also import DataRequired and Email validators from wtforms.validators, which are used to validate the form data. Finally, we define a class called ContactForm that inherits from FlaskForm and defines two fields, name and email, and a submit button.
Rendering the Form
Now that we have defined the form, we need to render it in our Flask application. In our previous blog, we created two routes, / and /about. Let's add a new route called /contact and render the form on that page. Open up your Flask application file and add the following code:
from forms import ContactForm
@app.route('/contact', methods=['GET', 'POST'])
def contact():
form = ContactForm()
if form.validate_on_submit():
# Do something with the form data
pass
return render_template('contact.html',form=form)
In this code, we import the ContactForm class from the forms module we created earlier. We then define a new route called /contact, which accepts both GET and POST requests. We create an instance of ContactForm, and if the form is submitted and passes validation, we can do something with the form data. Finally, we render the contact.html template and pass in the form instance.
Creating the Template
Now that we have defined the form and rendered it in our Flask application, we need to create a template that will render the form to the user. Create a new file called contact.html in your templates folder and do the following
First extend the base template by using the {% extends 'base.html' %} tag
Define a block called content where we will put our form
Create a form element and set its method to POST (<form method="POST">)
We then use the hidden_tag() method of the form instance to include a hidden CSRF token to protect against cross-site request forgery attacks ( {{form.hidden_tag()}} )
Next, we render the name and email fields using their respective labels and fields. Finally, we render the submit button.
Handling Form Submission
Now that we have created the form and rendered it in our template, we need to handle the form submission in our Flask application. If the form is submitted and passes validation, we can do something with the form data. In this example, we will simply print the form data to the console. To do this simply get the names and email from the form data use the print option to print the name and email to the console. A sample way to collect data is shown below.
variable = form.variable.data
In this blog post, we learned about forms in Flask and how to use Flask-WTF to create and handle forms. We created a simple form that allowed users to input their name and email address and learned how to render the form in a template and handle form submission in our Flask application. Forms are an essential part of any web application, and Flask-WTF makes it easy to handle forms in Flask.
To get the exact implementation or guidance on using Flask for your own project or assignment do drop a message to contact@codersarts.com

Comments