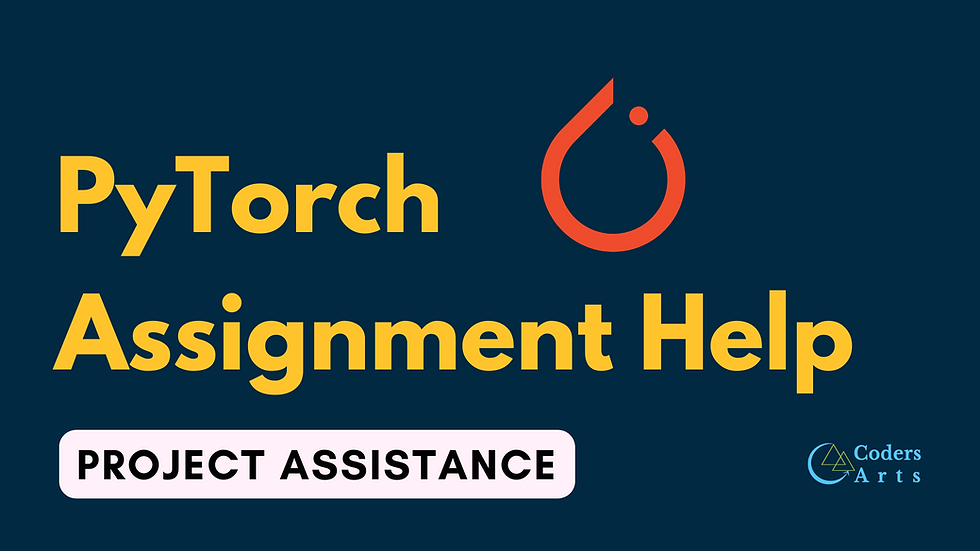
PyTorch Tensors:
Create a PyTorch tensor with values from 0 to 9.
Create a PyTorch tensor of size (3, 3) with all elements initialized to 1.
Create a PyTorch tensor with random values of size (2, 3, 4).
Create a PyTorch tensor of size (5, 5) with all elements initialized to 0.
Add two PyTorch tensors of the same size.
Multiply two PyTorch tensors element-wise.
PyTorch Operations:
Create a PyTorch tensor and compute the sum of its elements.
Create a PyTorch tensor and compute the mean of its elements.
Create a PyTorch tensor and compute its standard deviation.
Create a PyTorch tensor and compute the element-wise absolute values.
Create a PyTorch tensor and compute the natural logarithm of its elements.
Create a PyTorch tensor and compute the exponential of its elements.
PyTorch Autograd:
Create a PyTorch tensor and set its requires_grad attribute to True.
Create a PyTorch tensor and define a computation that involves it and some other tensor.
Compute the gradient of the computation with respect to the tensor with requires_grad=True.
Compute the gradient of a more complex computation with respect to multiple tensors.
Use the detach() method to stop tracking the computation history of a tensor.
Use the with torch.no_grad(): context manager to disable gradient computations.
PyTorch nn.Module:
Define a simple feedforward neural network with one hidden layer.
Define a loss function (e.g. mean squared error) and an optimizer (e.g. stochastic gradient descent).
Train the neural network on a small dataset (e.g. the iris dataset).
Evaluate the trained neural network on a test set and compute its accuracy.
Save and load the trained neural network to/from disk.
PyTorch Data Loading and Preprocessing:
Download a dataset (e.g. the CIFAR-10 dataset).
Write a custom dataset class that loads the dataset and preprocesses it.
Use the DataLoader class to load the dataset in batches.
Apply data augmentation techniques (e.g. random cropping and flipping) to the dataset.
Split the dataset into training and validation sets.
These are just some example questions, and of course the difficulty level can be adjusted depending on the target audience.
Comments