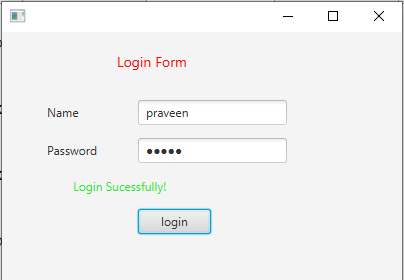
Every database application requires an interface so that the user can interact with the database information. Better, if it is a GUI interface where we do not have to stoop down to a low-level, intimidating command interface but get what we want with a click of a button. In this aspect, JavaFX with JDBC can be a killer combination because it has quite a number of visually exciting GUI components that can be used to represent database records in a more meaningful manner.
A JDBC application with JavaFX essentially means that the JavaFX GUI framework was used as the front-end development engine and JDBC was used for the back-end database interaction.
We will use Oracle XE database and its default HR schema. In order to connect Oracle DB, we will use JDBC driver. JDBC driver is a software component enabling a Java application to interact with a database. To add JDBC driver in our project.
Main.fxml
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.layout.AnchorPane?>
<AnchorPane xmlns:fx="http://javafx.com/fxml/1">
<!-- TODO Add Nodes -->
</AnchorPane>
Login.fxml
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.control.PasswordField?>
<?import javafx.scene.control.TextField?>
<?import javafx.scene.layout.AnchorPane?>
<?import javafx.scene.text.Font?>
<AnchorPane prefHeight="253.0" prefWidth="339.0" xmlns="http://javafx.com/javafx/11.0.1" xmlns:fx="http://javafx.com/fxml/1" fx:controller="application.LoginController">
<children>
<PasswordField fx:id="password" layoutX="136.0" layoutY="106.0" promptText="password" />
<Button layoutX="-39.0" layoutY="-123.0" mnemonicParsing="false" text="Button" />
<Button layoutX="136.0" layoutY="177.0" mnemonicParsing="false" onAction="#Login" prefHeight="25.0" prefWidth="73.0" text="login" />
<Label layoutX="115.0" layoutY="14.0" prefHeight="32.0" prefWidth="73.0" text="Login Form" textFill="#fc0000">
<font>
<Font size="14.0" />
</font>
</Label>
<Label layoutX="45.0" layoutY="64.0" prefHeight="32.0" prefWidth="61.0" text="Name" />
<Label layoutX="45.0" layoutY="110.0" prefHeight="17.0" prefWidth="61.0" text="Password" />
<TextField fx:id="userName" layoutX="136.0" layoutY="68.0" promptText="userName" />
<Label fx:id="lebel" layoutX="71.0" layoutY="138.0" prefHeight="32.0" prefWidth="197.0" textFill="#27e532" />
</children>
</AnchorPane>
LoginController.java
package application;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
public class LoginController {
@FXML
private TextField userName;
@FXML
private TextField password;
@FXML
private Label lebel;
PreparedStatement pstmt=null;
Connection con=null;
ResultSet rs=null;
public void Login(ActionEvent event){
try {
con=DBUtil.getConnection();
con.createStatement();
String sql = "select * from login where username=? and password=?";
pstmt = con.prepareStatement(sql);
pstmt.setString(1,userName.getText());
pstmt.setString(2, password.getText());
rs = pstmt.executeQuery();
System.out.println(rs);
if(rs.next()) {
lebel.setText("Login Sucessfully!");
}else{
lebel.setText("Login Not Sucessfully!");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
DBUtil.java
package application;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public final class DBUtil {
private static boolean isDriverLoaded = false;
static{
try{
Class.forName("oracle.jdbc.driver.OracleDriver");
System.out.println("Driver Loaded");
isDriverLoaded = true;
}catch(ClassNotFoundException e){
e.printStackTrace();
}
}
private final static String url="jdbc:oracle:thin:@localhost:1521:XE";
private final static String user="SYSTEM";
private final static String password="system";
public static Connection getConnection() throws SQLException{
Connection con = null;
if(isDriverLoaded){
con = DriverManager.getConnection(url,user,password);
System.out.println("Connection established");
}
return con;
}
public static void closeConnection(Connection con) throws SQLException{
if(con!=null){
con.close();
System.out.println("connection closed");
}
}
}
DBUtil Class
I want to start to explain the code to DBUtil class. In our example, DBUtil class is responsible for DB connection, DB disconnection, database query operations.
– getConnection() method connects to DB.
– closeConnection() method closes DB connection.
Main.java
package application;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
try {
Parent root =FXMLLoader.load(getClass().getResource("/application/login.fxml"));
Scene scene = new Scene(root,400,400);
scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm());
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
launch(args);
}
}
Comments