How To Implement GET and POST method in Django
- Pushkar Nandgaonkar
- Jan 6, 2023
- 5 min read
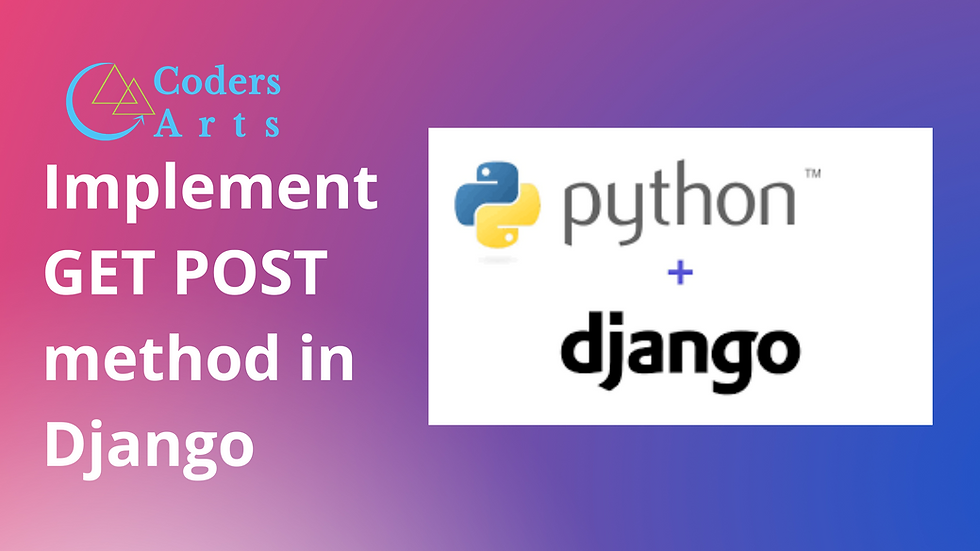
How to use GET and POST method in Django
Hello everyone!!
In this article we are going to discuss the GET and POST method in django, what it is, how it works and how to use it.
Django provides built in features of django form like django model. We can create forms in django and use them to fetch data from the user. To get started with forms we need to know about GET and post requests in forms.
What is the GET and POST method?
GET method : This method we use to submit bundled data into string and use this to compose the url. Where the data must be sent, key, values and address these information are contained in the url.
POST method : This method is used to change the state of the system for example a request that makes changes in the database.
Lets see how to use the Get and post method we will show you step by step in this article.
Step 1
Create Virtual Environment
First of all, create an empty folder and create a virtual environment in it. After that install django in it. Then create a project in that folder. How to create virtual environments, installation packages, how to activate virtual environments in visual studio all have been explained in my previous blog. If you don't know how to create a virtual environment, activate virtual environment and install packages please read my previous blog. Link Click here
Step 2
Now here I consider you created a django project.
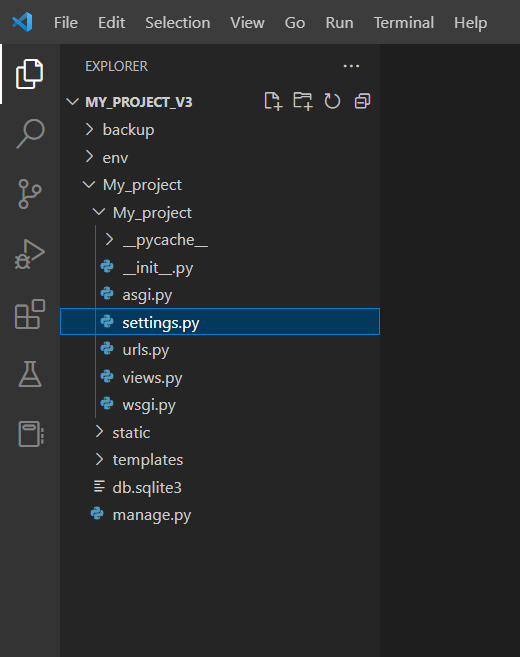
Now running the project to ensure that the out project is working correctly. Run the following command.
Python .\manage runserver
In this code use GET method
My_project>My_project>views.py
from django.http import HttpResponse
from django.shortcuts import render
def HomePage(request):
return render(request,"index.html")
def Form(request):
try:
res=0
f_num=request.GET['fnum']
s_num = request.GET['snum']
res = int(f_num) +int(s_num)
except:
pass
return render(request,"form.html", {'result': res})
Then create a new folder in testdb “templates”. In this folder contains all webpage related files html files.
My_project>My_project>urls.py
from django.contrib import admin
from django.urls import path
from My_project import views
urlpatterns = [
path('admin-panel/', admin.site.urls),
#path('home/',views.Home),
path('',views.HomePage),
path('form/',views.Form),
]
Then create a new folder in testdb “templates”. In this folder contains all webpage related files html files.
My_project>templates>index.html
<!DOCTYPE html>
<html>
<head>
<title>
CodersArts
</title>
<style>
/* CSS property for header section */
.header {
background-color: rgb(66, 156, 216);
padding: 5px;
font-size: 20px;
}
/* CSS property for navigation menu */
.nav_menu {
overflow: hidden;
background-color: rgb(19, 6, 6);
font-size: larger;
}
.nav_menu a {
float: left;
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
.nav_menu a:hover {
background-color: white;
color: green;
}
article {
float: left;
padding: 20px;
height: 350px;
width: 100%;
background-color: white;
/* only for demonstration, should be removed */
text-align: ;
}
/* Style for footer section */
.footer {
background-color: rgb(18, 24, 18);
padding: 15px;
text-align:justify;
color: white;
}
</style>
</head>
<body>
<!-- header of website layout -->
<div class = "header">
<h1 style = "color:white;font-size:200%;">
CodersArts
</h1>
</div>
<!-- navigation menu for website layout -->
<div class = "nav_menu">
<a href = "/">Home</a>
<a href = "#">Programming Language</a>
<a href = "#">Data Science</a>
<a href = "form/">Form</a>
</div><br>
<center style = "font-size:200%; text-align: left; text-align: center;" >
Need Help in Programming Task?
</center>
<article>
<p style="font-size: 25px;">CodersArts is trusted and top rated leading website for help in Programming Assignment, Coursework, Coding, and Project. Get help from vetted software developers, engineers, ML Researchers and programming experts.</p>
</article>
<!-- footer Section -->
<div class = "footer">
<a href = "#" style="color: white;">About</a><br>
<a href = "#" style="color: white;">Career</a><br>
<a href = "form/" style="color: white;">Contact Us</a>
</div>
</body>
</html>
My_project>templates>form.html
<!DOCTYPE html>
<html>
<head>
<title>
CodersArts
</title>
<style>
/* CSS property for header section */
.header {
background-color: rgb(66, 156, 216);
padding: 5px;
font-size: 20px;
}
/* CSS property for navigation menu */
.nav_menu {
overflow: hidden;
background-color: rgb(19, 6, 6);
font-size: larger;
}
.nav_menu a {
float: left;
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
.nav_menu a:hover {
background-color: white;
color: green;
}
article {
float: left;
padding: 20px;
height: 350px;
width: 100%;
background-color: white;
/* only for demonstration, should be removed */
text-align: ;
}
/* Style for footer section */
.footer {
background-color: rgb(18, 24, 18);
padding: 15px;
text-align:justify;
color: white;
}
</style>
</head>
<body>
<!-- header of website layout -->
<div class = "header">
<h1 style = "color:white;font-size:200%;">
CodersArts
</h1>
</div>
<!-- navigation menu for website layout -->
<div class = "nav_menu">
<a href = "/">Home</a>
<a href = "#">Programming Language</a>
<a href = "#">Data Science</a>
<a href = "http://127.0.0.1:8000/form/">Form</a>
</div><br>
<center style = "font-size:200%; text-align: left;" >
Addition of Two Numbers
</center>
<article>
<form action="">
<div>
<label for="fnum" style="font-size: 22px;">First Number</label><br>
<input type="text" name="fnum" size="50" maxlength="15"><br><br>
<label for="snum" style="font-size: 22px;">Second Number</label><br>
<input type="text" name="snum" size="50" maxlength="15"><br><br>
<button type="submit">Submit</button>
<input type="text" value="{{result}}"/>
</div>
</form>
</article>
<!-- footer Section -->
<div class = "footer">
<a href = "#" style="color: white;">About</a><br>
<a href = "#" style="color: white;">Career</a><br>
<a href = "#" style="color: white;">Contact Us</a>
</div>
</body>
</html>
Now run the code. You can see if we use the GET method, input data is shown in the url bar.
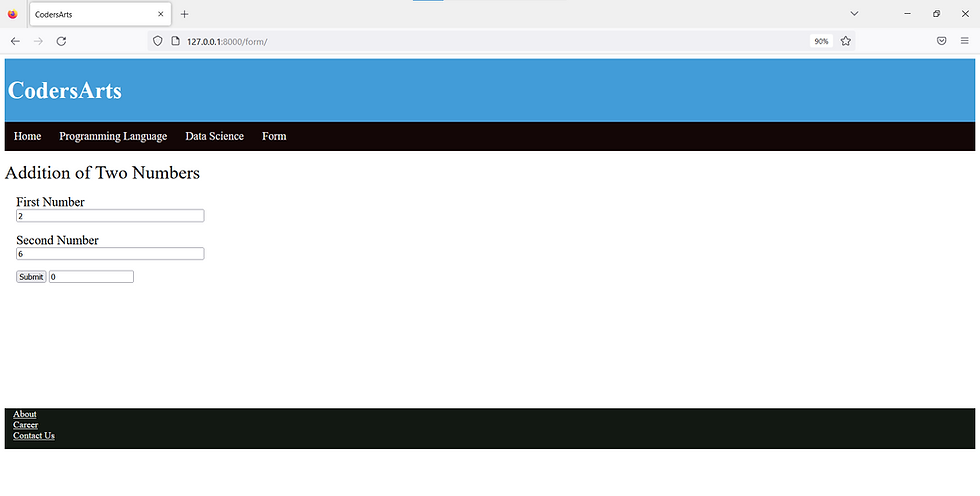
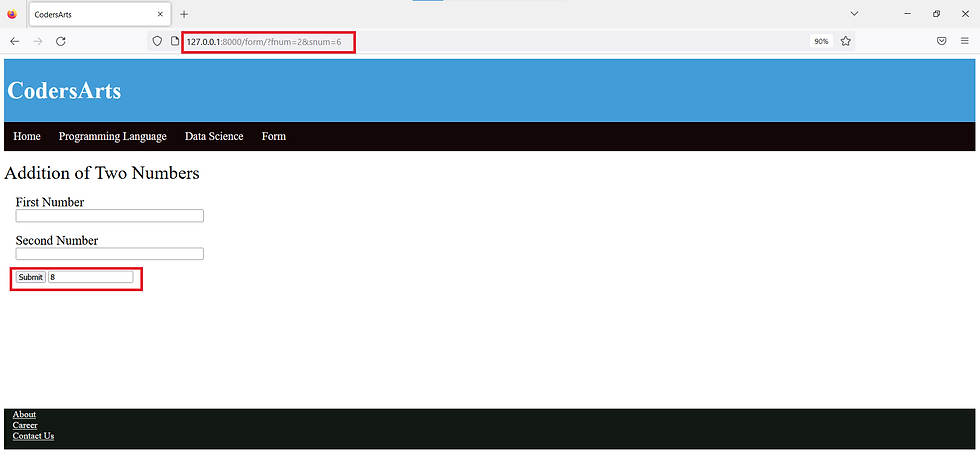
Now I am using the POST method. So that I am going to changes in the code view.py file and form.html
My_project>My_project>views.py
from django.http import HttpResponse
from django.shortcuts import render
def HomePage(request):
return render(request,"index.html")
def Form(request):
res=0
data={}
try:
if request.method=='POST':
f_num=request.POST.get('fnum')
s_num = request.POST.get('snum')
res = int(f_num) +int(s_num)
data = {"f_num":f_num,"s_num":s_num,"res":res}
except:
pass
return render(request,"form.html", data)
whenever we create a form request, Django requires you to add IN form section {% csrf_token %} in form for security purposes
My_project>templates>form.html
<!DOCTYPE html>
<html>
<head>
<title>
CodersArts
</title>
<style>
/* CSS property for header section */
.header {
background-color: rgb(66, 156, 216);
padding: 5px;
font-size: 20px;
}
/* CSS property for navigation menu */
.nav_menu {
overflow: hidden;
background-color: rgb(19, 6, 6);
font-size: larger;
}
.nav_menu a {
float: left;
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
.nav_menu a:hover {
background-color: white;
color: green;
}
article {
float: left;
padding: 20px;
height: 350px;
width: 100%;
background-color: white;
/* only for demonstration, should be removed */
text-align: ;
}
/* Style for footer section */
.footer {
background-color: rgb(18, 24, 18);
padding: 15px;
text-align:justify;
color: white;
}
</style>
</head>
<body>
<!-- header of website layout -->
<div class = "header">
<h1 style = "color:white;font-size:200%;">
CodersArts
</h1>
</div>
<!-- navigation menu for website layout -->
<div class = "nav_menu">
<a href = "/">Home</a>
<a href = "#">Programming Language</a>
<a href = "#">Data Science</a>
<a href = "http://127.0.0.1:8000/form/">Form</a>
</div><br>
<center style = "font-size:200%; text-align: left;" >
Addition of Two Numbers
</center>
<article>
<form method="POST">
{% csrf_token %}
<div>
<label for="fnum" style="font-size: 22px;">First Number</label><br>
<input type="text" name="fnum" value="{{f_num}}" size="50" maxlength="15"><br><br>
<label for="snum" style="font-size: 22px;">Second Number</label><br>
<input type="text" name="snum" value="{{s_num}}" size="50" maxlength="15"><br><br>
<button type="submit">Submit</button>
<input type="text" value="{{res}}"/>
</div>
</form>
</article>
<!-- footer Section -->
<div class = "footer">
<a href = "#" style="color: white;">About</a><br>
<a href = "#" style="color: white;">Career</a><br>
<a href = "#" style="color: white;">Contact Us</a>
</div>
</body>
</html>
Now run the project using this command
python .\manage.py runserver
You can see that when we use the POST method the input data is secure, it is not shown on the address bar.
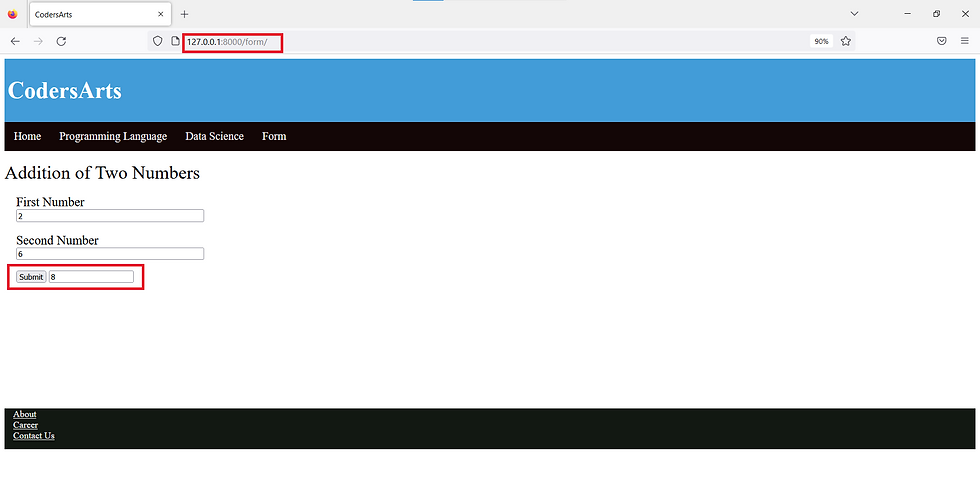
Need more help in Django Projects ?
Django Assignment help
Django Error Resolving Help
Mentorship in Django from Experts
Django Development Project
If you are looking for help in Django project contact us contact@codersarts.com

Comments