Spring Boot is an open-source Java framework that is designed to simplify the process of building and deploying production-grade, standalone Spring-based applications. It provides developers with a pre-configured set of tools and conventions that allow them to focus on writing application code rather than boilerplate configuration.
Spring Boot is built on top of the Spring Framework and leverages its existing features, such as dependency injection and inversion of control, to provide a more streamlined development experience. It also includes a number of additional features, such as embedded servers, auto-configuration, and production-ready metrics, which make it easier to build, package, and deploy Spring-based applications.
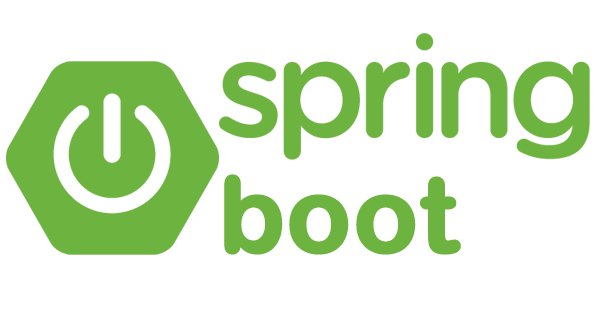
What Spring boot adds to Spring framework
Auto-configuration: Spring Boot provides a set of default configurations for many common Spring features, which can be overridden or customized as needed.
Embedded servers: Spring Boot includes an embedded servlet container (such as Tomcat, Jetty, or Undertow) that can be used to run and test applications without the need for a separate server.
Developer tools: Spring Boot includes a number of development tools and plugins that integrate with popular IDEs, making it easier to write and test code.
Production-ready metrics: Spring Boot includes a number of features that make it easier to monitor and manage applications in production, such as health checks, metrics, and tracing.
Actuator: Spring Boot's Actuator is a feature that provides endpoints for monitoring and managing an application's health, metrics, and other useful information.
Spring Boot Architecture
The spring boot follows a layered architecture in which each layer communicates to other layers.
The spring boot consists of the following four layers:
Presentation Layer – Authentication & JSON Translation
Business Layer – Business Logic, Validation & Authorization
Persistence Layer – Storage Logic
Database Layer – Actual Database
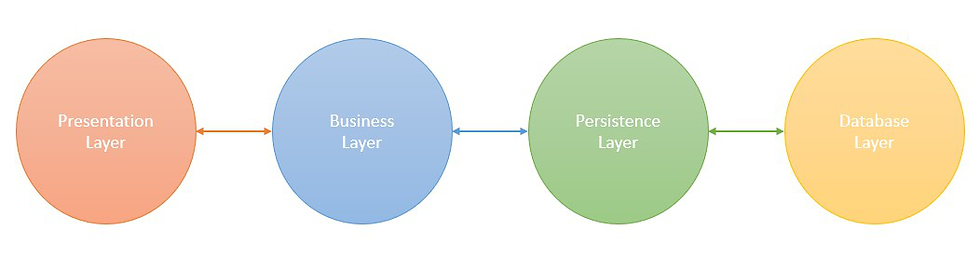
1. Presentation Layer
The presentation layer is the top layer of the spring boot architecture. It consists of Views. i.e., the front-end part of the application. It handles the HTTP requests and performs authentication. It is responsible for converting the JSON field’s parameter to Java Objects and vice-versa. Once it performs the authentication of the request it passes it to the next layer. i.e., the business layer.
2. Business Layer
The business layer contains all the business logic. It consists of services classes. It is responsible for validation and authorization.
3. Persistence Layer
The persistence layer contains all the database storage logic. It is responsible for converting business objects to the database row and vice-versa
4. Database Layer
The database layer contains all the databases such as MySQL, MongoDB, etc. This layer can contain multiple databases. It is responsible for performing the CRUD operations.
Spring Boot Flow Architecture
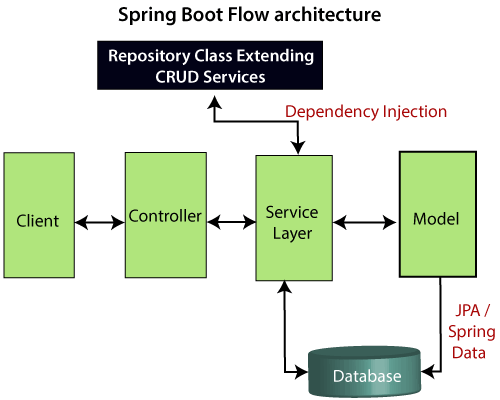
The Client makes an HTTP request(GET, PUT, POST, etc.)
The HTTP request is forwarded to the Controller. The controller maps the request. It processes the handles and calls the server logic.
The business logic is performed in the Service layer. The spring boot performs all the logic over the data of the database which is mapped to the spring boot model class through Java Persistence Library(JPA).
The JSP page is returned as Response from the controller.
Spring Boot Use Cases
RESTful web services: Spring Boot makes it easy to build RESTful web services, which are often used for building web APIs that can be consumed by other applications or services.
Microservices: Spring Boot's lightweight and modular architecture make it well-suited for building microservices, which are small, independent services that work together to build larger applications.
Web applications: Spring Boot can be used to build web applications that run on a web server, such as Tomcat or Jetty. It includes support for popular web technologies such as Thymeleaf, JSP, and Spring WebFlux.
Batch processing: Spring Boot provides support for batch processing, which involves processing large volumes of data in batches. This can be useful for tasks such as generating reports or processing large data sets.
Internet of Things (IoT): Spring Boot can be used to build IoT applications, which involve connecting physical devices to the internet and collecting data from them. It includes support for protocols such as MQTT and AMQP.
Real-time data processing: Spring Boot provides support for real-time data processing using technologies such as WebSockets and Spring WebFlux.
Cloud-native applications: Spring Boot is well-suited for building cloud-native applications, which are designed to run on cloud infrastructure such as AWS, Azure, or Google Cloud Platform. It includes support for cloud-native technologies such as Spring Cloud Config and Spring Cloud Netflix.
Overall, Spring Boot's flexibility and ease of use make it a popular choice for a wide range of applications, from simple web services to complex microservices architectures.
Spring Boot Community and Resources
Spring Boot has a large and active community, with many resources available to help developers learn and use the framework. Here are some of the key community and resources for Spring Boot:
Spring Boot Documentation: The official documentation for Spring Boot is a comprehensive resource that covers all aspects of the framework, including getting started guides, reference documentation, and tutorials. Click here to visit the website
Spring Boot Guides: Spring Boot provides a series of guides that cover a wide range of topics, from building a simple web application to integrating with databases and deploying to the cloud. Click here to visit the website
Spring Boot GitHub Repository: The source code for Spring Boot is hosted on GitHub, where developers can report bugs, request features, and contribute to the project.
Stack Overflow: Stack Overflow is a popular Q&A site where developers can ask and answer questions about Spring Boot and other programming topics. Click here to visit the website
Codersarts: Codersarts provide assistance in developing projects related to Java, Spring Boot, Angular, react and many more technologies. Click here to visit the website
Overall, the Spring Boot community is very active and supportive, with many resources available to help developers learn and use the framework effectively.
Future of Spring Boot
Spring Boot is a popular and widely used framework, and its future looks bright. Here are some potential directions for Spring Boot in the future:
Continued support for cloud-native architectures: As more and more applications move to the cloud, Spring Boot is well-suited to help developers build cloud-native applications that are scalable, resilient, and easy to deploy.
More support for reactive programming: Spring Boot currently supports reactive programming using Spring WebFlux, and it's likely that this support will continue to grow in the future as more developers adopt reactive programming techniques.
Improved support for microservices: Spring Boot is already a popular choice for building microservices, and it's likely that future versions of the framework will continue to improve support for building and managing microservices architectures.
Better integration with serverless computing platforms: Serverless computing platforms such as AWS Lambda and Azure Functions are becoming increasingly popular, and it's likely that Spring Boot will continue to improve its integration with these platforms to make it easier for developers to build serverless applications.
Increased focus on developer productivity: Spring Boot is already known for its ease of use and developer productivity, and future versions of the framework are likely to continue to prioritize these qualities.
Overall, Spring Boot is a mature and stable framework that is likely to continue to be a popular choice for building Java-based web applications and microservices in the years to come. Its focus on productivity, scalability, and cloud-native architectures make it well-suited to the needs of modern application development.
Comentários