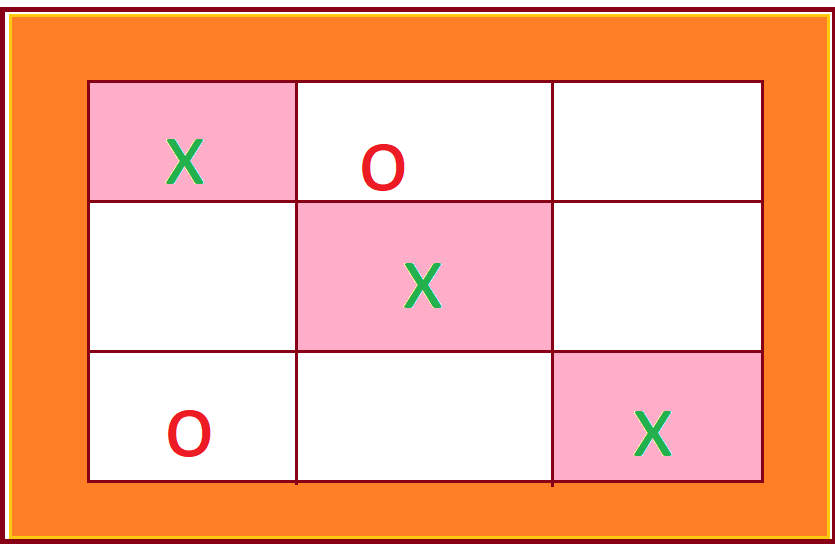
Use Events and DOM manipulation to create a playable Tic Tac Toe game. Do the following using only JavaScript. Do not modify the CSS file, the HTML file, or the winner.js file. The script tags are already included in the HTML file. You will not be able to write all these steps in order in your code. In some cases you have to go to specific locations in the code to add the next step. When you see this symbol save and refresh the browser (this should happen automatically if you are using Brackets with Live Preview), then check to make sure it is working before you proceed. This will make debugging much easier than if you wait until you have written a lot of code before trying it. Open the CSS files so you can reference it as you go through the steps. Note that class and variable names that refer to X and O are referring to letter O not the number zero.
1. Create two variables called playerX and playerO. Set them to the DOM element with the id "x" and "o" respectively. This is the text at the top that says who's turn it is.
2. Create a named function with a meaningful name of your choosing. (This will be referred to as the "event handler" in further instructions.) In this function add a console.log() statement to display a message in the console.
3. Create a variable that contains all of the TD elements. Use a for loop or the .forEach() method to add the function as a click event handler to each of the TD elements. Be sure to use .addEventListener() rather than older methods of adding event handlers. Open the console and click a square. You should see the message from your console.log.
4. In your event handler, add a statement to get the id value of the element with the class "currentplayer" and store it to a variable. Display this value using the same console.log() you already have. Try clicking a square to see the value.
5. At the end of your event handler, add a statement to switch the "current-player" class from the playerX to playerO elements or visa-versa. (Remember you can toggle classes.) Try clicking a few squares, you should see the player at the top of the page change as well as the output in the console.
6. In your event handler, add a statement to add the class "x" or "o" to the target element depending on who's turn it is. (the target element is the one that was clicked on. Hint: the event object has a target property.) You should see "X" or "O" appear in the square whenyou click on it. (The letter is displayed using CSS, refer to that file if you want to see how it is done.)
7. Add an event handler to the "New Game" button to clear all the squares.
8. Notice that if you click on a square that is taken by the other player, the marker may turn into a question mark. This is because you have added both "x" and "o" classes to the document. If you used className instead of classList you would be able to take over another players square. Also, if you click on your own square you wasted your turn. Modify the event handler to check to see if there is a class on the element already. (Hint: this is a good use of className but there are other ways to do it.) Don't put a marker or count the turn if the square is already taken.
9. Notice that there is a file called winner.js already referenced from the HTML file. This contains a function called checkWin(). Since it is included before the main.js file, you can call this function from your code. You can examine this function so that you understand how it works. Call the checkWin() function from the event handler. The first argument is a string identifying the player, "x" or "o". The second argument is the list of td elements you selected previously.
10. If there is a winner the checkWin() function will return an array of the winning elements, set the background colour to yellow of the three winning squares to highlight them and open an alert box to say who the winner is. Depending on which browser you use, you may not see the last marker or the highlight color until you close the alert box. This is fine. There is a trick to fix this but don't worry about it.
11. In the event handler for the "New Game" button, add code to remove the background colour. Play Tic Tac Toe!
Contact us for this JavaScript assignment Solutions by Codersarts Specialist who can help you mentor and guide for such JavaScript assignments.
If you have project or assignment files, You can send at contact@codersarts.com directly
Comments