In this example we are seeing how to develop Pie Chart application using technologies JavaFX.
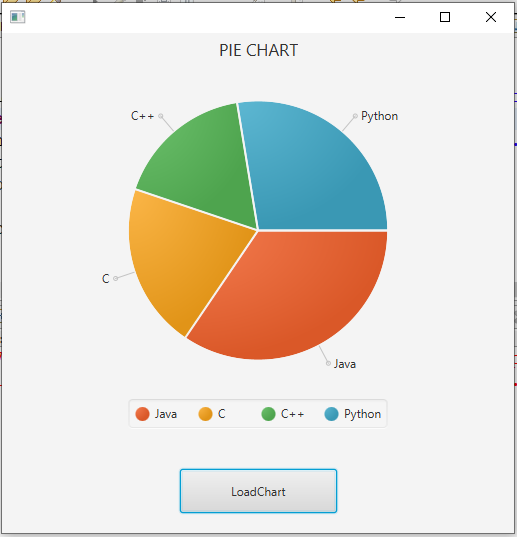
A pie-chart is a representation of values as slices of a circle with different colors. These slices are labeled and the values corresponding to each slice is represented in the chart.
In JavaFX, a pie chart is represented by a class named PieChart. This class belongs to the package javafx.scene.chart.
This class has 5 properties which are as follows −
clockwise − This is a Boolean Operator; on setting this operator true, the data slices in the pie charts will be arranged clockwise starting from the start angle of the pie chart.
data − This represents an ObservableList object, which holds the data of the pie chart.
labelLineLength − An integer operator representing the length of the lines connecting the labels and the slices of the pie chart.
labelsVisible − This is a Boolean Operator; on setting this operator true, the labels for the pie charts will be drawn. By default, this operator is set to be true.
startAngle − This is a double type operator, which represents the angle to start the first pie slice at.
PieChart.fxml
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.chart.PieChart?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.layout.AnchorPane?>
<AnchorPane prefHeight="500.0" prefWidth="500.0" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/11.0.1" fx:controller="application.PieChartController">
<children>
<PieChart fx:id="pieChart" prefHeight="400.0" prefWidth="512.0" title="PIE CHART" />
<Button layoutX="178.0" layoutY="436.0" mnemonicParsing="false" onAction="#generateChart" prefHeight="44.0" prefWidth="157.0" text="LoadChart" />
</children>
</AnchorPane>
PieChartController.java
package application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.chart.PieChart;
import javafx.scene.chart.PieChart.Data;
public class PieChartController {
@FXML
PieChart pieChart;
public void generateChart(ActionEvent ae){
ObservableList<Data> list=FXCollections.observableArrayList(
new PieChart.Data("Java",50),
new PieChart.Data("C",30),
new PieChart.Data("C++",25),
new PieChart.Data("Python",40)
);
pieChart.setData(list);
}
}
Main.java
package application;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.stage.Stage;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
try {
Parent root=FXMLLoader.load(getClass().getResource("/application/PieChart.fxml"));
Scene scene = new Scene(root);
scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm());
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
launch(args);
}
}
Comments