Registration Form in Angular 8
- praveen3197
- Sep 15, 2020
- 2 min read
Updated: Nov 5, 2020
To create a new workspace and an initial app project
Ensure that you are not already in an Angular workspace folder. For example, if you have previously created the Getting Started workspace, change to the parent of that folder.
Run the CLI command ng new and provide the name Registration, as shown here:
ng new Registration
3. The ng new command prompts you for information about features to include in the initial app project. Accept the defaults by pressing the Enter or Return key.
Angular components
The page you see is the application shell. The shell is controlled by an Angular component named AppComponent.
Components are the fundamental building blocks of Angular applications. They display data on the screen, listen for user input, and take action based on that input.
Make changes to the application
Open the project in your favorite editor or IDE and navigate to the src/app folder to make some changes to the starter app.
registration.component.html
the component template, written in HTML.
<form [formGroup]="frmValue" (ngSubmit)="login()">
<table>
<tr>
<td>Reg No:</td> <td><input type="number" placeholder="Registration No." formControlName="regNo"></td>
</tr>
<tr>
<td>Name:</td><td><input type="text" placeholder="name" formControlName="fristName"></td>
</tr>
<tr>
<td>Descipline:</td><td><select formControlName="descipline">
<option *ngFor="let dis of Discipline" value="{{dis}}">{{dis}}</option>
</select></td>
</tr>
<tr>
<td>Gender:</td><td><input type="radio" value="male" name="gender" formControlName="gender">male
<input type="radio" value="female" name="gender" formControlName="gender">female</td>
</tr>
<tr>
<td>Subjects:</td><td>
<div *ngFor="let subject of subjectData">
<input type="checkbox" (change)="onChange(subject.name, $event.target.checked)">
{{ subject.name}}
</div></td>
</tr>
<tr>
<td><input type="submit" value="View on Console"></td><td><input type="submit" value="Store in File"></td>
</tr>
</table>
</form>
registration.component.ts
the component class code, written in TypeScript.
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, FormControl, FormArray } from '@angular/forms';
export interface Subject {
id: number;
name: string;
}
@Component({
selector: 'app-login',
templateUrl: './login.component.html',
styleUrls: ['./login.component.css']
})
export class LoginComponent implements OnInit {
frmValue:FormGroup;
record:any;
constructor(private formBuilder:FormBuilder) { }
Discipline: any = ['MCS', 'WMC', 'DIF'];
subjectData: Subject[] = [
{ id: 0, name: 'MPL' },
{ id: 1, name: 'OOP' },
{ id: 2, name: 'OS' },
{ id: 3, name: 'PF' },
{ id: 2, name: 'VP' }
];
ngOnInit(): void {
this.frmValue=this.formBuilder.group({
regNo:[''],
fristName:[''],
gender: [''],
descipline:[''],
name: this.formBuilder.array([])
})
}
onChange(name: string, isChecked: boolean) {
const sub = (this.frmValue.controls.name as FormArray);
if (isChecked) {
sub.push(new FormControl(name));
} else {
const index = sub.controls.findIndex(x => x.value === name);
sub.removeAt(index);
}
}
login(){
this.record=this.frmValue;
console.log("Registration No. ....."+this.frmValue.value.regNo);
console.log("Name .............."+this.frmValue.value.fristName);
console.log("Gender ....... ...."+this.frmValue.value.gender);
console.log("descipline ........"+this.frmValue.value.descipline);
console.log("Subject Name ........"+this.frmValue.value.name);
}
}
app.routing.module.ts
Routing basically means navigating between page.
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LoginComponent } from './login/login.component';
const routes: Routes = [
{path:'',redirectTo:'/login',pathMatch:'full'},
{path:'login',component:LoginComponent}
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
export const routingComponents=[LoginComponent
]
app.module.ts
the main parent component app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule,ReactiveFormsModule } from '@angular/forms';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule,
ReactiveFormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Run the application
The ng serve command launches the server, watches your files, and rebuilds the app as you make changes to those files.
The --open (or just -o) option automatically opens your browser to http://localhost:4200/.
ng serve -o
Output
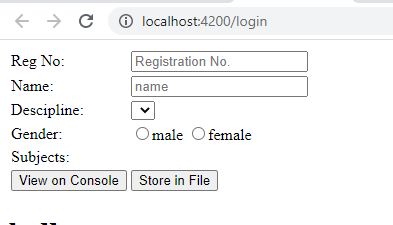
Komentari