Introduction
In programming when working with arrays, it is not uncommon for us to remove overlapping elements while saving the order of other elements. This problem often occurs when data needs to be processed or analyzed, and duplicate values can distort the results or cause unnecessary overhead. let's explore a common programming challenge: removing duplicates from a sorted array in place. We address the problem in detail and present an efficient Java solution that preserves the relative order of the elements and returns the number of unique elements in the modified array.
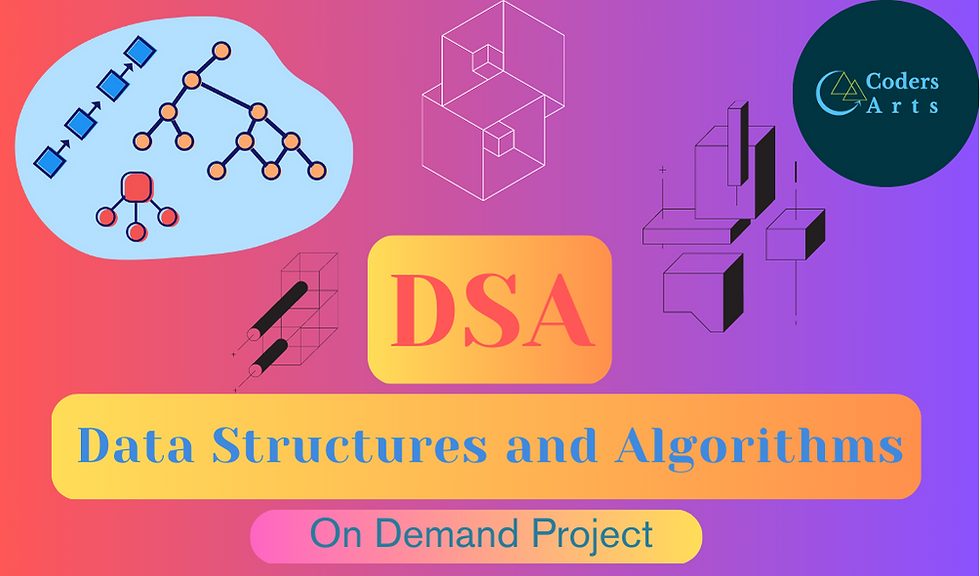
Problem Statement
Given an integer array, 'nums', that is sorted in non-decreasing order, our task is to remove the duplicate elements in place. The resulting array should contain each unique element only once, and the relative order of the elements should be maintained. Our goal is to return the number of unique elements present in the modified array.
Algorithm
Create an empty 'Map<Integer, Integer> ' to store the unique elements as a key.
Iterate through the input array and check if the elements are present in the array and add to the map. if the element is already present then increment its value and if not then add an element.
Call a for loop on the map and get the all keys and store them in the array.
Return the resulting array.
Code
import java.util.*;
public class Main {
public int removeDuplicate(int[] nums) {
// To store the elements in the map
Map<Integer, Integer> map = new HashMap<>();
// add elements in map and update their value
for (int num : nums) {
map.put(num, map.getOrDefault(num, 0) + 1);
}
// update the orginal array
int index = 0;
for (int key : map.keySet()) {
nums[index++]=key;
}
// return the elements size
return map.size();
}
public static void main(String[] args) {
int[] nums = {1, 1, 2, 2, 2, 3, 4, 5, 5, 6};
Main main=new Main();
int k = main.removeDuplicate(nums);
System.out.println("unique element size " + k);
System.out.print("unique elements: ");
for (int i = 0; i < k; i++) {
System.out.print(nums[i] + " ");
}
}
}
At codersArts:
Expert Assistance: Our team consists of highly skilled Java, and Spring Boot developers who have extensive experience in the field. They are proficient in the latest tools, frameworks, and technologies, ensuring top-notch solutions for your assignments, Projects.
Timely Delivery: We understand the importance of deadlines. Our experts work diligently to deliver your completed assignments, Projects on time, allowing you to submit your work without any worries.
Customized Solutions: We believe in providing personalized solutions that meet your specific requirements. Our experts carefully analyze your assignment or Project and provide tailored solutions to ensure your success.
Affordable Pricing: We offer competitive and affordable pricing to make our services accessible to students, Developers. We understand the financial constraints that students, Developers often face, and our pricing is designed to accommodate their needs.
24/7 Support: Our customer care team is available round the clock to assist you with any queries or concerns you may have. You can reach us via phone, email, or live chat.
Contact CodersArts today for reliable and professional Spring Boot, Spring Security, and Java help. Let our experts take your Java programming skills to the next level!

Comments