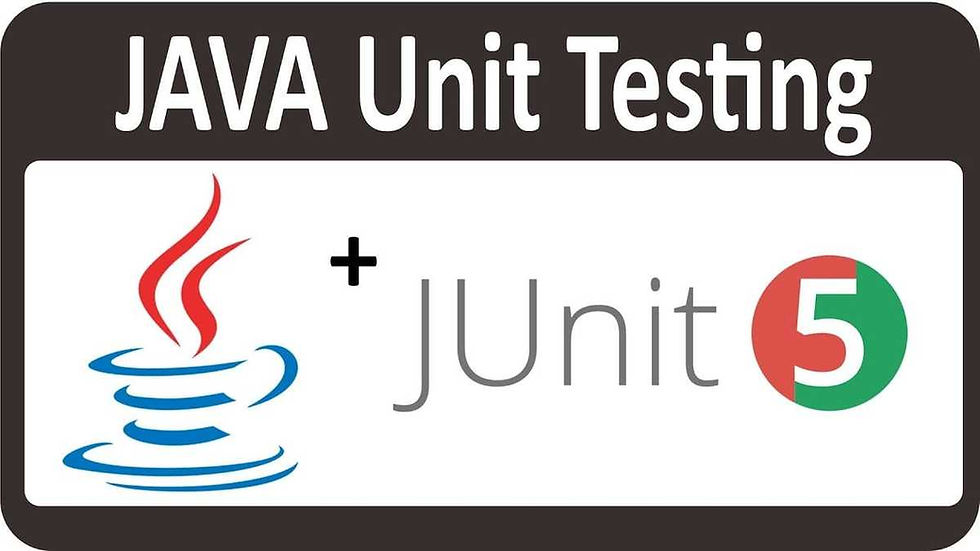
JUnit is the most popular Java Unit testing framework
We typically work in large projects - some of these projects have more than 2000 source files or sometimes it might be as big as 10000 files with one million lines of code.
Before unit testing, we depend on deploying the entire app and checking if the screens look great. But that’s not very efficient. And it is manual.
Unit Testing focuses on writing automated tests for individual classes and methods.
JUnit is a framework which will help you call a method and check (or assert) whether the output is as expected.
The important thing about automation testing is that these tests can be run with continuous integration - as soon as some code changes.
TestController.java
package com.example.demo.controller;
public class TestController {
public int sum(int[] numbers) {
int sum = 0;
for (int i : numbers) {
sum += i;
}
return sum;
}
}
SpringJUnitTestApplicationTests .java
package com.example.demo;
import org.junit.jupiter.api.Test;
import static org.junit.Assert.assertEquals;
import org.springframework.boot.test.context.SpringBootTest;
import com.example.demo.controller.TestController;
@SpringBootTest
class SpringJUnitTestApplicationTests {
TestController myMath = new TestController();
// MyMath.sum
// 1,2,3 => 6
@Test
public void sum_with3numbers() {
System.out.println("Test1");
assertEquals(6,myMath.sum(new int[] { 1, 2, 3 }));
}
@Test
public void sum_with1number() {
System.out.println("Test2");
assertEquals(3, myMath.sum(new int[] { 3 }));
}
}
SpringJUnitTestApplication.java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringJUnitTestApplication {
public static void main(String[] args) {
SpringApplication.run(SpringJUnitTestApplication.class, args);
}
}
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.5.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>SpringJUnitTest</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>SpringJUnitTest</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
How to Run
Right click on project and select Run As -> JUnit Test
Output
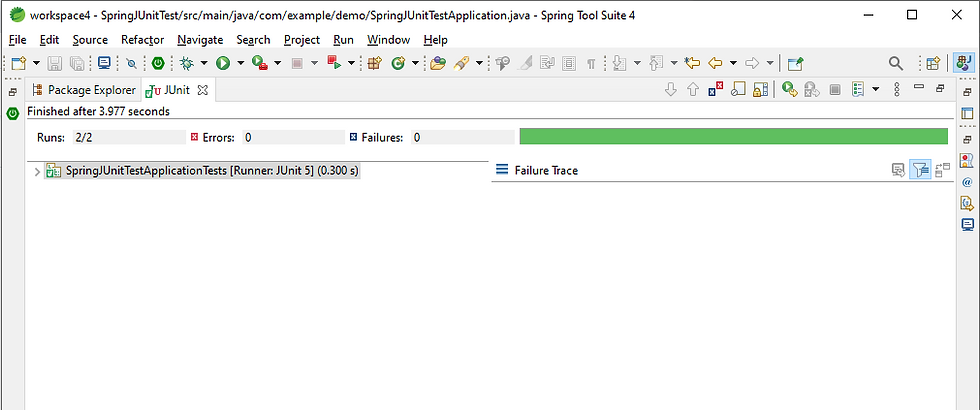
Comments