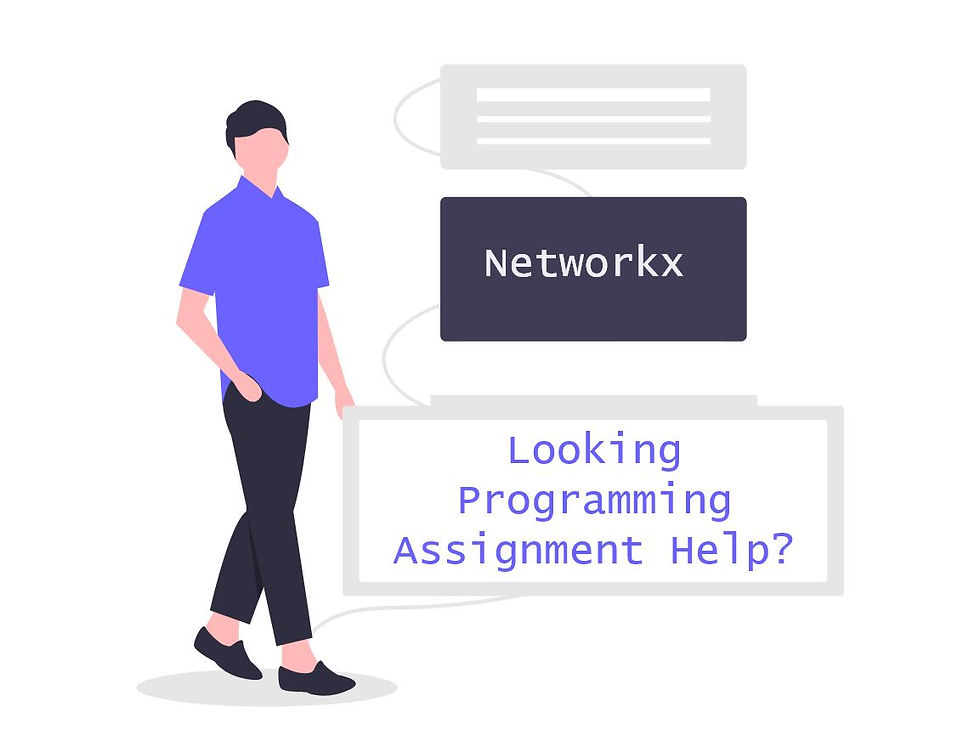
In this blog, we will provide some important R Programs which make your programming skills better.
1. Write a function to accept an array x of integers and return an array y of 0’s and
1’s. If the number in x is divisible by 7, the element in y is a 1; otherwise, it is a 0. You are
NOT allowed to use any loop, exponentiation operation, any function such as length(),
apply(), floor(), ceiling(), etc. You may use other arithmetic operations (addition,
subtraction, multiplication, and division). You CANNOT use the modulo operation, i.e.
%. You are not allowed to use any function that is not mentioned in our lectures. You may use if-else() function. If you cannot find the solution, you may use the for-loop or the
exponentiation operation for a reduced grade of 15 points. (Note: %/% is the integer
division operator.)
2. Write an R program to drop the smallest one exam grade out of four grades. If a
student misses an exam, the grade is NA. You must use NA instead of 0 to represent the
value for exams missing. (a) Complete a function to convert an array to another array in
which all NA grades are replaced with zero. (b) Complete your function to compute the
mean of all elements row by row after dropping one smallest grade. You cannot use any
loop statement inside or outside your functions for both (a) and (b). You may use the
built-in function in R to find the minimum value in an array. You may use ifelse() only
once. You may use the built-in function mean() to find the mean. But be careful! For
testing purpose, you may use the following exam grades for each student in each row:
Exam1 Exam2 Exam3 Exam4
80 NA 90 NA
60 80 100 NA
90 80 100 40
The average grade of each student must be displayed as a row in a matrix. For the example
above, you need to display three rows of values of one column.You may use functions: is.na() or na.omit().
3. (a) Write a function to convert an array of numbers with elements 1, 2, 3, or 4 based on the rule: 1 → 2, 2 → 1, 3 → 4, 4 → 3. If there is any invalid number, not 1, 2, 3, or 4, you may convert it to NA. You are not allowed to use multiple if-statements. You are allowed to use only one R statement in your function to complete the conversion.
(b) Define a matrix contain rows and columns with only 1, 2, 3, or 4 in the matrix. You need to translate all of the matrix elements based on the above rule. You are required to use apply() family function. You cannot use any loop statements. You need to display the input matrix and the output matrix to show that your program works.
For the purpose of testing, you may use:
x ← matrix(c(1, 2, 2, 3, 4, 4, 1, 2, 5), nrow=3, ncol=3)
4. Given a matrix X of integers and an array Y of integers and NA, (a) Write a
function to compare each row of the matrix with the array Y ignoring the element of
NA. Your program will return a matrix of TRUE, FALSE, and NA (if any). (b) Count the
number of rows that return all TRUE and NA (if any). You may use is.na(n) or
na.omit(n).
For both (a) and (b), you cannot use any loop-statement or if statement.
You may use ifelse() though.
For example,
x<- c(1,0,1,0,1,1,1,1,0,1,0,1)
y<- c( 1 , NA, 1, NA)
[1] "*** (a) print the matrix after checking with y ***"
[,1] [,2] [,3] [,4]
[1,] TRUE NA TRUE NA
[2,] TRUE NA TRUE NA
[3,] FALSE NA FALSE NA
[1] "*** (b) print the number of rows after comparing with y ***"
[1] 2
You cannot hardcode the number of rows or columns in your code for the matrix X.
You may assume that the number of elements in each row of the matrix is the same as
the number of elements in the array Y. Your code must work for all of the examples
below:
# displays 2
x<- c(1,0,1,0,1,1,1,1,1, 0 ,1, 1)
y<- c( 1 , 0, 1, NA)
# displays 3
x<- c(1,0,1, 1, 0,1,1,1,0,1,0,0)
y<- c( 1 , 0, NA)
# displays 4
x<- c(1,0,1, 1, 0,1,1,1,0,1,0,0)
1. y<- c( 1 , NA, NA)
Contact us for this machine learning assignment Solutions by Codersarts Specialist who can help you mentor and guide for such machine learning assignments. If you have project or assignment files, You can send at contact@codersarts.com directly.

Comments